ANSI escape codes are sequences of characters used to control text formatting, color, and other display attributes on terminals and consoles. These codes are commonly used in command-line interfaces and are supported by most modern terminal emulators.
In Python, you can use ANSI escape codes to change the color of text printed to the console. To do this, you can add special characters to your strings that are interpreted by the terminal emulator as instructions to change the color or other formatting of the text that follows.
For example, to print text in blue, you can use the escape code “\033[34m“, which sets the foreground color to blue. To print text in red, you can use the escape code “\033[31m“, which sets the foreground color to red.
To reset the color to the default, you can use the escape code “\033[0m“.
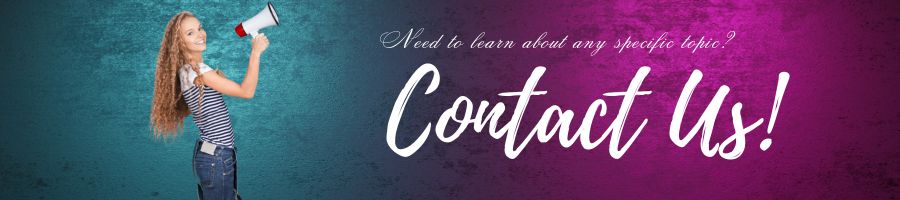
Note that not all terminals and consoles support ANSI escape codes, and some may support a different set of escape codes or none at all. It’s a good practice to test your code on different terminal emulators to ensure it works as expected.
List of Ansi Codes
Here is the same list of ANSI escape codes presented in a table format:
Code | Effect |
---|---|
\033[0m | Resets all formatting and colors to the default |
\033[1m | Bold text |
\033[2m | Faint text |
\033[3m | Italic text |
\033[4m | Underlined text |
\033[30m | Black text |
\033[31m | Red text |
\033[32m | Green text |
\033[33m | Yellow text |
\033[34m | Blue text |
\033[35m | Purple text |
\033[36m | Cyan text |
\033[37m | White text |
\033[40m | Black background |
\033[41m | Red background |
\033[42m | Green background |
\033[43m | Yellow background |
\033[44m | Blue background |
\033[45m | Purple background |
\033[46m | Cyan background |
\033[47m | White background |
Note that the code \033
is the escape character for ANSI escape codes, but can also be written as \x1b
. Additionally, some terminal emulators may use slightly different codes or support additional codes beyond this list.
ANSI codes for Cursor
Here are some additional ANSI escape codes that can be used for cursor positioning and window manipulation:
Code | Function |
---|---|
\033[nA | Moves the cursor up n lines |
\033[nB | Moves the cursor down n lines |
\033[nC | Moves the cursor forward n columns |
\033[nD | Moves the cursor backward n columns |
\033[y;xH | Moves the cursor to the (y,x) position |
\033[2J | Clears the entire screen |
\033[K | Clears the line from the cursor position to the end of the line |
\033[s | Saves the cursor position |
\033[u | Restores the cursor position |
Note that these codes are just a small sample of what is possible with ANSI escape codes, and there are many more codes that can be used for more complex terminal applications.
Python Using ANSI Escape Codes
Basic Examples
Here are 10 examples of using ANSI escape codes in Python’s print
function for creative text formatting:
- Print text in bold:
print("\033[1mThis text is bold\033[0m")
- Print text in italic:
print("\033[3mThis text is italic\033[0m")
- Print text in underline:
print("\033[4mThis text is underlined\033[0m")
- Print text in red:
print("\033[31mThis text is in red\033[0m")
- Print text in green:
print("\033[32mThis text is in green\033[0m")
- Print text in yellow:
print("\033[33mThis text is in yellow\033[0m")
- Print text in blue:
print("\033[34mThis text is in blue\033[0m")
- Print text in purple:
print("\033[35mThis text is in purple\033[0m")
- Print text in cyan:
print("\033[36mThis text is in cyan\033[0m")
- Print text with a rainbow effect:
import time colors = [31, 33, 32, 36, 34, 35] # Red, yellow, green, cyan, blue, purple for i in range(20): for color in colors: print(f"\033[{color}m*\033[0m", end="") time.sleep(0.1) print()
Note that these codes may not work on all terminal emulators and the color may differ based on the terminal.
Advanced examples:
- Printing a progress bar with colored bars:
import time def print_progress_bar(progress): bar_length = 40 filled_length = int(bar_length * progress) bar = '\u001b[42m' + 'â–ˆ' * filled_length + '\u001b[0m' + '-' * (bar_length - filled_length) print('\rProgress: |{}| {:.0f}%'.format(bar, progress * 100), end='', flush=True) time.sleep(0.1) for i in range(101): print_progress_bar(i/100)
- Printing a colored warning message:
def print_warning(msg): print('\u001b[43m\u001b[31mWARNING:\u001b[0m', msg) print_warning('This is a warning message')
- Printing a colored success message:
def print_success(msg): print('\u001b[42m\u001b[30mSUCCESS:\u001b[0m', msg) print_success('This is a success message')
- Printing a colored error message:
def print_error(msg): print('\u001b[41m\u001b[37mERROR:\u001b[0m', msg) print_error('This is an error message')
- Printing a table with colored headers:
def print_table(headers, rows): print('\u001b[4m', end='') for i, header in enumerate(headers): print(header.ljust(10) if i < len(headers)-1 else header) print('\u001b[0m', end='') for row in rows: print() for i, col in enumerate(row): color = '\u001b[31m' if i == 0 else '\u001b[0m' print(color + str(col).ljust(10) if i < len(row)-1 else color + str(col) + '\u001b[0m', end='') headers = ['Name', 'Age', 'Gender'] rows = [['Alice', 25, 'F'], ['Bob', 35, 'M'], ['Charlie', 45, 'M']] print_table(headers, rows)
- Printing a colored calendar:
import calendar def print_calendar(year, month): print('\u001b[1m\u001b[4m', calendar.month_name[month], year, '\u001b[0m') print('\u001b[4m', 'Mo Tu We Th Fr Sa Su', '\u001b[0m') cal = calendar.monthcalendar(year, month) for week in cal: for day in week: if day == 0: print(' ', end='') else: color = '\u001b[31m' if day == 7 else '\u001b[0m' print(color + str(day).rjust(2) + ' ' + '\u001b[0m', end='') print() print_calendar(2023, 4)
- Printing a colored gradient:
for i in range(30): print('\u001b[38;2;255;{};0mâ–ˆ'.format(i * 255 // 29) * 30, end='') print('\u001b[0m')
- Printing a colored histogram:
import random def print_histogram(data): max_value = max(data) for value in data: bar_length = int(value / max_value * 50) bar = '#' * bar_length if value >= 80: color_code = '\033[1;32m' # green elif value >= 50: color_code = '\033[1;33m' # yellow else: color_code = '\033[1;31m' # red print(f"{color_code}{bar:>50}\033[0m") # Example usage: data = [random.randint(0, 100) for i in range(10)] print_histogram(data)
Bonus Examples
- Creating a rainbow effect by printing text in different colors repeatedly:
import time colors = ['\033[31m', '\033[33m', '\033[32m', '\033[36m', '\033[34m', '\033[35m'] while True: for color in colors: print(color + "Hello, world!", end='') time.sleep(0.2) print('\033[0m') # reset the color
- Printing a progress bar using colored blocks:
import time total = 100 progress = 0 while progress <= total: print('\r' + 'â–ˆ' * progress + '-' * (total - progress), end='') print(f' {progress}/{total}', end='', flush=True) progress += 1 time.sleep(0.1)
- Creating a color-changing background by printing spaces in different colors:
import random import time colors = ['\033[41m', '\033[42m', '\033[43m', '\033[44m', '\033[45m', '\033[46m'] while True: for i in range(100): print(random.choice(colors) + ' ' * 100, end='') time.sleep(0.01) print('\033[0m') # reset the color
- Displaying a flashing warning message in red:
import time while True: print('\033[31m' + 'WARNING: SYSTEM OVERHEATING!' + '\033[0m', end='') time.sleep(0.5) print('\r' + ' ' * 30, end='') time.sleep(0.5)
- Creating a random color generator:
import random while True: color_code = '\033[38;2;{};{};{}m'.format(random.randint(0, 255), random.randint(0, 255), random.randint(0, 255)) print(color_code + 'Hello, world!', end='') print('\033[0m') # reset the color time.sleep(0.5)
- Displaying a message in a rainbow of colors:
import time colors = ['\033[31m', '\033[33m', '\033[32m', '\033[36m', '\033[34m', '\033[35m'] message = "RAINBOW MESSAGE!" for i in range(len(message)): print(colors[i % len(colors)] + message[i], end='') print('\033[0m') # reset the color
- Printing a Christmas tree with colored decorations:
import random tree = [ ' * ', ' *** ', ' ***** ', ' ******* ', ' ********* ', ' *********** ', ' ************* ', ' *************** ', ' ||| ', ' ||| ', ' ||| ', ' ||| ', ' ||| ', ' ||| ', ' * ||| * ', ' *** ||| *** ', '***** ||| *****', '||***|||||||||***|||', '||***|||||||||***|||', '||***|||||||||***|||',] for i in range(len(tree)): line = tree[i]
In conclusion, ANSI escape codes provide a powerful tool for manipulating text in the console window, allowing for more dynamic and visually appealing output in command-line programs. By using these codes, developers can control the color, formatting, and position of text, and even create animations or progress bars. It’s important to note that not all terminal emulators or operating systems support all ANSI escape codes, so it’s important to test your code on different platforms to ensure compatibility. Overall, mastering the use of ANSI escape codes can greatly enhance the functionality and aesthetic appeal of command-line programs.