In Python, dictionaries are a powerful data structure used for mapping key-value pairs. They provide a flexible and efficient way to represent and manipulate data. Python dictionaries come with several built-in methods that can be used to perform various operations on them. In this article, we’ll explore the available dictionary methods, their functionality, and the benefits of studying and practicing them.
Creating a Dictionary
Before we dive into the methods, let’s first review how to create a dictionary in Python. A dictionary is created by enclosing a comma-separated list of key-value pairs in curly braces {}
. Each key-value pair is separated by a colon :
. Here’s an example:
my_dict = {'key1': 'value1', 'key2': 'value2', 'key3': 'value3'}
In the example above, we create a dictionary my_dict
with three key-value pairs. The keys are 'key1'
, 'key2'
, and 'key3'
, and the values are 'value1'
, 'value2'
, and 'value3'
, respectively.
Dictionary Methods
Python provides several built-in methods that can be used to manipulate dictionaries. Here’s a list of the available dictionary methods:
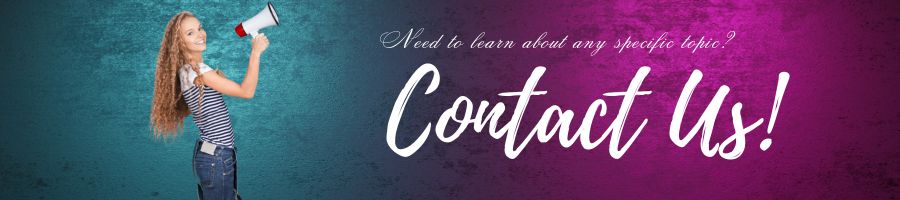
clear()
The clear()
method removes all the key-value pairs from the dictionary, leaving it empty. Here’s an example:
my_dict = {'key1': 'value1', 'key2': 'value2', 'key3': 'value3'} my_dict.clear() print(my_dict) # Output: {}
In the example above, we call the clear()
method on the dictionary my_dict
, which removes all the key-value pairs and leaves the dictionary empty.
copy()
The copy()
method is a built-in method in Python’s dictionary class. It is used to create a shallow copy of the dictionary. The syntax for using the copy()
method is as follows:
new_dict = dict.copy()
Here, dict
is the dictionary that you want to copy, and new_dict
is the new dictionary that will be created as a shallow copy of dict
. Here’s an example of using the copy()
method:
my_dict = {'apple': 5, 'banana': 10, 'orange': 15} new_dict = my_dict.copy() print(new_dict) # Output: {'apple': 5, 'banana': 10, 'orange': 15}
In the example above, we first define a dictionary my_dict
with three key-value pairs. We then use the copy()
method to create a shallow copy of the dictionary, and store it in the variable new_dict
. We then print the value of new_dict
, which shows that it is a shallow copy of my_dict
.
A shallow copy of a dictionary is a new dictionary that contains the same key-value pairs as the original dictionary. However, if the values of the original dictionary are mutable objects, such as lists or other dictionaries, the values in the shallow copy will reference the same objects as the original dictionary. This means that if you modify a mutable value in the shallow copy, the same value in the original dictionary will also be modified.
It is worth noting that the copy()
method creates a new dictionary object, so any modifications made to the copy will not affect the original dictionary. This can be useful when you want to make changes to a dictionary without modifying the original dictionary.
Overall, the copy()
method can be useful when you need to create a new dictionary that contains the same key-value pairs as an existing dictionary, without modifying the original dictionary.
fromkeys()
The fromkeys()
method is a built-in method in Python’s dictionary class. It creates a new dictionary with the specified keys and the same value for each key. The syntax for using the fromkeys()
method is as follows:
dict.fromkeys(keys, value)
Here, keys
is a list of keys to be included in the new dictionary, and value
is the value that is to be assigned to each key. If value
is not specified, then the default value is None
.
Here’s an example of using the fromkeys()
method:
my_dict = dict.fromkeys(['key1', 'key2', 'key3'], 0) print(my_dict) # Output: {'key1': 0, 'key2': 0, 'key3': 0}
In the example above, we create a new dictionary my_dict
with keys 'key1'
, 'key2'
, and 'key3'
, and a value of 0
for each key. The output of the print()
statement shows that the dictionary has been created successfully.
The fromkeys()
method is useful when you want to create a new dictionary with a set of keys and the same default value for each key. It can save time and effort when creating a dictionary with a large number of keys that have the same default value.
get()
The get()
method is a built-in method in Python’s dictionary class. It is used to retrieve the value of a specified key from a dictionary. The syntax for using the get()
method is as follows:
dict.get(key, default=None)
Here, key
is the key whose value is to be retrieved from the dictionary, and default
is the value to be returned if the specified key is not found in the dictionary. If default
is not specified, then the default value is None
. Here’s an example of using the get()
method:
my_dict = {'apple': 5, 'banana': 10, 'orange': 15} apple_count = my_dict.get('apple') print(apple_count) # Output: 5 mango_count = my_dict.get('mango', 0) print(mango_count) # Output: 0
In the example above, we first define a dictionary my_dict
with three key-value pairs. We then use the get()
method to retrieve the value of the key 'apple'
and store it in the variable apple_count
. The output of the first print()
statement shows that the value of the key 'apple'
is 5
.
We then use the get()
method again to retrieve the value of the key 'mango'
, which is not present in the dictionary. We specify a default value of 0
to be returned if the key is not found. The output of the second print()
statement shows that the default value of 0
is returned, as expected.
The get()
method is useful when you want to retrieve the value of a key from a dictionary, but you are not sure if the key exists in the dictionary. It can also be used to avoid raising a KeyError
exception when trying to retrieve the value of a non-existent key.
items()
The items()
method is a built-in method in Python’s dictionary class. It returns a view object that contains the key-value pairs of the dictionary as tuples. The syntax for using the items()
method is as follows:
dict.items()
Here, dict
is the dictionary from which the key-value pairs are to be retrieved. Here’s an example of using the items()
method:
my_dict = {'apple': 5, 'banana': 10, 'orange': 15} items = my_dict.items() print(items) # Output: dict_items([('apple', 5), ('banana', 10), ('orange', 15)])
In the example above, we first define a dictionary my_dict
with three key-value pairs. We then use the items()
method to retrieve a view object containing the key-value pairs of the dictionary. We store the view object in the variable items
and print its value. The output of the print()
statement shows that the view object contains the key-value pairs of the dictionary as tuples.
The items()
method is useful when you want to iterate over the key-value pairs of a dictionary. You can use it in a for
loop to access the keys and values of the dictionary as tuples. Here’s an example:
for key, value in my_dict.items(): print(key, value) #output apple 5 banana 10 orange 15
The items()
method can also be used to create a new dictionary from the key-value pairs of an existing dictionary. Here’s an example:
my_dict = {'apple': 5, 'banana': 10, 'orange': 15} new_dict = dict(my_dict.items()) print(new_dict) # Output: {'apple': 5, 'banana': 10, 'orange': 15}
In the example above, we use the items()
method to retrieve a view object containing the key-value pairs of the dictionary. We then pass this view object as an argument to the dict()
constructor to create a new dictionary new_dict
with the same key-value pairs as my_dict
. The output of the print()
statement shows that the new dictionary has been created successfully.
keys()
The keys()
method is a built-in method in Python’s dictionary class. It returns a view object that contains the keys of the dictionary. The syntax for using the keys()
method is as follows:
dict.keys()
Here, dict
is the dictionary from which the keys are to be retrieved. Here’s an example of using the keys()
method:
my_dict = {'apple': 5, 'banana': 10, 'orange': 15} keys = my_dict.keys() print(keys) # Output: dict_keys(['apple', 'banana', 'orange'])
In the example above, we first define a dictionary my_dict
with three key-value pairs. We then use the keys()
method to retrieve a view object containing the keys of the dictionary. We store the view object in the variable keys
and print its value. The output of the print()
statement shows that the view object contains the keys of the dictionary.
The keys()
method is useful when you want to iterate over the keys of a dictionary. You can use it in a for
loop to access the keys of the dictionary. Here’s an example:
for key in my_dict.keys(): print(key) #output apple banana orange
The keys()
method can also be used to create a new list from the keys of an existing dictionary. Here’s an example:
my_dict = {'apple': 5, 'banana': 10, 'orange': 15} keys_list = list(my_dict.keys()) print(keys_list) # Output: ['apple', 'banana', 'orange']
In the example above, we use the keys()
method to retrieve a view object containing the keys of the dictionary. We then convert this view object to a list using the list()
constructor to create a new list keys_list
with the same keys as my_dict
. The output of the print()
statement shows that the new list has been created successfully.
Values()
The values()
method is a built-in method in Python’s dictionary class. It returns a view object that contains the values of the dictionary. The syntax for using the values()
method is as follows:
dict.values()
Here, dict
is the dictionary from which the values are to be retrieved. Here’s an example of using the values()
method:
my_dict = {'apple': 5, 'banana': 10, 'orange': 15} values = my_dict.values() print(values) # Output: dict_values([5, 10, 15])
In the example above, we first define a dictionary my_dict
with three key-value pairs. We then use the values()
method to retrieve a view object containing the values of the dictionary. We store the view object in the variable values
and print its value. The output of the print()
statement shows that the view object contains the values of the dictionary.
The values()
method is useful when you want to iterate over the values of a dictionary. You can use it in a for
loop to access the values of the dictionary. Here’s an example:
for value in my_dict.values(): print(value) #output 5 10 15
The values()
method can also be used to create a new list from the values of an existing dictionary. Here’s an example:
my_dict = {'apple': 5, 'banana': 10, 'orange': 15} values_list = list(my_dict.values()) print(values_list) # Output: [5, 10, 15]
In the example above, we use the values()
method to retrieve a view object containing the values of the dictionary. We then convert this view object to a list using the list()
constructor to create a new list values_list
with the same values as my_dict
. The output of the print()
statement shows that the new list has been created successfully.
pop()
The pop()
method is a built-in method in Python’s dictionary class. It is used to remove and return an element from the dictionary that has the specified key. The syntax for using the pop()
method is as follows:
dict.pop(key[, default])
Here, dict
is the dictionary from which the element is to be removed, key
is the key of the element to be removed, and default
is the value to be returned if the key is not present in the dictionary (optional). Here’s an example of using the pop()
method:
my_dict = {'apple': 5, 'banana': 10, 'orange': 15} value = my_dict.pop('banana') print(value) # Output: 10 print(my_dict) # Output: {'apple': 5, 'orange': 15}
In the example above, we first define a dictionary my_dict
with three key-value pairs. We then use the pop()
method to remove the element with the key 'banana'
from the dictionary. The value of the removed element is stored in the variable value
. We then print the value of value
, which shows that it is 10
, the value of the 'banana'
key. We also print the dictionary my_dict
after the removal, which shows that the element with the key 'banana'
has been removed.
If the specified key is not present in the dictionary and no default value is provided, the pop()
method raises a KeyError
exception. If a default value is provided, it is returned instead of raising an exception. Here’s an example:
my_dict = {'apple': 5, 'banana': 10, 'orange': 15} value = my_dict.pop('grape', 0) print(value) # Output: 0
In the example above, we try to remove an element with the key 'grape'
from the dictionary my_dict
. Since the key 'grape'
is not present in the dictionary, the default value of 0
is returned and stored in the variable value
. We then print the value of value
, which shows that it is 0
.
The pop()
method is useful when you want to remove an element from a dictionary and also retrieve its value at the same time. It can also be used to handle the case where the specified key is not present in the dictionary, by providing a default value to be returned instead of raising an exception.
popitem()
The popitem()
method is a built-in method in Python’s dictionary class. It is used to remove and return an arbitrary (key, value) pair from the dictionary. The syntax for using the popitem()
method is as follows:
dict.popitem()
Here, dict
is the dictionary from which the (key, value) pair is to be removed. Here’s an example of using the popitem()
method:
my_dict = {'apple': 5, 'banana': 10, 'orange': 15} item = my_dict.popitem() print(item) # Output: ('orange', 15) print(my_dict) # Output: {'apple': 5, 'banana': 10}
In the example above, we first define a dictionary my_dict
with three key-value pairs. We then use the popitem()
method to remove an arbitrary (key, value) pair from the dictionary. The (key, value) pair that is removed is stored in the variable item
. We then print the value of item
, which shows that it is ('orange', 15)
, an arbitrary (key, value) pair from the dictionary. We also print the dictionary my_dict
after the removal, which shows that the (key, value) pair that was removed has been removed from the dictionary.
The popitem()
method can be useful when you want to remove an arbitrary (key, value) pair from a dictionary. However, since the (key, value) pair that is removed is arbitrary, it is not possible to predict which (key, value) pair will be removed from the dictionary. If you need to remove a specific (key, value) pair from the dictionary, it is better to use the pop()
method with the specific key that you want to remove.
It is worth noting that the popitem()
method raises a KeyError
exception if the dictionary is empty. Therefore, you should ensure that the dictionary is not empty before calling the popitem()
method.
Setdefault()
The setdefault() method is used to get the value of a key in a Python dictionary. It takes two parameters: the first one is the key that you want to retrieve the value for, and the second one is the default value that you want to return if the key is not found in the dictionary.
If the key is present in the dictionary, setdefault()
returns the value associated with the key. If the key is not present in the dictionary, setdefault()
adds the key with the default value to the dictionary and returns the default value. Here is an example:
# create a dictionary my_dict = {'a': 1, 'b': 2} # get the value for the key 'a' value = my_dict.setdefault('a', 3) print(value) # output: 1 # get the value for the key 'c' value = my_dict.setdefault('c', 3) print(value) # output: 3 # the dictionary is updated with the key 'c' print(my_dict) # output: {'a': 1, 'b': 2, 'c': 3}
In the example above, we first create a dictionary my_dict
with two key-value pairs: {'a': 1, 'b': 2}
. Then, we use the setdefault()
method to retrieve the value for the key 'a'
. Since 'a'
is present in the dictionary, the method returns the value 1
.
Next, we use setdefault()
to retrieve the value for the key 'c'
. Since 'c'
is not present in the dictionary, the method adds the key 'c'
with the default value 3
and returns the same value.
Finally, we print the updated dictionary, which now includes the key-value pair {'c': 3}
.
The setdefault()
method is useful when you want to get the value of a key in a dictionary and add a default value if the key is not present. It can help simplify your code by avoiding the need to check for the presence of a key before retrieving its value.
Update
The update()
method is used to add or update items in a Python dictionary. It takes either another dictionary object or an iterable of key-value pairs as input, and updates the calling dictionary with the new items. Here is an example:
# create a dictionary my_dict = {'a': 1, 'b': 2} # add a new key-value pair my_dict.update({'c': 3}) print(my_dict) # output: {'a': 1, 'b': 2, 'c': 3} # update an existing key-value pair my_dict.update({'a': 4}) print(my_dict) # output: {'a': 4, 'b': 2, 'c': 3} # add multiple key-value pairs using an iterable my_dict.update([('d', 5), ('e', 6)]) print(my_dict) # output: {'a': 4, 'b': 2, 'c': 3, 'd': 5, 'e': 6}
In the example above, we first create a dictionary my_dict
with two key-value pairs: {'a': 1, 'b': 2}
. Then, we use the update()
method to add a new key-value pair {'c': 3}
to the dictionary.
Next, we use update()
to update the value of the key 'a'
to 4
. Since the key 'a'
already exists in the dictionary, its value is simply updated.
Finally, we use update()
to add multiple key-value pairs to the dictionary, using an iterable of key-value pairs.
The update()
method is useful when you want to add or update items in a dictionary without having to manually loop through each item. It can help simplify your code and make it more readable.
By taking the time to practice and master these methods, you can significantly improve your ability to work with Python dictionaries and build more efficient and effective Python programs. Whether you are working on data science, web development, or any other field that requires programming in Python, having a strong understanding of dictionaries and their methods can help you become a more proficient developer.