When you start your journey with Python, one thing becomes clear quite early: Python is powerful because of its extensive ecosystem of libraries and packages. These packages do everything from data analysis to machine learning, web development, automation, and beyond. However, managing these packages efficiently requires the use of package managers—tools designed to make your life easier as a Python developer.
In this article, we will explore Python package managers from the ground up, covering both beginner-friendly and advanced concepts. By the end, you’ll understand the major players in the Python package management world and how to use them effectively.
What Is a Package Manager?
A package manager is a tool that automates the process of installing, updating, and managing software packages. For Python developers, this means you can:
- Easily install third-party libraries.
- Keep your projects organized with specific versions of dependencies.
- Avoid dependency conflicts between projects.
Think of it like this: a package manager is your assistant who takes care of fetching the right tools (libraries) from the vast Python ecosystem and ensures they’re ready for use in your project.
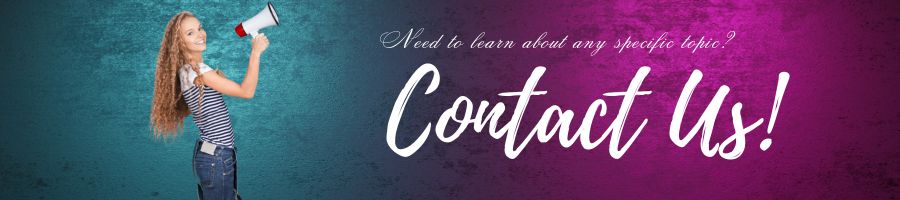
The Major Python Package Managers
Let’s dive into the most popular package managers in the Python world, their use cases, and when to choose each.
1. pip (Python’s Default Package Manager)
What is pip? Pip stands for “Pip Installs Packages,” and it’s the default package manager for Python. It comes bundled with Python (since version 3.4), making it the go-to tool for most developers starting out.
Key Features:
- Install packages from the Python Package Index (PyPI).
- Upgrade or uninstall packages easily.
- Lightweight and straightforward.
Basic Commands:
# Install a package pip install package_name # Install a specific version pip install package_name==1.2.3 # Upgrade a package pip install --upgrade package_name # Uninstall a package pip uninstall package_name
Pro Tips for pip:
- Use a
requirements.txt
file to manage dependencies for your project:pip freeze > requirements.txt pip install -r requirements.txt
- Combine pip with virtual environments (more on that later) for better project isolation.
2. pipenv
What is pipenv? Pipenv is an advanced tool that combines pip
and virtualenv
(a tool for creating isolated environments). It simplifies dependency management by providing a single tool to handle everything.
Key Features:
- Automatically creates and manages virtual environments.
- Uses
Pipfile
andPipfile.lock
for dependency tracking. - Ensures deterministic builds by locking package versions.
Basic Commands:
# Install a package pipenv install package_name # Install a development dependency pipenv install package_name --dev # Activate the virtual environment pipenv shell # Run a script within the virtual environment pipenv run python script.py
Why Use pipenv? If you’re managing medium-to-large projects and want both ease of use and robust dependency tracking, pipenv is an excellent choice.
3. conda
What is conda? Conda is more than just a Python package manager. It’s a general-purpose package manager that works for multiple programming languages (Python, R, etc.) and handles non-Python dependencies, such as C libraries.
Key Features:
- Manages Python environments and packages.
- Works seamlessly for data science and machine learning projects.
- Provides access to both PyPI and Conda-specific packages.
Basic Commands:
# Install a package conda install package_name # Create a new environment conda create --name env_name python=3.9 # Activate an environment conda activate env_name # Deactivate an environment conda deactivate
Why Use conda? Conda shines in scientific computing. If your project involves complex dependencies, such as TensorFlow or OpenCV, conda can handle these with ease.
4. poetry
What is poetry? Poetry is a modern package manager focused on simplicity and reliability. It combines dependency management and packaging into a single tool.
Key Features:
- Manages dependencies via
pyproject.toml
. - Handles publishing your package to PyPI.
- Ensures reproducible builds with lock files.
Basic Commands:
# Initialize a new project poetry init # Add a dependency poetry add package_name # Install all dependencies poetry install # Run your project’s code poetry run python script.py
Why Use poetry? If you’re working on libraries or open-source projects and want a clean, modern workflow, poetry is an excellent choice.
Virtual Environments: A Crucial Concept
Virtual environments allow you to isolate your project’s dependencies, ensuring that packages installed for one project don’t interfere with others.
Tools for Virtual Environments:
venv
: Built into Python (since version 3.3). Use it like this:python -m venv env_name source env_name/bin/activate # On Linux/Mac env_name\Scripts\activate # On Windows
virtualenv
: An older but feature-rich tool for creating virtual environments.- As mentioned earlier,
pipenv
andconda
also manage virtual environments seamlessly.
Advanced Concepts
1. Dependency Resolution
When multiple packages have overlapping dependencies, the package manager determines the best version to install. Tools like pipenv
and poetry
excel here by locking versions to avoid conflicts.
2. Publishing Your Own Package
Ready to share your code with the world? Use poetry
or twine
to publish your package to PyPI.
Basic Steps:
- Create a
setup.py
or usepoetry
to define your package. - Build your package:
python setup.py sdist
- Upload to PyPI:
twine upload dist/*
3. Choosing the Right Tool
Here’s a quick decision matrix:
- Use
pip
for small projects or quick experiments. - Use
pipenv
for straightforward dependency and environment management. - Use
conda
for data science or projects with non-Python dependencies. - Use
poetry
for library development or modern workflows.
Final Thoughts
Package managers are the backbone of efficient Python development. By understanding the strengths and use cases of tools like pip
, pipenv
, conda
, and poetry
, you can choose the right tool for the job and focus on what truly matters: building great software.
So go ahead, explore these tools, and take your Python projects to the next level. Happy coding!