If you’ve ever worked with large datasets or needed to process an infinite data stream, you’ve probably faced memory issues. Python’s yield keyword is a game-changer—it lets you generate values on the fly instead of holding everything in memory. In this guide, we’ll break down yield in a way that makes sense, even if you’re new to Python.
What is yield?
Think of yield
as a smarter return
. Instead of sending back a single value and exiting the function, yield pauses the function and saves its state. The next time you call it, execution resumes right where it left off. That being said, The yield keyword is used to create generators, which are special types of iterators that allow values to be produced lazily, one at a time, instead of returning them all at once. This makes yield particularly useful for handling large datasets efficiently, as it allows iteration without storing the entire sequence in memory
def my_generator(): yield 1 yield 2 yield 3 # Using the generator for value in my_generator(): print(value) #output 1 2 3
Unlike returning a list of [1, 2, 3]
, this approach keeps memory usage minimal because values are generated one by one as needed.
How yield Works
A function that contains yield becomes a generator function. Calling it doesn’t execute the function right away; instead, it returns a generator object that you can iterate over.
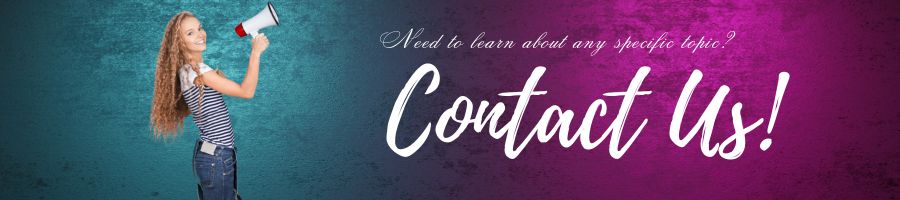
def count_up_to(n): count = 1 while count <= n: yield count count += 1 counter = count_up_to(5) print(next(counter)) # 1 print(next(counter)) # 2 print(next(counter)) # 3
Each next(counter)
call resumes execution at the last yield, producing the next value.
yield vs. return
Feature | return | yield |
---|---|---|
Returns value | Once | Multiple times |
Function type | Normal function | Generator function |
Memory usage | Stores all values | Generates values on demand |
Execution | Ends immediately | Pauses and resumes |
Using return (Consumes More Memory)
def squares_list(n): return [i ** 2 for i in range(n)] print(squares_list(5)) # [0, 1, 4, 9, 16]
Using yield
(More Efficient)
def squares_generator(n): for i in range(n): yield i ** 2 print(list(squares_generator(5))) # [0, 1, 4, 9, 16]
Why Use yield?
- ✅ Boosts Performance: Avoids unnecessary calculations.
- ✅ Saves Memory: Generates values only when needed.
- ✅ Retains State: Remembers variable values between calls.
- ✅ Easier Iterators: No need to write
__iter__()
and__next__()
manually.
Downsides of yield
- ❌ Debugging Can Be Tricky: Since execution jumps around, it’s harder to trace errors.
- ❌ One-Time Use: Generators can’t be rewound like lists—you need to recreate them if you want to iterate again.
- ❌ No Indexing: Unlike lists, you can’t access elements with my_gen[2].
When to Use yield
Processing Large Files
def read_large_file(file_path): with open(file_path, 'r') as file: for line in file: yield line.strip() for line in read_large_file("large_file.txt"): print(line)
This method reads a file line by line, preventing memory overload.
Generating Infinite Sequences
def fibonacci(): a, b = 0, 1 while True: yield a a, b = b, a + b fib = fibonacci() print(next(fib)) # 0 print(next(fib)) # 1 print(next(fib)) # 1
Great for things like real-time data streams or live dashboards.
Streaming API Data
def api_data_stream(): import time for i in range(1, 4): yield {"data": f"Chunk {i}"} time.sleep(1) for chunk in api_data_stream(): print(chunk)
Useful for fetching and processing API responses without blocking execution.
The yield keyword is an absolute powerhouse when it comes to handling large datasets, optimizing performance, and writing clean, efficient Python code. While it takes some getting used to, mastering yield
will make you a much more effective Python developer. Give it a try in your next project and see the difference it makes!