Python keywords are reserved words that have special meanings and are used to define the syntax and structure of the Python language. These keywords cannot be used as variable names or other identifiers because they are reserved for specific purposes in the language. The number of keywords might change in different python versions. There are 35 keywords in Python 3.10.4
List of Python Keywords:
'False', 'None', 'True', 'and', 'as', 'assert', 'async', 'await', 'break', 'class', 'continue', 'def', 'del', 'elif', 'else', 'except', 'finally', 'for', 'from', 'global', 'if', 'import', 'in', 'is', 'lambda', 'nonlocal', 'not', 'or', 'pass', 'raise', 'return', 'try', 'while', 'with', 'yield'
Since the keywords for a specific version might change, you can always get a list of keywords using below code:
import keyword keywords_list = keyword.kwlist print('Total No. Of Keywords: ', len(keywords_list)) print('All Keywords: ',keywords_list) ##output will be Total No. Of Keywords: 35 All Keywords: ['False', 'None', 'True', 'and', 'as', 'assert', 'async', 'await', 'break', 'class', 'continue', 'def', 'del', 'elif', 'else', 'except', 'finally', 'for', 'from', 'global', 'if', 'import', 'in', 'is', 'lambda', 'nonlocal', 'not', 'or', 'pass', 'raise', 'return', 'try', 'while', 'with', 'yield']
Soft Keywords:
Some identifiers are only reserved under specific contexts. These are known as soft keywords. The identifiers match
, case
and _
can syntactically act as keywords in contexts related to the pattern-matching statement, but this distinction is done at the parser level, not when tokenizing. As soft keywords, their use with pattern matching is possible while still preserving compatibility with existing code that uses match
, case
and _
as identifier names
import keyword keywords_list = keyword.softkwlist print('Total No. Of Soft Keywords: ', len(keywords_list)) print('All Soft Keywords: ',keywords_list) ##output will be #Total No. Of Soft Keywords: 3 #All Soft Keywords: ['_', 'case', 'match']
How to check if a string is a valid keyword in Python?
#how to check if a string is a reserved keyword or not import keyword s = 'while' if keyword.iskeyword(s): print(s,' is a reserved keyword') else: print(s,' is a not a reserved keyword') # output will be # while is a reserved keyword
How to check if a string is a valid soft keyword in Python?
#how to check if a string is a soft keyword or not import keyword s = 'match' if keyword.issoftkeyword(s): print(s,' is a soft keyword') else: print(s,' is a not a soft keyword') ##output will be #match is a soft keyword
Keywords In Detail
Here’s an explanation of each Python keyword:
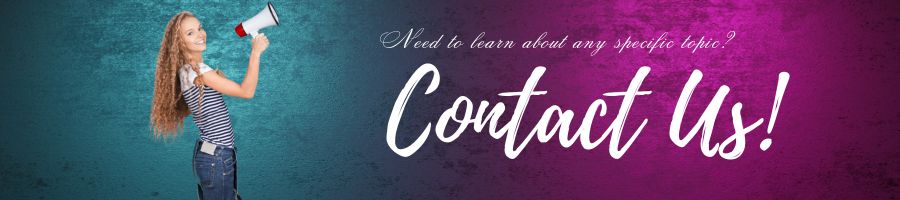
False
: This keyword represents the boolean valueFalse
.class
: This keyword is used to define a new class in Python.finally
: This keyword is used to define a block of code that is executed after atry
block, regardless of whether an exception was raised or not.is
: This keyword is used to test if two variables refer to the same object in memory.return
: This keyword is used to return a value from a function.None
: This keyword represents the null value in Python.continue
: This keyword is used to skip the current iteration of a loop.for
: This keyword is used to define a loop that iterates over a sequence of elements.lambda
: This keyword is used to create a small anonymous function.try
: This keyword is used to define a block of code that may raise an exception.True
: This keyword represents the boolean valueTrue
.def
: This keyword is used to define a new function.from
: This keyword is used to import specific attributes or functions from a module.nonlocal
: This keyword is used to reference a variable in the closest enclosing scope outside of the current function.while
: This keyword is used to define a loop that executes while a certain condition is true.and
: This keyword is used to perform a logicaland
operation.del
: This keyword is used to delete an object.global
: This keyword is used to indicate that a variable is a global variable.not
: This keyword is used to perform a logicalnot
operation.with
: This keyword is used to define a block of code that uses a context manager.as
: This keyword is used to give a different name to an imported module or attribute.elif
: This keyword is used to define an alternate condition in anif
statement.if
: This keyword is used to define a conditional statement.or
: This keyword is used to perform a logicalor
operation.yield
: This keyword is used in a function to yield a value to the caller.assert
: This keyword is used to assert that a certain condition is true.else
: This keyword is used to define the alternate branch of anif
statement.import
: This keyword is used to import a module.pass
: This keyword is used as a placeholder where code will eventually go.break
: This keyword is used to break out of a loop.except
: This keyword is used to catch an exception.in
: This keyword is used to test if a sequence contains a certain value.raise
: This keyword is used to raise an exception.async
: This keyword is used to define a coroutine function.await
: This keyword is used to wait for a coroutine function to complete.
The codes mentioned in this post can be downloaded from github.com. Share the post with someone you think can benefit from the information.