Python strings methods are powerful and essential string methods in the Python programming language. They are used to store and manipulate textual data, such as names, addresses, and messages. In addition to the basic operations like indexing, slicing, concatenation, and repetition, Python also provides a variety of built-in methods that can be used to perform more advanced operations on strings. In this article, we will explore some of the most common string methods in Python and provide examples of their usage.
String Methods in Python
1. capitalize()
The capitalize()
method returns a copy of the string with its first character capitalized and the rest of the characters in lower case. Here’s an example:
string = "hello, world!" new_string = string.capitalize() print(new_string) # Output: Hello, world!
In this example, the capitalize()
method is called on the string
variable, which converts the first letter to uppercase.
2. lower()
The lower()
method returns a copy of the string with all characters in lower case. Here’s an example:
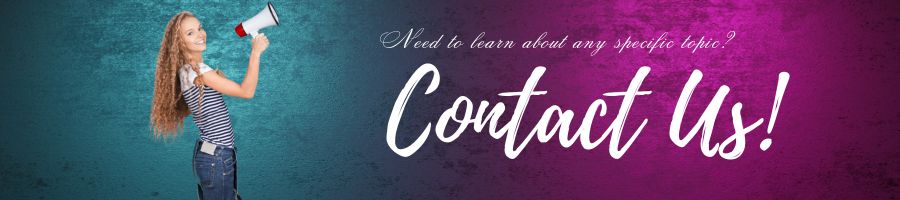
string = "Hello, World!" new_string = string.lower() print(new_string) # Output: hello, world!
In this example, the lower()
method is called on the string
variable, which converts all letters to lower case.
3. upper()
The upper()
method returns a copy of the string with all characters in upper case. Here’s an example:
string = "Hello, World!" new_string = string.upper() print(new_string) # Output: HELLO, WORLD!
In this example, the upper()
method is called on the string
variable, which converts all letters to upper case.
4. swapcase()
The swapcase()
method returns a copy of the string with all uppercase characters converted to lowercase and vice versa. Here’s an example:
string = "Hello, World!" new_string = string.swapcase() print(new_string) # Output: hELLO, wORLD!
In this example, the swapcase()
method is called on the string
variable, which converts all uppercase letters to lowercase and vice versa.
5. title()
The title()
method returns a copy of the string with the first letter of each word capitalized and the rest of the letters in lower case. Here’s an example:
string = "hello, world!" new_string = string.title() print(new_string) # Output: Hello, World!
In this example, the title()
method is called on the string
variable, which capitalizes the first letter of each word.
6. strip()
The strip()
method returns a copy of the string with all leading and trailing whitespace characters removed. Here’s an example:
string = " hello, world! " new_string = string.strip() print(new_string) # Output: hello, world!
In this example, the strip()
method is called on the string
variable, which removes the leading and trailing whitespace characters.
7. replace()
The replace()
method returns a copy of the string with all occurrences of a specified substring replaced with another substring. Here’s an example:
string = "Hello, world!" new_string = string.replace("world", "Python") print(new_string) # Output: Hello, Python!
In this example, the replace()
method is called on the string
variable, which replaces the substring "world"
with "Python"
.
8. split()
The split()
method returns a list of substrings separated by a specified delimiter. Here’s an example:
string = "Hello, world!" new_list = string.split(",") print(new_list) # Output: ['Hello', ' world!']
In this example, the split()
method is called on the string
variable, which splits the string into a list of substrings separated by the comma character.
9. startswith()
The startswith()
method returns True
if the string starts with the specified substring, and False
otherwise. Here’s an example:
string = "Hello, world!" result = string.startswith("Hello") print(result) # Output: True
In this example, the startswith()
method is called on the string
variable, which checks if the string starts with the substring "Hello"
.
10. endswith()
The endswith()
method returns True
if the string ends with the specified substring, and False
otherwise. Here’s an example:
string = "Hello, world!" result = string.endswith("world!") print(result) # Output: True
In this example, the endswith()
method is called on the string
variable, which checks if the string ends with the substring "world!"
.
11. join()
The join()
method joins a sequence of strings with the specified delimiter. Here’s an example:
list = ["Hello", "world"] delimiter = ", " new_string = delimiter.join(list) print(new_string) # Output: "Hello, world"
In this example, the join()
method is called on the delimiter
variable, which joins the elements of the list
variable with the specified delimiter.
12. count()
The count()
method returns the number of occurrences of a specified substring in the string. Here’s an example:
string = "Hello, world!" count = string.count("l") print(count) # Output: 3
In this example, the count()
method is called on the string
variable, which counts the number of occurrences of the substring "l"
.
String Constants
In Python, string constants refer to pre-defined string values that can be used in your code. These constants are typically stored in the string
module of Python and can be imported and used in your code.
The string
module provides a number of pre-defined string constants that can be useful for performing various string operations. For example, the string.ascii_letters
constant contains all ASCII letters (abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ
), while the string.digits
constant contains all decimal digits (0123456789
).
Using these constants can make your code more readable and maintainable, as you can refer to them by name rather than using hardcoded string values in your code. Additionally, the use of string constants can help you avoid errors caused by typos or incorrect values.
List of string constants.
Constant | Description |
---|---|
string.ascii_letters | A string containing all ASCII letters (abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ ). |
string.ascii_lowercase | A string containing all ASCII lowercase letters (abcdefghijklmnopqrstuvwxyz ). |
string.ascii_uppercase | A string containing all ASCII uppercase letters (ABCDEFGHIJKLMNOPQRSTUVWXYZ ). |
string.digits | A string containing all decimal digits (0123456789 ). |
string.hexdigits | A string containing all hexadecimal digits (0123456789abcdefABCDEF ). |
string.octdigits | A string containing all octal digits (01234567 ). |
string.printable | A string containing all printable ASCII characters (i.e., all characters with ASCII codes between 32 and 126, inclusive). |
string.punctuation | A string containing all ASCII punctuation characters (i.e., all characters with ASCII codes between 33 and 47, inclusive, and between 58 and 64, inclusive, and between 91 and 96, inclusive, and between 123 and 126, inclusive). |
You can use these constants in your Python code to perform various string operations, such as filtering out non-printable characters or checking if a string contains only digits.
Conclusion
Python provides a rich set of string methods that make it easy to manipulate and process textual data. In this article, we have covered some of the most commonly used string methods in Python, along with examples of their usage. By mastering these methods, you will be able to work with strings more effectively and write more efficient and powerful Python code.
The codes (if any) mentioned in this post can be downloaded from github.com. Share the post with someone you think can benefit from the information