Python is a popular high-level programming language that is widely used for a variety of tasks, ranging from simple scripting to complex machine learning algorithms. One of the most powerful features of Python is the ability to work with iterators and generators. In this article, we will explore generator expressions in Python, a useful construct that allows for efficient and memory-safe creation of iterators.
What are Generator Expressions?
In Python, generator expressions are a concise way to create an iterator. They are similar to list comprehensions, but instead of creating a list, they create a generator object. A generator is a type of iterator that yields a value each time it is called. The difference between a list comprehension and a generator expression is that the former creates a list in memory, while the latter creates a generator object that produces the same values on the fly.
Syntax of Generator Expressions
The syntax for a generator expression is similar to that of a list comprehension. Here is the basic structure:
(generator expression) = (expression for variable in iterable if condition)
The generator expression is enclosed in parentheses and consists of an expression, a variable, an iterable, and an optional condition. The expression is evaluated each time the generator yields a value. The variable is used to iterate over the iterable, and the condition is used to filter the elements of the iterable. Examples
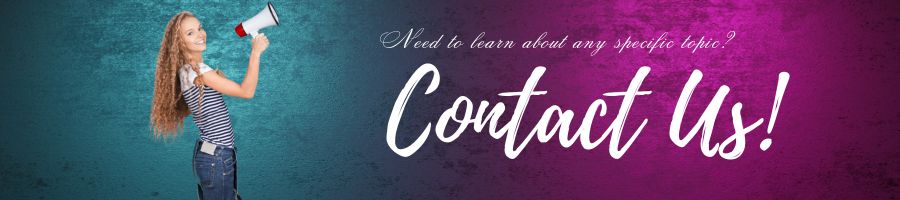
Here are some examples of generator expressions:
- Squares of numbers
squares = (x**2 for x in range(10)) print(list(squares)) # Output: [0, 1, 4, 9, 16, 25, 36, 49, 64, 81]
In this example, we create a generator expression that generates the squares of the first 10 numbers. We then convert the generator object to a list and print the result.
- Filtering even numbers
even_numbers = (x for x in range(10) if x % 2 == 0) print(list(even_numbers)) # Output: [0, 2, 4, 6, 8]
In this example, we create a generator expression that generates the even numbers from 0 to 9. We use the if clause to filter out the odd numbers.
- Cartesian product
cartesian_product = ((x, y) for x in range(3) for y in range(2)) print(list(cartesian_product)) # Output: [(0, 0), (0, 1), (1, 0), (1, 1), (2, 0), (2, 1)]
In this example, we create a generator expression that generates the Cartesian product of two ranges. We use two for loops to iterate over the two ranges.
Advanced Usage
Generator expressions are a powerful construct that can be used in many ways. Here are some advanced examples:
Infinite generator
def fibonacci(): a, b = 0, 1 while True: yield a a, b = b, a + b fibonacci_numbers = (x for x in fibonacci() if x < 100) print(list(fibonacci_numbers)) # Output: [0, 1, 1, 2, 3, 5, 8, 13, 21, 34, 55, 89]
In this example, we create an infinite generator that generates the Fibonacci sequence. We use the generator expression to generate the Fibonacci numbers that are less than 100.
Using generator expressions in functions
def sum_of_squares(numbers): return sum(x**2 for x in numbers) numbers = [1, 2, 3, 4, 5] result = sum_of_squares(numbers) print(result) # Output: 55
In this example, we create a function sum_of_squares
that takes a list of numbers and returns the sum of their squares. We use a generator expression to calculate the squares of each number in the list, and then use the sum
function to calculate their sum.
Processing large data sets:
Suppose you have a large list of integers that you need to process, but you don’t want to load the entire list into memory at once. Here’s how you could use a generator to read the list in chunks:
def process_data(data, chunk_size=1000): """Generator function to process a large list of data in chunks.""" i = 0 while i < len(data): yield data[i:i+chunk_size] i += chunk_size # Process the data large_data = list(range(10000000)) for chunk in process_data(large_data): # Process each chunk of data here print(f"Processed {len(chunk)} items")
In this example, we define a generator function process_data
that takes a list of data and a chunk size as input. The function reads the data in chunks of size chunk_size
using slicing, and yields each chunk of data as it is processed.
By using a generator to read the data in chunks, we can process the large list of data efficiently without having to load the entire list into memory at once.
Stream processing:
Suppose you want to read and process data from a network socket in real-time. Here’s how you could use a generator to implement a data stream:
import socket def receive_data(sock, chunk_size=1024): """Generator function to receive data from a network socket in real-time.""" while True: data = sock.recv(chunk_size) if not data: break yield data # Process the data sock = socket.socket(socket.AF_INET, socket.SOCK_STREAM) sock.connect(("localhost", 8080)) for chunk in receive_data(sock): # Process each chunk of data here print(f"Received {len(chunk)} bytes")
In this example, we define a generator function receive_data
that takes a network socket and a chunk size as input. The function reads data from the socket in chunks of size chunk_size
, yielding each chunk of data as it is received.
By using a generator to read the data from the network socket, we can process the data in real-time without having to wait for the entire data stream to be received.
Lazy evaluation:
Suppose you have a list of integers that you want to square, but you don’t want to compute the squares until they are actually needed. Here’s how you could use a generator to implement lazy evaluation:
def square_numbers(numbers): """Generator function to lazily compute the square of each number in a list.""" for num in numbers: yield num ** 2 # Compute the squares large_list = list(range(10000000)) squares = square_numbers(large_list) # Print the first 10 squares for i in range(10): print(next(squares))
In this example, we define a generator function square_numbers
that takes a list of numbers as input. The function computes the square of each number in the list lazily using the yield
statement.
By using a generator to compute the squares lazily, we only compute the squares as they are needed, rather than computing all of them upfront. This can be a significant performance improvement when dealing with large data sets or complex computations.
Generator Performance
Generator expressions are a memory-efficient way of creating iterators in Python, which can be very helpful when dealing with large data sets or infinite sequences. In terms of performance, generator expressions can often be faster and more efficient than other methods for creating iterators, such as list comprehensions.
One of the key benefits of using generator expressions is that they only generate the values as they are needed, rather than generating the entire sequence upfront. This means that if you only need to access the first few items of a large sequence, you don’t need to generate the entire sequence in memory, which can be a significant performance boost.
Additionally, because generator expressions are implemented as iterators, they can be used to process large amounts of data without having to load everything into memory at once. This can be especially important for applications that deal with large data sets, as it can significantly reduce memory usage and improve performance.
However, it’s important to note that the performance benefits of using generator expressions can vary depending on the specific use case and implementation. In some cases, other approaches such as list comprehensions or traditional loops may be more efficient. It’s always a good idea to benchmark and test different approaches to determine the best one for your specific use case.
Overall, generator expressions are a powerful tool for creating memory-efficient and high-performance iterators in Python. By using them in the right way, you can often improve the performance of your code and make it more efficient and scalable.
Generator expressions are a powerful and memory-efficient way to create iterators in Python. They allow you to create complex iterators on the fly, without having to create lists in memory. They are especially useful when working with large data sets or infinite sequences. I hope this article has helped you understand generator expressions and how to use them in your Python code.