In Python, a namespace is a mapping from names to objects. Every name that is defined in a Python program has a namespace associated with it. Namespaces are used to avoid naming conflicts, and they allow you to organize your code and separate it into logical units.
There are several types of namespaces in Python:
- Built-in namespace: This namespace contains all the built-in functions, constants, and types that are available in Python. You can use these objects in your code without having to import anything.
- Global namespace: This namespace contains all the names that are defined at the top level of a module. These names can be accessed from anywhere within the module.
- Local namespace: This namespace is created whenever a function is called, and it contains all the names that are defined within that function.
- Enclosing namespace: This namespace is used when you have nested functions. If a name is not found in the local namespace, Python will look for it in the enclosing namespace.
Python namespaces are implemented as dictionaries. When you create a new variable or function in Python, it is added to the current namespace as a key-value pair. The key is the name of the variable or function, and the value is a reference to the object.
You can access the current namespace using the locals()
function or the global namespace using the globals()
function. These functions return a dictionary that contains all the names defined in the corresponding namespace.
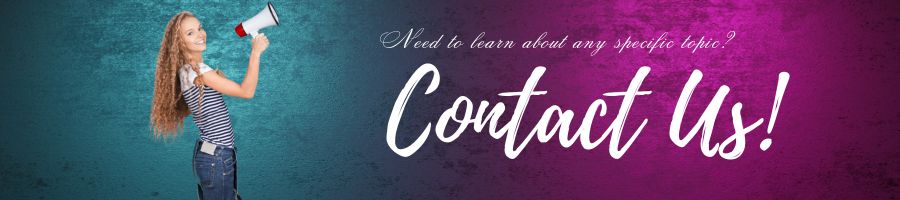
When you import a module in Python, it creates a new namespace for that module. You can access the names defined in a module using dot notation. For example, if you have a module called mymodule
that defines a function called myfunction
, you can access it using the syntax mymodule.myfunction()
. You can also use the dir()
function to get a list of names defined in a namespace, and the getattr()
and setattr()
functions to get and set attributes of objects in a namespace.
The lifetime of a Namespace: The lifetime of a namespace depends on the scope of the object. Once the scope of the object ends, the lifetime of the namespace ends. That s why it is not possible to access inner namespace objects from outer namespaces.
Scope of Objects in Python:
The scope of a name refers to the region of the code where that name is visible or accessible. In Python, there are four types of scopes:
- Local Scope: The scope of a name defined inside a function is local to that function. This means that the name is not visible outside the function.
- Enclosing Scope: If a function is defined inside another function, the enclosing function’s scope is also visible inside the inner function.
- Global Scope: The global scope is the outermost scope in a Python program. Names defined at the global level are visible throughout the entire program.
- Built-in Scope: The built-in scope contains all the names that are built into Python. Names in the built-in scope are always visible and can be used without importing any modules.
To access a name in a different namespace or scope, you can use the dot notation to specify the namespace or scope of the name. For example, to access a name defined in the global namespace, you can use the syntax global_name = module_name.global_name
. Similarly, to access a name defined in an enclosing scope, you can use the syntax enclosing_name = enclosing_function.enclosing_name
.
Namespace is one of the most important concepts in Python and if not understood properly, it might create problems. So, it’s always advised to invest some time to understand it properly.
The codes (if any) mentioned in this post can be downloaded from github.com. Share the post with someone you think can benefit from the information