Memory management is an important aspect of any programming language, and Python is no exception. In Python, memory management is handled automatically by the interpreter. This means that you don’t have to worry about allocating and deallocating memory like you do in low-level languages such as C or C++.
However, this doesn’t mean that you can ignore memory management entirely. Python’s automatic memory management system is designed to be efficient, but it’s not perfect. In this tutorial, we’ll explore some of the ways that you can optimize memory usage in your Python programs.
Understanding Python’s Memory Model
Before we dive into memory optimization techniques, it’s important to have a basic understanding of how Python’s memory model works.
In Python, all objects are stored in memory as a combination of a header and a body. The header contains information about the object’s type and size, while the body contains the actual data.
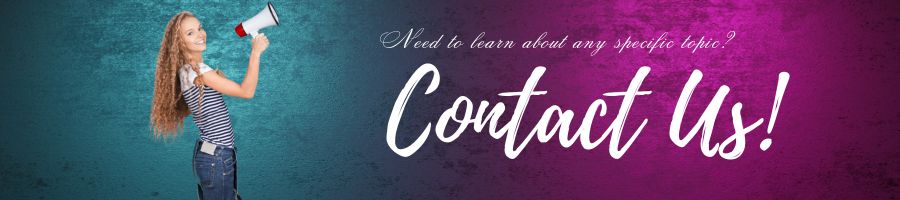
When you create an object in Python, the interpreter allocates memory to store the object’s header and body. When you’re done with the object, the interpreter frees up the memory so that it can be used for other purposes.
One important thing to note is that Python uses reference counting to keep track of how many references there are to an object. When an object’s reference count drops to zero, the interpreter frees up the memory used by the object.
Optimizing Memory Usage
Now that we have a basic understanding of how Python’s memory model works, let’s look at some ways that you can optimize memory usage in your Python programs.
1. Use Generators
Generators are a powerful feature in Python that allows you to generate a sequence of values on-the-fly, rather than generating all the values at once and storing them in memory.
For example, consider the following code:
def create_list(): result = [] for i in range(1000000): result.append(i) return result
This code creates a list of one million integers. The list is stored in memory and can be quite large, depending on the size of each integer.
Now consider the following code, which uses a generator instead:
def create_generator(): for i in range(1000000): yield i
This code generates the same sequence of integers, but it does so on-the-fly, without storing the entire sequence in memory at once. This can be much more memory-efficient for large sequences.
2. Use Context Managers
In Python, you can use context managers to automatically clean up resources when you’re done with them. This can be especially useful when working with large objects that consume a lot of memory.
For example, consider the following code:
file = open('large_file.txt', 'r') data = file.read() file.close()
This code reads the entire contents of a large file into memory. When you’re done with the file, you need to remember to close it to free up the memory.
Now consider the following code, which uses a context manager instead:
with open('large_file.txt', 'r') as file: data = file.read()
This code uses a context manager to automatically close the file when you’re done with it, freeing up the memory used by the file’s contents.
3. Use Immutable Data Structures
In Python, immutable data structures such as tuples and frozensets are stored more efficiently than mutable data structures such as lists and sets. This is because Python can optimize the memory usage of immutable objects more effectively.
For example, consider the following code:
my_list = [1, 2, 3] my_tuple = (1, 2, 3)
The codes (if any) mentioned in this post can be downloaded from github.com. Share the post with someone you think can benefit from the information