Python list comprehensions are a powerful feature that allow you to create a new list by applying an expression to each element of an existing list. List comprehensions provide a concise and efficient way to create and manipulate lists in Python.
In this article, we will discuss the syntax and usage of list comprehensions, including multiple-level deep list comprehensions, their benefits, and their performance characteristics.
List Comprehension Syntax
The basic syntax of list comprehension is as follows:
new_list = [expression for element in old_list if condition]
The expression is the operation that is applied to each element of the old list to create a new value. The element is a variable that represents each element of the old list in turn. The condition is an optional filter that only includes elements that meet a certain criteria. For example, consider the following code:
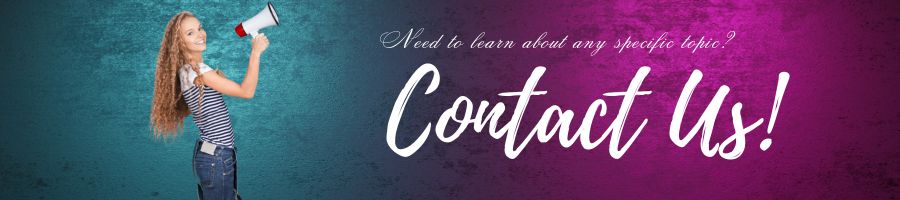
numbers = [1, 2, 3, 4, 5] squares = [x ** 2 for x in numbers if x % 2 == 0] print(squares) #output: [4, 16]
This code creates a new list called squares
that contains the squares of only the even numbers in the numbers
list.
Multiple Level Deep List Comprehensions
List comprehensions can also be nested to create multiple level deep list comprehensions. This allows you to create complex lists with more complex expressions and conditions.
For example, consider the following code:
matrix = [[1, 2, 3], [4, 5, 6], [7, 8, 9]] flattened = [num for row in matrix for num in row if num % 2 == 0] print(flattened) #output: [2, 4, 6, 8]
This code creates a new list called flattened
that contains only the even numbers from the matrix
list, flattened into a single list.
In this example, the nested list comprehension is achieved by having two for
statements in the list comprehension, with the inner for
statement iterating over each element in the rows of the matrix
list.
Nested List Comprehensions
Nested list comprehensions are a powerful feature of Python that allow you to create complex lists with multiple levels of nesting. They are created by placing one or more list comprehensions inside another list comprehension.
The syntax for nested list comprehensions is similar to regular list comprehensions, with the addition of one or more nested for
loops. Here is an example of a simple nested list comprehension that creates a matrix of values:
matrix = [[x*y for x in range(5)] for y in range(5)] print(matrix) #output: [[0, 0, 0, 0, 0], [0, 1, 2, 3, 4], [0, 2, 4, 6, 8], [0, 3, 6, 9, 12], [0, 4, 8, 12, 16]]
In this example, the outer list comprehension creates a new list by iterating over a range of values for y
, and for each value of y
, the inner list comprehension creates a list of values by iterating over a range of values for x
.
This creates a 5×5 matrix of values, where each element is the product of its row and column index.
Nested list comprehensions can also be used to filter and transform data in more complex ways. Here is an example that creates a new list of even numbers from a nested list:
numbers = [[1, 2, 3], [4, 5, 6], [7, 8, 9]] even_numbers = [x for sublist in numbers for x in sublist if x % 2 == 0] print(even_numbers) #output: [2, 4, 6, 8]
In this example, the nested list comprehension iterates over each sublist in the numbers
list, and for each sublist, it iterates over each element x
. If x
is even, it is included in the even_numbers
list.
This creates a new list of even numbers by flattening the nested numbers
list into a single list and filtering for even numbers.
Nested list comprehensions can also be used to create more complex data structures, such as nested dictionaries or sets. Here is an example that creates a new dictionary of sets from a nested list:
data = [[1, 2, 3], [2, 3, 4], [3, 4, 5]] dictionary_of_sets = {i: {j for row in data for j in row if i == j} for i in set(x for row in data for x in row)} print(dictionary_of_sets) #output: {1: {1}, 2: {2}, 3: {3}, 4: {2, 4}, 5: {5}}
In this example, the nested list comprehension creates a new dictionary of sets by iterating over each unique element in the data
list and creating a set of all elements that are equal to it in each row of the data
list. This creates a new dictionary where each key is a unique element from the data
list, and each value is a set of all elements that are equal to it in the data
list.
Nested list comprehensions provide a flexible and powerful way to create and manipulate complex data structures in Python. With practice and experimentation, you can use nested list comprehensions to create more complex and efficient code for a wide range of data manipulation tasks.
List Comprehension Benefits
List comprehensions offer several benefits over traditional methods of list manipulation in Python. Some of these benefits include:
- Concise syntax: List comprehensions allow you to express complex operations in a single line of code, making your code more readable and easier to maintain.
- Performance: List comprehensions can be faster than traditional
for
loops, especially for large lists. This is because list comprehensions are optimized for speed by the Python interpreter. - Flexibility: List comprehensions can be used to create a wide range of new lists, from simple lists to complex nested lists. This allows you to handle a variety of data manipulation tasks with ease.
List Comprehension Performance
List comprehensions are generally faster than traditional for
loops, especially for large lists. This is because list comprehensions are optimized for speed by the Python interpreter.
However, there are some cases where list comprehensions may not be the best choice. For example, if you are working with very large lists or if you need to perform a complex operation on each element of the list, a traditional for
loop may be faster and more efficient.
It’s also worth noting that list comprehensions can be memory-intensive, especially for large lists or for deeply nested list comprehensions. In these cases, it may be necessary to use other techniques, such as generators or iterators, to reduce memory usage.
Conclusion
Python list comprehensions are a powerful feature that provide a concise and efficient way to create and manipulate lists. They offer several benefits over traditional methods of list manipulation, including concise syntax, improved performance, and flexibility. By understanding the syntax and usage of list comprehensions, you can leverage their power to create complex lists with ease. However, it’s important to be aware of their performance characteristics, and to use other techniques, such as generators or iterators, when necessary to optimize memory usage. Overall, list comprehensions are a valuable tool in any Python programmer’s toolbox.