When working with Python, you may have encountered the with
statement. This innocuous little keyword unlocks the magic of context managers. But what exactly are context managers, and how can they simplify your code? In this article, we’ll explore context managers from the basics to advanced concepts, with plenty of examples along the way.
What is a Context Manager?
A context manager is a Python construct that allows you to manage resources efficiently and safely. Resources, such as files, network connections, or database sessions, often need proper setup and cleanup. Context managers automate this process, ensuring that resources are released even if an error occurs.
The most common way to use a context manager is with the with
statement:
with open("example.txt", "r") as file: content = file.read() print(content) # The file is automatically closed here.
In this example, the open()
function returns a file object that acts as a context manager. When the with
block is exited, the file is automatically closed—no need for a manual file.close()
.
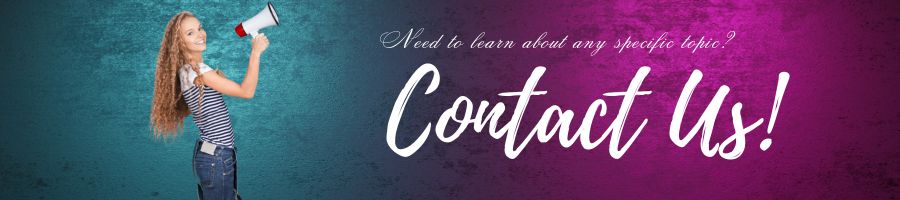
How Context Managers Work
Behind the scenes, a context manager uses two special methods:
__enter__(self)
: Sets up the resource and optionally returns it.__exit__(self, exc_type, exc_value, traceback)
: Cleans up the resource. If an exception occurs, it receives details about the exception and can choose to suppress it.
Let’s create a simple custom context manager to understand these methods:
class SimpleContextManager: def __enter__(self): print("Entering the context") return "Resource" def __exit__(self, exc_type, exc_value, traceback): print("Exiting the context") if exc_type: print(f"An exception occurred: {exc_value}") return False # Do not suppress exceptions with SimpleContextManager() as resource: print(f"Using the {resource}") # Uncomment the line below to test exception handling # raise ValueError("Oops!")
Output:
Entering the context Using the Resource Exiting the context
If an exception occurs, __exit__
handles it, but in this case, we’ve chosen not to suppress it.
Using contextlib
for Simpler Context Managers
Python’s contextlib
module provides tools to create context managers more easily. For example, the @contextmanager
decorator allows you to use a generator to define a context manager:
from contextlib import contextmanager @contextmanager def managed_resource(): print("Setting up resource") yield "Resource" print("Cleaning up resource") with managed_resource() as resource: print(f"Using {resource}")
Output:
Setting up resource Using Resource Cleaning up resource
This approach reduces boilerplate code and makes your context managers more concise.
Real-World Examples of Context Managers
1. File Handling
The built-in open()
function is the quintessential example of a context manager:
with open("data.txt", "w") as file: file.write("Hello, world!") # File is closed automatically
2. Lock Management
Context managers are often used for thread synchronization:
from threading import Lock lock = Lock() with lock: print("Critical section")
3. Temporary Files
The tempfile
module provides context managers for creating temporary files and directories:
import tempfile with tempfile.TemporaryFile() as temp_file: temp_file.write(b"Temporary data") temp_file.seek(0) print(temp_file.read())
4. Database Connections
Database libraries often use context managers to manage connections and transactions:
import sqlite3 with sqlite3.connect("example.db") as conn: cursor = conn.cursor() cursor.execute("CREATE TABLE IF NOT EXISTS users (id INTEGER PRIMARY KEY, name TEXT)") cursor.execute("INSERT INTO users (name) VALUES (?)", ("Alice",)) conn.commit()
Advanced Concepts
Nesting Context Managers
You can nest multiple context managers using nested with
statements or the contextlib.ExitStack
:
with open("input.txt") as infile, open("output.txt", "w") as outfile: data = infile.read() outfile.write(data)
Or using ExitStack
for dynamic context management:
from contextlib import ExitStack with ExitStack() as stack: files = [stack.enter_context(open(f"file_{i}.txt", "w")) for i in range(3)] for i, file in enumerate(files): file.write(f"File {i}\n")
Suppressing Exceptions
The contextlib.suppress
context manager ignores specified exceptions:
from contextlib import suppress with suppress(FileNotFoundError): open("nonexistent.txt") print("No exception raised")
Async Context Managers
Python’s asyncio
library supports asynchronous context managers:
import asyncio class AsyncContextManager: async def __aenter__(self): print("Async setup") return "Async resource" async def __aexit__(self, exc_type, exc_value, traceback): print("Async cleanup") async def main(): async with AsyncContextManager() as resource: print(f"Using {resource}") asyncio.run(main())
Output:
Async setup Using Async resource Async cleanup
Conclusion
Context managers are a powerful Python feature that simplifies resource management, improves code readability, and helps prevent bugs. From managing files and locks to handling temporary resources and database connections, their applications are vast.
By mastering context managers, you’ll write cleaner, more Pythonic code. Start small, experiment with custom implementations, and gradually explore advanced features like contextlib
and asynchronous context managers. With practice, you’ll soon see how indispensable they are in your Python toolkit.