In Python, tuples are an ordered and immutable collection of elements enclosed in parentheses (). Tuples are similar to lists, but they cannot be modified once created. However, tuples support several operations, methods, and functions that can be used to work with them.
Operations on tuples include indexing, slicing, concatenation, and repetition. Indexing allows you to access individual elements of a tuple by their position, while slicing allows you to access a range of elements. Concatenation allows you to combine two or more tuples into a new tuple, and repetition allows you to create a new tuple by repeating an existing tuple a specified number of times.
Methods are built-in functions that are specific to tuples and can be called on a tuple object. Some commonly used tuple methods include count(), which returns the number of occurrences of a specified element in the tuple, and index(), which returns the index of the first occurrence of a specified element in the tuple.
Functions are built-in functions that can be used to perform various operations on tuples. Some commonly used tuple functions include len(), which returns the number of elements in the tuple, max(), which returns the largest element in the tuple, and min(), which returns the smallest element in the tuple.
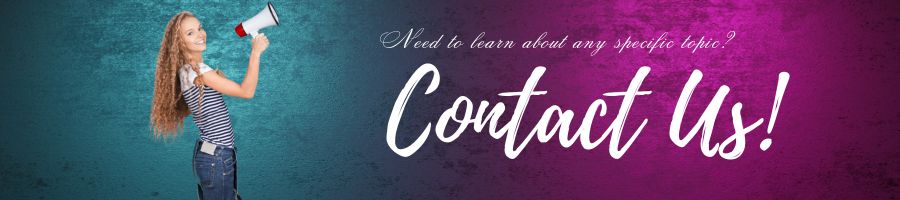
Overall, operations, methods, and functions provide a range of tools for working with tuples in Python, allowing you to manipulate, analyze, and process data stored in tuples.
Tuple Operations
Tuples support several operations, including concatenation, slicing, deleting and repetition.
Concatenation
Python tuple concatenation is a process of combining two or more tuples into a single tuple. Concatenation is done using the + operator. The resulting tuple contains all the elements from the original tuples, in the order in which they were combined. Here’s an example:
tuple1 = (1, 2, 3) tuple2 = (4, 5, 6) concatenated_tuple = tuple1 + tuple2 print(concatenated_tuple) # Output: (1, 2, 3, 4, 5, 6)
In this example, the two tuples tuple1
and tuple2
are concatenated using the + operator, resulting in a new tuple concatenated_tuple. The new tuple contains all the elements from tuple1
followed by all the elements from tuple2
. You can also concatenate more than two tuples by chaining the + operator. For example:
tuple1 = (1, 2, 3) tuple2 = (4, 5, 6) tuple3 = (7, 8, 9) concatenated_tuple = tuple1 + tuple2 + tuple3 print(concatenated_tuple) # Output: (1, 2, 3, 4, 5, 6, 7, 8, 9)
In this example, three tuples tuple1
, tuple2
, and tuple3
are concatenated using the + operator to create a new tuple concatenated_tuple
.
It’s important to note that tuple concatenation creates a new tuple and does not modify the original tuples. This is because tuples are immutable, which means that their elements cannot be changed once they are created.
Tuple concatenation is a useful technique in Python for combining and organizing data stored in tuples. Combining multiple tuples into a single tuple can simplify your code and make it more efficient for data processing and analysis.
Slicing
Tuple slicing is a technique that allows you to access a subset of elements from a tuple. You can use slice notation to specify the range of elements you want to retrieve. Slice notation consists of two indices separated by a colon within square brackets.
The first index indicates the starting position of the slice, while the second index indicates the ending position of the slice. The ending position is exclusive, meaning that the element at the ending index is not included in the slice. For example, consider the following tuple:
my_tuple = (1, 2, 3, 4, 5, 6, 7, 8, 9, 10) # If you want to retrieve the first three elements of the tuple, you can use slice notation as follows: my_slice = my_tuple[0:3] print(my_slice) # Output: (1, 2, 3)
You can also omit the starting index or ending index to indicate the beginning or end of the tuple, respectively. For example:
my_slice = my_tuple[:5] # Get the first 5 elements print(my_slice) # Output: (1, 2, 3, 4, 5) my_slice = my_tuple[5:] # Get all elements from index 5 to the end print(my_slice) # Output: (6, 7, 8, 9, 10)
You can also specify a step value in the slice notation. The step value determines the interval between elements to be included in the slice. For example:
my_slice = my_tuple[::2] # Get every second element print(my_slice) # Output: (1, 3, 5, 7, 9)
Here, the slice starts from the beginning of the tuple, ends at the end of the tuple, and includes every second element. Tuple slicing is a powerful technique that allows you to extract specific subsets of data from a tuple, making it a useful tool for data processing and analysis in Python.
Repetition
Python tuple repetition is a technique for creating a new tuple by repeating an existing tuple a specified number of times. This is done using the * operator. Here’s an example:
my_tuple = (1, 2, 3) repeated_tuple = my_tuple * 3 print(repeated_tuple) # Output: (1, 2, 3, 1, 2, 3, 1, 2, 3)
In this example, the tuple my_tuple
is repeated three times using the * operator, resulting in a new tuple repeated_tuple
that contains three copies of the original tuple. You can also repeat an empty tuple to create a new empty tuple:
empty_tuple = () repeated_empty_tuple = empty_tuple * 3 print(repeated_empty_tuple) # Output: ()
In this example, the empty tuple is repeated three times, resulting in a new empty tuple.
It’s important to note that tuple repetition creates a new tuple and does not modify the original tuple. This is because tuples are immutable, which means that their elements cannot be changed once they are created.
Tuple repetition is a useful technique in Python for creating tuples with repeated elements, such as for initializing a tuple with a fixed number of identical elements. By repeating an existing tuple, you can simplify your code and make it more efficient for data processing and analysis.
Tuple Methods
Tuples have several built-in methods that can be used to manipulate them.
count():
The count() method returns the number of times a specified element appears in the tuple. The syntax for the count()
method is as follows:
tuple_name.count(element)
Here, tuple_name
is the name of the tuple on which the count operation is performed and element
is the element to be counted. Here’s an example:
my_tuple = (1, 2, 3, 1, 1, 4, 5, 1) count = my_tuple.count(1) print(count) # Output: 4
In this example, the count()
method is used to count the number of times the element 1
appears in the tuple my_tuple
. The output is 4 because 1
appears four times in the tuple.
index():
The index()
method returns the index of the first occurrence of a specified element in the tuple. The syntax for the index()
method is as follows:
tuple_name.index(element)
Here, tuple_name
is the name of the tuple on which the index operation is performed and element
is the element to be searched. Here’s an example:
my_tuple = (1, 2, 3, 1, 1, 4, 5, 1) index = my_tuple.index(4) print(index) # Output: 5
In this example, the index()
method is used to find the index of the first occurrence of the element 4
in the tuple my_tuple
. The output is 5 because the index of 4
in the tuple is 5.
If the specified element is not found in the tuple, the index()
method will raise a ValueError
exception. For example:
my_tuple = (1, 2, 3, 1, 1, 4, 5, 1) index = my_tuple.index(6) # Raises ValueError: tuple.index(x): x not in tuple
In this example, the index()
method is used to find the index of the element 6
in the tuple my_tuple
. Since 6
is not present in the tuple, a ValueError
exception is raised.
Tuple Functions
In addition to methods, Python provides several built-in functions that can be used with tuples.
len()
The len() function returns the number of elements in a tuple. For example:
my_tuple = (1, 2, 3, 4, 5) print(len(my_tuple)) # Output: 5
max()
The max() function returns the maximum element in a tuple. The elements in the tuple must be of the same type and support comparison. For example:
my_tuple = (1, 2, 3, 4, 5) print(max(my_tuple)) # Output: 5
min()
The min() function returns the minimum element in a tuple. The elements in the tuple must be of the same type and support comparison. For example:
my_tuple = (1, 2, 3, 4, 5) print(min(my_tuple)) # Output: 1
sum()
The sum() function returns the sum of all elements in a tuple. The elements in the tuple must be numeric. For example:
my_tuple = (1, 2, 3, 4, 5) print(sum(my_tuple)) # Output: 15
tuple()
The tuple() function can be used to convert other data structures into tuples. For example:
my_list = [1, 2, 3, 4, 5] my_tuple = tuple(my_list) print(my_tuple) # Output: (1, 2, 3, 4, 5)
sorted()
The sorted()
function is used to sort the elements of a tuple in ascending order. The syntax for using sorted()
with a tuple is as follows:
sorted_tuple = sorted(my_tuple)
Here, my_tuple
is the tuple that needs to be sorted, and sorted_tuple
is the sorted tuple. For example:
my_tuple = (3, 1, 4, 1, 5, 9, 2, 6, 5, 3, 5) sorted_tuple = sorted(my_tuple) print(sorted_tuple) # Output: [1, 1, 2, 3, 3, 4, 5, 5, 5, 6, 9]
In this example, the sorted()
function is used to sort the elements of the tuple my_tuple
.
enumerate()
The enumerate()
function is used to iterate over a tuple and return the index and value of each element as a tuple. The syntax for using enumerate()
with a tuple is as follows:
for index, value in enumerate(my_tuple): # do something with index and value
Here, my_tuple
is the tuple that needs to be iterated over, and index
and value
are the index and value of each element in the tuple. For example:
my_tuple = ('apple', 'banana', 'cherry') for index, value in enumerate(my_tuple): print(index, value) # Output: # 0 apple # 1 banana # 2 cherry
In this example, the enumerate()
function is used to iterate over the elements of the tuple my_tuple
and print the index and value of each element.
any()
The any()
function is used to check if any element in a tuple is True
. The syntax for using any()
with a tuple is as follows:
result = any(my_tuple)
Here, my_tuple
is the tuple that needs to be checked, and result
is a Boolean value that indicates whether any element in the tuple is True
. For example:
my_tuple = (False, False, True, False) result = any(my_tuple) print(result) # Output: True
In this example, the any()
function is used to check if any element in the tuple my_tuple
is True
. Since the third element in the tuple is True
, the result is True
.
all()
The all()
function is used to check if all elements in a tuple are True
. The syntax for using all()
with a tuple is as follows:
result = all(my_tuple)
Here, my_tuple
is the tuple that needs to be checked, and result
is a Boolean value that indicates whether all elements in the tuple are True
. For example:
my_tuple = (True, True, False, True) result = all(my_tuple) print(result) # Output: False
In this example, the all()
function is used to check if all elements in the tuple my_tuple
are True
. Since the third element in the tuple is False
, the result is False
.
Conclusion
Tuples are a useful data structure in Python that allow for ordered, immutable collections of elements. They have several operations, methods, and functions available that can be used to manipulate and analyze them. By understanding these capabilities, you can take full advantage of the power and flexibility of Python tuples in your code.