Set methods are built-in functions or operations that can be performed on sets in Python. These methods provide a convenient and efficient way to manipulate sets, add or remove elements, and perform set operations such as union, intersection, and difference.
Some common set methods in Python include add(), remove(), union(), intersection(), difference(), and issubset(). Each of these methods has a specific functionality and can be used to perform different operations on sets.
In addition to these common methods, Python sets have several other built-in methods that allow for various operations on sets. These methods include issuperset(), pop(), symmetric_difference(), symmetric_difference_update(), update(), and others.
By learning about these set methods, programmers can use Python sets to their full potential and effectively manipulate and work with sets in their Python programs. In this article, we will explore the different methods that can be performed on sets in Python.
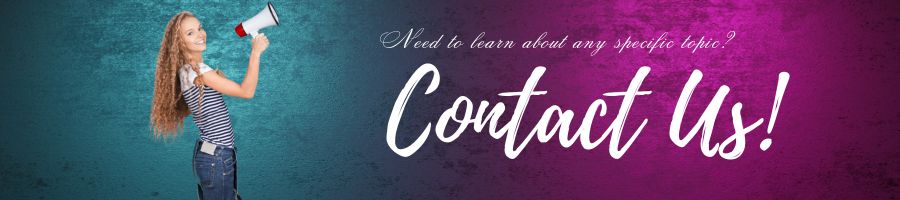
add()
The add() method adds an element to the set. If the element is already present in the set, it does not add it again. Example:
>>> my_set = {1, 2, 3} >>> my_set.add(4) >>> print(my_set) {1, 2, 3, 4}
clear()
The clear() method removes all the elements from the set. Example:
>>> my_set = {1, 2, 3} >>> my_set.clear() >>> print(my_set) set()
copy()
The copy() method returns a shallow copy of the set. Example:
>>> my_set = {1, 2, 3} >>> new_set = my_set.copy() >>> print(new_set) {1, 2, 3}
difference()
The difference() method returns a set containing the elements that are present in the first set but not in the second set. Example:
>>> set1 = {1, 2, 3} >>> set2 = {3, 4, 5} >>> print(set1.difference(set2)) {1, 2}
difference_update()
The difference_update() method removes the elements that are present in both sets from the first set. Example:
>>> set1 = {1, 2, 3} >>> set2 = {3, 4, 5} >>> set1.difference_update(set2) >>> print(set1) {1, 2}
discard()
The discard() method removes the specified element from the set. If the element is not present in the set, it does not raise an error. Example:
>>> my_set = {1, 2, 3} >>> my_set.discard(2) >>> print(my_set) {1, 3}
intersection()
The intersection() method returns a set containing the elements that are common to both sets. Example:
>>> set1 = {1, 2, 3} >>> set2 = {3, 4, 5} >>> print(set1.intersection(set2)) {3}
intersection_update()
The intersection_update() method removes the elements that are not present in both sets from the first set. Example:
>>> set1 = {1, 2, 3} >>> set2 = {3, 4, 5} >>> set1.intersection_update(set2) >>> print(set1) {3}
isdisjoint()
The isdisjoint() method returns True if two sets have no common elements, and False otherwise. Example:
>>> set1 = {1, 2, 3} >>> set2 = {4, 5, 6} >>> set3 = {3, 4, 5} >>> print(set1.isdisjoint(set2)) True >>> print(set1.isdisjoint(set3)) False
issubset()
The issubset() method returns True if all elements of a set are present in another set. If the set is not a subset of the other set, the method returns False. Example:
>>> set1 = {1, 2, 3} >>> set2 = {1, 2} >>> print(set2.issubset(set1)) True
In the example above, set2
is a subset of set1
, because all elements of set2
(1 and 2) are present in set1
. Therefore, set2.issubset(set1)
returns True. The issubset()
method can also be used with other collection types, such as lists and tuples:
>>> set1 = {1, 2, 3} >>> list1 = [1, 2] >>> print(set(list1).issubset(set1)) True
In this example, we convert list1
to a set using set(list1)
and then use the issubset()
method to check if all elements of list1
are present in set1
. Since all elements of list1
are present in set1
, the method returns True. It’s important to note that a set is always a subset of itself:
>>> set1 = {1, 2, 3} >>> print(set1.issubset(set1)) True
In summary, the issubset()
method is useful for checking if a set is a subset of another set or collection. It can help programmers write more efficient and concise code when working with sets in Python.
issuperset()
The issuperset() method returns True if all elements of a set are present in another set. Example:
>>> set1 = {1, 2, 3} >>> set2 = {1, 2} >>> print(set1.issuperset(set2)) True
pop()
The pop() method removes and returns an arbitrary element from the set. If the set is empty, it raises an error. Example:
>>> my_set = {1, 2, 3} >>> print(my_set.pop()) 1 >>> print(my_set) {2, 3}
remove()
The remove() method removes the specified element from the set. If the element is not present in the set, it raises an error. Example:
>>> my_set = {1, 2, 3} >>> my_set.remove(2) >>> print(my_set) {1, 3}
symmetric_difference()
The symmetric_difference() method returns a set containing the elements that are present in either of the sets, but not in both. Example:
>>> set1 = {1, 2, 3} >>> set2 = {3, 4, 5} >>> print(set1.symmetric_difference(set2)) {1, 2, 4, 5}
symmetric_difference_update()
The symmetric_difference_update() method updates the first set with the elements that are present in either of the sets, but not in both. Example:
>>> set1 = {1, 2, 3} >>> set2 = {3, 4, 5} >>> set1.symmetric_difference_update(set2) >>> print(set1) {1, 2, 4, 5}
union()
The union() method returns a set containing all the elements that are present in either of the sets. Example:
>>> set1 = {1, 2, 3} >>> set2 = {3, 4, 5} >>> print(set1.union(set2)) {1, 2, 3, 4, 5}
update()
The update() method updates the first set with the elements that are present in both sets. Example:
>>> set1 = {1, 2, 3} >>> set2 = {3, 4, 5} >>> set1.update(set2) >>> print(set1) {1, 2, 3, 4, 5}
Conclusion
In this article, we explored the different methods that can be performed on sets in Python. These methods allow us to add, remove, and modify elements in sets, as well as perform mathematical operations on them. Understanding these methods can help you use sets effectively in your Python programs.