The os
module in Python provides a way to interact with the operating system, allowing you to perform various tasks related to file and directory management, process management, environment variables, and more. It serves as a powerful tool for working with the underlying operating system in a cross-platform manner. Let’s explore the main functionalities of the os
module with examples.
To begin, you need to import the os
module in your Python script:
import os
- Working with Files and Directories:
1.1. Creating Directories: You can use the mkdir()
function to create a new directory. It takes the directory path as an argument and creates the directory in the specified location.
os.mkdir('new_directory')
1.2. Changing Directories: The chdir()
function allows you to change the current working directory to the specified path.
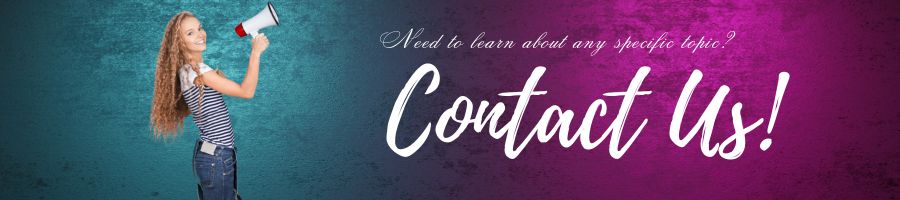
os.chdir('new_directory')
1.3. Getting Current Directory: You can use the getcwd()
function to retrieve the current working directory.
current_dir = os.getcwd() print(current_dir)
1.4. Listing Files and Directories: The listdir()
function returns a list of files and directories in the specified directory.
files = os.listdir('.') for file in files: print(file)
1.5. Removing Files and Directories: To remove a file, you can use the remove()
function, which deletes the file at the specified path. For directories, you can use the rmdir()
function, but the directory must be empty. If you want to remove a directory along with its contents, you can use the rmtree()
function from the shutil
module.
os.remove('file.txt') os.rmdir('empty_directory')
- Working with Paths:
The os.path
submodule provides various functions to manipulate and analyze file paths.
2.1. Joining Paths: The join()
function joins multiple path components into a single path.
path = os.path.join('dir1', 'dir2', 'file.txt') print(path)
2.2. Getting the Basename: The basename()
function returns the base name of a file path.
file_path = '/path/to/file.txt' basename = os.path.basename(file_path) print(basename)
2.3. Getting the Directory Name: The dirname()
function returns the directory name of a file path.
file_path = '/path/to/file.txt' dirname = os.path.dirname(file_path) print(dirname)
2.4. Splitting the Extension: The splitext()
function splits a file path into the base name and extension.
file_path = '/path/to/file.txt' base_name, extension = os.path.splitext(file_path) print(base_name) print(extension)
- Working with Processes:
3.1. Running System Commands: The system()
function allows you to run a command in the system shell.
os.system('ls')
3.2. Running Commands and Getting Output: The popen()
function runs a command in the system shell and returns a file-like object that allows you to read the command’s output.
output = os.popen('ls').read() print(output)
- Working with Environment Variables:
4.1. Getting Environment Variables: The environ
dictionary contains the current environment variables. You can access them using the get()
method.
# Get the value of a specific environment variable home_dir = os.environ.get('HOME') print(home_dir)
4.2. Setting Environment Variables: You can set environment variables using the environ
dictionary.
# Set a new environment variable os.environ['MY_VAR'] = 'my_value'
4.3. Removing Environment Variables: To remove an environment variable, you can use the pop()
method on the environ
dictionary.
# Remove an environment variable os.environ.pop('MY_VAR')
- Platform-Specific Features:
The os
module provides functions to work with platform-specific features, such as executing commands, accessing the file system, and more.
5.1. Checking the Current Operating System: The name
attribute returns the name of the operating system.
if os.name == 'posix': print("Running on a POSIX system (Linux, macOS, etc.)") elif os.name == 'nt': print("Running on Windows")
5.2. Executing Platform-Specific Commands: The system()
function can be used to execute platform-specific commands.
if os.name == 'posix': os.system('ls') elif os.name == 'nt': os.system('dir')
These are some of the key features provided by the os
module. By leveraging these functions, you can perform a wide range of tasks related to file and directory management, process management, and working with environment variables in a cross-platform manner.
Tips and Tricks:
- When working with file paths, it’s recommended to use the
os.path
submodule to ensure compatibility across different operating systems. - You can use the
os.path.exists()
function to check if a file or directory exists before performing operations on it. - The
os.path.isdir()
andos.path.isfile()
functions can be used to check if a path is a directory or a file, respectively. - If you need to perform more advanced file operations, such as copying, moving, or renaming files, consider using the
shutil
module, which provides higher-level file operations built on top of theos
module.
Remember to refer to the official Python documentation for more detailed information on the os
module: https://docs.python.org/3/library/os.html