Exception handling is a fundamental concept in programming that allows developers to gracefully handle errors and unexpected events in their code. In Python, exception handling is implemented through a combination of the try
, except
, else
, and finally
keywords.
The try
and except
Blocks
The basic syntax for exception handling in Python is as follows:
try: # code that might raise an exception except ExceptionType1: # code to handle exceptions of type ExceptionType1 except ExceptionType2: # code to handle exceptions of type ExceptionType2 else: # code to run if no exceptions were raised in the try block finally: # code to run regardless of whether an exception was raised
The try
block contains the code that might raise an exception. If an exception is raised, Python immediately jumps to the first except
block whose exception type matches the raised exception. If there are no matching except
blocks, the exception is propagated up the call stack until it is handled by an appropriate except
block or the program terminates.
It’s important to note that a try
block can contain multiple lines of code that might raise exceptions. In this case, each line of code is checked for exceptions in turn, and the first exception that is raised will cause Python to jump to the appropriate except
block.
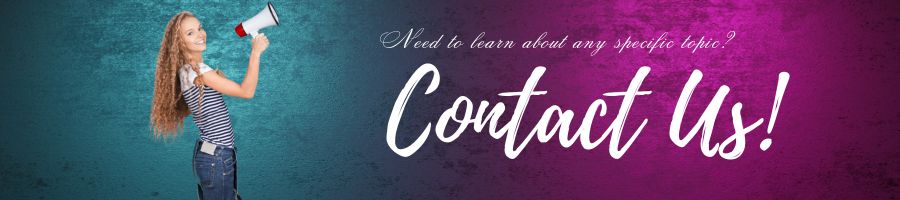
Handling Specific Exceptions
As shown in the example above, you can use multiple except
blocks to handle different types of exceptions. Each except
block should specify the type of exception it can handle using the ExceptionType
syntax. For example, to handle a TypeError
exception, you would use the following syntax:
try: # code that might raise a TypeError except TypeError: # code to handle the TypeError
You can also handle multiple exception types in a single except
block by using parentheses and separating the exception types with commas:
try: # code that might raise a TypeError or a ValueError except (TypeError, ValueError): # code to handle the TypeError or the ValueError
If you want to handle all types of exceptions in a single except
block, you can use the Exception
base class:
try: # code that might raise an exception except Exception: # code to handle the exception
The else
Block
The else
block is optional, and contains code that will run only if no exceptions were raised in the try
block. This block is often used to perform cleanup or finalization tasks that should be executed regardless of whether an exception occurred.
For example, if you’re working with a file that needs to be closed regardless of whether an exception was raised, you could use the following code:
try: f = open('filename.txt') # code that reads from or writes to the file except Exception: # code to handle the exception else: f.close()
In this example, the open()
function is called within the try
block, and the resulting file object is assigned to the variable f
. If an exception is raised while working with the file, the exception is handled by the appropriate except
block. If no exceptions are raised, the else
block runs and the file is closed using the close()
method.
The finally
Block
The finally
block is also optional, and contains code that will run regardless of whether an exception was raised in the try
block. This block is often used to perform cleanup or finalization tasks that should be executed regardless of whether an exception occurred.
For example, if you’re working with a file that needs to be closed regardless of whether an exception was raised, you could use the following code:
try: f = open('filename.txt') # code that reads from or writes to the file except Exception: # code to handle the exception finally: f.close()
In this example, the open()
function is called within the try
block, and the resulting file object is assigned to the variable f
. If an exception is raised while working with the file, the exception is handled by the appropriate except
block. If no exceptions are raised, the finally
block runs and the file is closed using the close()
method.
Raising Exceptions
Sometimes you may want to explicitly raise an exception to indicate that an error has occurred. This can be done using the raise
keyword.
The raise
keyword can be used on its own to re-raise the last exception that was caught, like this:
try: # code that might raise an exception except Exception as e: # code to handle the exception raise
You can also raise a new exception by specifying the exception type and an optional error message, like this:
try: # code that might raise an exception except Exception as e: # code to handle the exception raise ValueError('An error occurred: {}'.format(str(e)))
This code raises a ValueError
exception with a message that includes the original exception’s error message.
Custom Exception Classes
In addition to the built-in exception types provided by Python, you can also define your own custom exception classes. Custom exception classes are useful when you want to provide more specific information about the cause of an error, or when you want to distinguish between different types of errors that might occur in your code.
To define a custom exception class, you simply create a new class that inherits from the Exception
base class. For example:
class MyException(Exception): pass
This creates a new exception class called MyException
. You can then raise this exception in your code like any other exception:
try: # code that might raise an exception except Exception as e: # code to handle the exception raise MyException('An error occurred: {}'.format(str(e)))
Conclusion
Exception handling is an important aspect of programming in Python. By using the try
, except
, else
, and finally
keywords, you can gracefully handle errors and unexpected events in your code, and provide useful information to your users about what went wrong. Custom exception classes can also be used to provide more specific information about the cause of an error, or to distinguish between different types of errors that might occur in your code.