In Python, shallow copy and deep copy are ways to create a copy of an object. The difference between the two is related to how references to the original object are handled. Let’s discuss each of them in detail.
Shallow Copy
A shallow copy creates a new object, but the contents of the original object are still referenced in the new object. That means that the new object points to the same memory location as the original object for its data, but the container itself is new. In other words, the copied object is a “shallow” copy of the original. Here is an example:
original_list = [1, 2, [3, 4]] shallow_copy = original_list.copy() print(f"Original List: {original_list}") print(f"Shallow Copy: {shallow_copy}") shallow_copy[2][0] = 99 print(f"Original List: {original_list}") print(f"Shallow Copy: {shallow_copy}")
Output:
Original List: [1, 2, [3, 4]] Shallow Copy: [1, 2, [3, 4]] Original List: [1, 2, [99, 4]] Shallow Copy: [1, 2, [99, 4]]
As you can see from the output, when we changed the value of the nested list in the shallow copy, it also changed the value in the original list. This is because both the original list and the shallow copy refer to the same nested list.
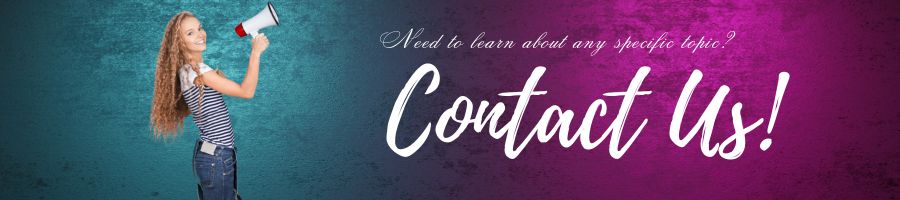
Deep Copy
A deep copy creates a new object and recursively copies all the objects it contains. That means that the new object and all of its contents are entirely new, with new memory addresses. In other words, the copied object is a “deep” copy of the original. Here is an example:
import copy original_list = [1, 2, [3, 4]] deep_copy = copy.deepcopy(original_list) print(f"Original List: {original_list}") print(f"Deep Copy: {deep_copy}") deep_copy[2][0] = 99 print(f"Original List: {original_list}") print(f"Deep Copy: {deep_copy}")
Output:
Original List: [1, 2, [3, 4]] Deep Copy: [1, 2, [3, 4]] Original List: [1, 2, [3, 4]] Deep Copy: [1, 2, [99, 4]]
As you can see from the output, when we changed the value of the nested list in the deep copy, it did not affect the value in the original list. This is because the deep copy created a new list that is entirely separate from the original.
Differences and When to Use
The main difference between shallow copy and deep copy is that a shallow copy only copies the references to the original object’s contents, while a deep copy creates new objects that are entirely separate from the original.
Use a shallow copy when you want to create a new object that shares some of the original object’s contents, but you don’t want to create entirely new objects for every piece of data. This can be useful when dealing with large objects that have many nested objects or data structures.
Use a deep copy when you want to create an entirely new object with no references to the original object’s contents. This is useful when you want to modify the copied object without affecting the original object.