A set object is an unordered collection of distinct hashable objects. Common uses include membership testing, removing duplicates from a sequence, and computing mathematical operations such as intersection, union, difference, and symmetric difference. In this article, we’ll explore Python sets in detail, including their operations, methods, and examples.
Creating a Set
To create a set in Python, you can use the set() function. You can pass a sequence (e.g., list, tuple, string) to this function, and it will return a set containing the unique elements of that sequence. Here’s an example:
>>> my_set = set([1, 2, 3, 2]) >>> print(my_set) # {1, 2, 3}
In this example, we pass a list [1, 2, 3, 2] to the set() function. The function returns a set containing the unique elements of the list.
Alternatively, you can create a set using curly braces {} and separate the elements with commas. However, this approach won’t work for creating an empty set because empty curly braces are reserved for creating an empty dictionary. To create an empty set, you need to use the set() function, as follows:
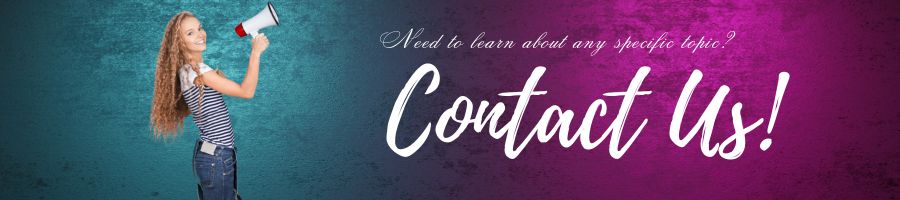
>>> my_set = {1, 2, 3} >>> print(my_set) {1, 2, 3} >>> empty_set = set() >>> print(empty_set) set()
Accessing Elements in a Set
Since sets are unordered collections, you cannot access their elements by index. Instead, you can check if an element exists in a set using the “in” operator. Here’s an example:
>>> my_set = {1, 2, 3} >>> print(1 in my_set) True >>> print(4 in my_set) False
Adding Elements to a Set
You can add elements to a set using the add() method. Here’s an example:
>>> my_set = {1, 2, 3} >>> my_set.add(4) >>> print(my_set) {1, 2, 3, 4}
Alternatively, you can use the update() method to add multiple elements to a set. Here’s an example:
>>> my_set = {1, 2, 3} >>> my_set.update([3, 4, 5]) >>> print(my_set) {1, 2, 3, 4, 5}
Removing Elements from a Set
You can remove elements from a set using the remove() method. Here’s an example:
>>> my_set = {1, 2, 3} >>> my_set.remove(2) >>> print(my_set) {1, 3}
If you try to remove an element that doesn’t exist in the set, the remove() method will raise a KeyError. To avoid this, you can use the discard() method, which removes the element if it exists in the set and does nothing otherwise. Here’s an example:
>>> my_set = {1, 2, 3} >>> my_set.discard(2) >>> print(my_set) {1, 3} >>> my_set.discard(2) >>> print(my_set) {1, 3}
You can also use the pop() method to remove and return an arbitrary element from the set. However, since sets are unordered collections, you cannot predict which element will be removed. Here’s an example:
>>> my_set = {1, 2, 3} >>> popped_element = my_set.pop() >>> print(popped_element) 1 >>> print(my_set)
Sets Time & Space Complexity
Combined time and space complexity table for Python sets and their operations:
Operation | Time Complexity | Space Complexity | Description |
---|---|---|---|
Create a Set | O(n) | O(n) | Build a set from an iterable with n elements. |
Add an Element | O(1) on average | O(n) | Insert a new element; hash-based insertion. |
Remove an Element | O(1) on average | O(n) | Remove an element with remove() or discard() . |
Membership Test | O(1) on average | O(n) | Check if an element exists (x in my_set ). |
Union (|) | O(len(s1) + len(s2)) | O(len(s1) + len(s2)) | O(n1 + n2) |
Intersection (& ) | O(min(len(s1), len(s2))) | O(min(n1, n2)) | Find common elements; result size depends on the overlap. |
Difference (- ) | O(len(s1)) | O(n1) | Subtract one set from another; space depends on the size of the first set. |
Symmetric Difference (^ ) | O(len(s1) + len(s2)) | O(n1 + n2) | Find elements in either set but not both; space depends on total unique items. |
Why Sets?
Learning about Python sets can help developers write more efficient, concise, and readable code. Sets are also an important data structure in many data science and machine learning applications, where data needs to be processed and analyzed quickly and accurately.
In addition, understanding sets and their operations is essential for passing coding interviews and technical assessments for software engineering roles. Many interview questions and coding challenges require working with sets and performing set operations.
In summary, learning about Python sets can greatly enhance your programming skills and improve your ability to work with data. They are a valuable tool for developers, data scientists, and anyone working with large datasets or complex algorithms. As a next step, Learn about sets operations and methods using this link.