Python’s import
statement is a crucial feature that allows programmers to include code from other modules or packages into their programs. The import
statement is used to import functions, classes, and variables from other modules and packages, making it a fundamental tool for building complex applications in Python.
3 Types of Basic Import Syntax
1: The basic syntax for importing modules is as follows:
import module_name
This statement imports the module module_name
and makes all the functions, classes, and variables in the module available in the current program. Example:
# Import the math module import math # Use the pi constant from the math module radius = 5 area = math.pi * radius**2 print(area) # Output: 78.53981633974483
2: If you only need to import a specific function, class, or variable from a module, you can use the following syntax:
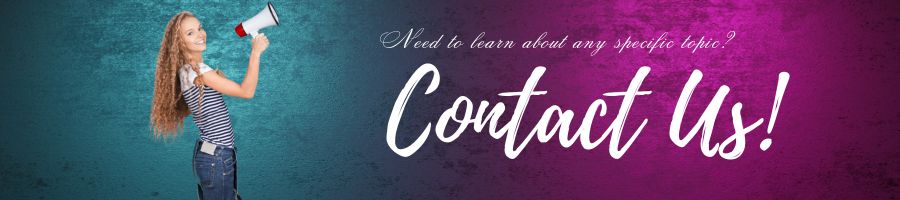
from module_name import function_name, class_name, variable_name
This statement imports only the specified names from the module and makes them available in the current program. Example:
# Import the sqrt function from the math module from math import sqrt # Use the sqrt function to find the square root of 25 result = sqrt(25) print(result) # Output: 5.0
3: You can also give the imported names different names in your program using the as
keyword:
from module_name import function_name as fn, class_name as cn, variable_name as vn
This statement imports the specified names from the module and gives them the alternative names fn
, cn
, and vn
, respectively, in your program. Example:
# Import the sqrt function from the math module and give it a different name from math import sqrt as square_root # Use the square_root function to find the square root of 36 result = square_root(36) print(result) # Output: 6.0
Importing Packages
In Python, a package is a collection of modules that are organized in a directory hierarchy. To import a package, you use the same syntax as for importing a module:
import package_name
This statement imports the package package_name
and makes all the modules in the package available in the current program. To access the functions, classes, and variables in the modules, you need to specify the module name and the name of the item you want to use, separated by a dot:
package_name.module_name.function_name()
Example:
# Import the datetime module from the datetime package import datetime.datetime # Use the now() function from the datetime module to get the current date and time now = datetime.datetime.now() print(now) # Output: 2022-11-06 20:30:00.000000
Absolute and Relative Imports
Python supports two types of import statements: absolute and relative. An absolute import specifies the full path to the module or package you want to import, starting from the root of your Python environment. For example:
import my_package.my_module
This statement imports the module my_module
from the package my_package
, which is located at the root level of your Python environment.
A relative import specifies the path to the module or package relative to the current module or package. For example:
from . import my_module
This statement imports the module my_module
from the current package, where the dot .
refers to the current directory. Suppose here’s an example folder structure, lets see how relative imports work:
my_package/ ├── __init__.py ├── my_module.py └── subpackage/ ├── __init__.py ├── sub_module.py └── sibling_module.py
In this example, the my_package
directory contains the __init__.py
file, which makes it a Python package. The my_module.py
file contains a function or class that you want to import in another module.
The subpackage
directory is a subpackage of my_package
, and it also contains an __init__.py
file that makes it a package. The sub_module.py
file contains a function or class that you want to import within the subpackage
. The sibling_module.py
file contains a function or class that you want to import from a module that is in a different package, but at the same level in the directory hierarchy as my_package
.
# Importing from a relative path from . import my_module # Importing from a subpackage from . import subpackage # Importing from a sibling module from .. import sibling_module
In this example, the first import statement imports the my_module
module from the current package, using a relative import. The dot before the module name indicates that the module should be searched for in the current package.
The second import statement imports the subpackage
module from the current package, also using a relative import.
The third import statement imports the sibling_module
module from the parent package of the current package, using a relative import with two dots. The number of dots indicates how many levels up in the package hierarchy to search for the module.
The __init__.py
File
In order for a directory to be recognized as a package in Python, it must contain a special file called __init__.py
. This file can be empty or contain initialization code for the package. The __init__.py
file is executed when the package is imported and can set up the package environment, import sub-packages and modules, and define variables and functions that are available to other modules in the package.
Importing Modules from Different Directories
Sometimes, you may need to import modules from different directories or folders. You can do this by adding the directory path to the Python search path using the sys.path
variable. Example:
import sys sys.path.append('/path/to/my/module') import my_module
This statement appends the directory /path/to/my/module
to the Python search path and imports the my_module
module from that directory.
Handling Import Errors
When importing modules, it’s possible to encounter various types of errors, such as ImportError
(module not found), SyntaxError
(invalid syntax in the module), or AttributeError
(module does not have the specified attribute).
To handle import errors, you can use a try
/except
block to catch the error and take appropriate action. For example, you might print an error message, log the error, or fallback to a different module. Here’s an example:
try: import my_module except ImportError: print('Could not import my_module')
Dealing with Version Conflicts
In some cases, you may encounter version conflicts when importing modules, where two or more modules require different versions of the same package or module. This can cause errors or unexpected behavior in your program.
To deal with version conflicts, you can use tools like virtualenv
or conda
to create isolated environments for your Python projects. These environments allow you to install and manage specific versions of packages and modules without affecting other projects on your system.
Another approach is to use a package manager like pip
to manage your dependencies and ensure that you have compatible versions of all required packages.
Overall, handling version conflicts requires careful planning and management of your dependencies to ensure that your code runs smoothly and without unexpected errors.
Docstrings and Importing Modules
It is a good practice to include docstrings in your module to provide information about the module, its functions, classes, and variables. Docstrings are enclosed in triple quotes and placed at the beginning of the module, function, class, or variable definition. Example:
""" This is a module that contains functions for calculating the area and circumference of a circle. """ import math def area(radius): """ This function calculates the area of a circle with the given radius. """ return math.pi * radius ** 2 def circumference(radius): """ This function calculates the circumference of a circle with the given radius. """ return 2 * math.pi * radius
When you import a module, Python automatically loads the module’s docstrings into memory and makes them available through the built-in help()
function:
import my_module help(my_module)
This statement displays the docstring for the my_module
module.
Best Practices
Here are some best practices to follow when using the import
statement in Python:
- Always import modules at the beginning of your program or module.
- Use absolute imports for better clarity and to avoid name conflicts.
- Use docstrings to provide information about your module, functions, classes, and variables.
- Avoid using wildcard imports (
from module import *
) as they can make your code less readable and harder to maintain. - Avoid circular imports, where two or more modules or packages import each other in a loop, as they can cause errors and slow down your code.
- Import only the modules, functions, classes, and variables that you need. This makes your code more readable and reduces the risk of naming conflicts.
- Use clear and descriptive module and package names to avoid confusion and make your code more understandable.
- Organize your modules and packages into logical groups and hierarchies to improve the maintainability and scalability of your code.
Organize Python Imports
As a good Python developer, you should organize your python imports. Lets see, How you might organize or group different types of import statements in your Python code:
# Standard library imports import os import sys # Third-party imports import numpy as np import pandas as pd # Local application imports from my_package import my_module from my_package.utils import my_utility_function
In this example, we group our import statements into three categories:
- Standard library imports: These are Python modules that are included in the standard library and don’t require any external installation. Examples include
os
andsys
. - Third-party imports: These are modules that are not included in the standard library and need to be installed separately, usually using a package manager like
pip
. Examples includenumpy
andpandas
. - Local application imports: These are modules or packages that you’ve written yourself and are part of your application codebase. You can organize them into packages and subpackages, just like you would with third-party packages. In this example, we import a module called
my_module
from a package calledmy_package
, and a function calledmy_utility_function
from a module calledutils
within themy_package
package.
By organizing your import statements like this, you can make it clear where each import comes from and reduce the risk of naming conflicts or errors caused by circular dependencies. It also makes it easier for other developers to understand your code and for you to maintain it in the future.
There’s no one “right” way to organize your imports in Python, as it often depends on the specific needs and conventions of your project or team. However, here are a few additional tips that can help you organize your imports more effectively:
- Sort your imports: Within each import group (standard library, third-party, and local), you should sort your imports alphabetically by module name. This makes it easier to find a specific import and helps avoid duplicates.
- Use absolute imports: When importing from within your own project, use absolute imports rather than relative imports (e.g.
from my_project.my_module import my_function
instead offrom .my_module import my_function
). This makes it easier to move modules around without breaking imports. - Use import aliases: If a module name is long or unwieldy, you can use an import alias to give it a shorter name. For example,
import pandas as pd
makes it easier to use thepandas
module without having to type out its full name every time. - Group related imports: Within each import group, you can further group related imports together. For example, you might group all of your Django-related imports together, or all of your database-related imports together.
Here’s an example of what a more complete set of import statements might look like, using these tips:
# Standard library imports import logging import os import sys # Third-party imports import numpy as np import pandas as pd # Local application imports from my_project.models import User, Post from my_project.utils import ( get_config, send_email, write_to_file, ) from my_project.web import app, db, routes # Third-party library imports with import aliases import django.db as db import matplotlib.pyplot as plt
In this example, we’ve sorted our imports alphabetically within each group, used absolute imports, and grouped related imports together. We’ve also used import aliases to make the django.db
module easier to use, and used parentheses to group related functions within the my_project.utils
module.
Performance
Python’s import mechanism is relatively fast, but it can become slow if you have a large number of imports or if you are importing modules from different directories. To improve import performance, you can use tools like importlib
and zipimport
.
Tips and Tricks
Here are some tips and tricks to help you work with the import
statement:
- Use the
dir()
function to list the contents of a module or package. - Use the
__name__
variable to determine if a module is being imported or run as the main program. - Use the
__file__
variable to get the path of the current module or package. - Use the
sys.modules
dictionary to get a dictionary of all loaded modules.
Conclusion
The import
statement is an essential part of Python programming that allows you to bring external modules and packages into your code. By understanding the different types of imports and how to use them effectively, you can write more modular, maintainable, and scalable code. Remember to follow best practices like grouping your imports, using absolute imports, and sorting your imports alphabetically, and to choose descriptive module and package names to avoid naming conflicts. With these tips in mind, you’ll be able to write Python code that is both efficient and easy to understand.