Python is a dynamically typed language, which means that data types are inferred automatically based on the values assigned to variables. However, it’s still important to understand the different data types available in Python and how to use them effectively.
Here’s an overview of the most common data types in Python:
Numeric Data Type:
- I
nt
: Integers are whole numbers with no decimal places. They can be positive or negative. For example:
x = 42 y = -10 z = 0
float
: Floats are numbers with decimal places. They can also be expressed in scientific notation. For example:
x = 3.14 y = 1.23e-4
complex
: Complex numbers are numbers with a real and imaginary part. They can be expressed using thej
suffix. For example:
x = 3 + 4j y = 1j
Boolean type:
bool
: Booleans represent truth values, which can either beTrue
orFalse
. They are typically used in control structures, such asif
statements and loops, to control the flow of a program. For example:
x = True y = False
Sequence types:
str
: Strings are used for working with text data. They are enclosed in either single quotes, double quotes, or triple quotes. For example:
x = 'Hello, World!' y = "Python is great" z = '''This is a multiline string'''
list
: Lists are used for working with collections of elements. They are enclosed in square brackets and can contain elements of different types. For example:
x = [1, 2, 3, 4] y = ['apple', 'banana', 'cherry'] z = [1, 'apple', True, 3.14]
tuple
: Tuples are similar to lists, but they are immutable, meaning they cannot be modified once created. They are enclosed in parentheses and can contain elements of different types. For example:
x = (1, 2, 3, 4) y = ('apple', 'banana', 'cherry') z = (1, 'apple', True, 3.14)
range
: Ranges are used for generating sequences of numbers. They are created using therange()
function and can take one, two, or three arguments. For example:
x = range(5) # [0, 1, 2, 3, 4] y = range(1, 5) # [1, 2, 3, 4] z = range(1, 10, 2) # [1, 3, 5, 7, 9]
Set types:
set
: Sets are used for performing set operations, such as union, intersection, and difference. They are enclosed in curly braces and contain unique elements. For example:
x = {1, 2, 3} y = {'apple', 'banana', 'cherry'} z = {1, 'apple', True, 3.14}
frozenset
: Frozensets are similar to sets, but they are immutable, meaning they cannot be modified once created. They are created using thefrozenset()
function. For example:
x = frozenset({1, 2, 3}) y = frozenset({'apple',
Mapping Data Type:
dict
: Dictionaries are used for working with key-value pairs. They are enclosed in curly braces and contain key-value pairs separated by colons. For example
x = {'name': 'John', 'age': 30} y = {1: 'apple', 2: 'banana', 3: 'cherry'} z = {'name': 'John', 'age': 30, 'is_male': True}
Binary types:
bytes
: Bytes are used for working with binary data, such as images and audio files. They are immutable and can contain values from 0 to 255. For example:
x = b'hello' y = bytes([72, 101, 108, 108, 111])
bytearray
: Bytearrays are similar to bytes, but they are mutable, meaning they can be modified once created. They are created using thebytearray()
function. For example:
x = bytearray(b'hello') y = bytearray([72, 101, 108, 108, 111])
In terms of efficiency, it depends on the specific use case. For example, if you are working with a large collection of elements and need to modify it frequently, a list may be more efficient than a tuple because it is mutable. On the other hand, if you need to perform set operations on a collection of elements, a set or frozenset may be more efficient than a list or tuple. Ultimately, the choice of data type depends on the specific requirements of your program.
In terms of performance, some data types are faster or more efficient than others depending on the use case. For example, int
and float
are generally faster than str
because they require less memory and fewer operations to perform calculations. Similarly, tuple
is generally faster than list
because it is immutable and can be optimized for certain operations. However, the choice of data type should ultimately depend on the specific requirements of your program and the type of data you are working with.
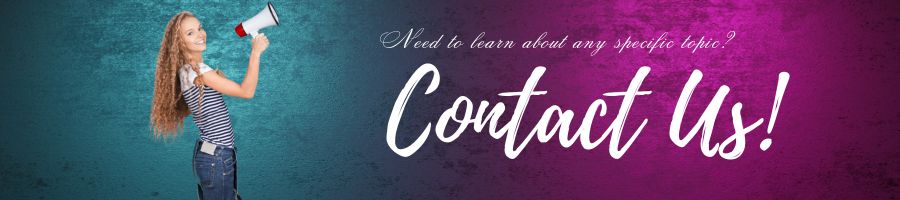
Python Data Type Memory Usage:
Here’s a table showing the approximate memory usage of the most common data types in Python 3: Note that the actual memory usage may vary depending on the implementation and version of Python, as well as the platform and system architecture. This table provides a rough estimate based on CPython 3.9 running on a 64-bit machine.
Data Type | Memory Usage |
---|---|
bool | 28 bytes |
int | 28 bytes + 4 bytes per additional digit |
float | 24 bytes |
complex | 32 bytes |
str | 49 bytes + 1-4 bytes per additional character |
list | 40 bytes + 8 bytes per item |
tuple | 24 bytes + 8 bytes per item |
range | 48 bytes + 24 bytes per digit |
set | 224 bytes + 28 bytes per item |
frozenset | 224 bytes + 28 bytes per item |
dict | 240 bytes + 52 bytes per item |
The codes (if any) mentioned in this post can be downloaded from github.com. Share the post with someone you think can benefit from the information