A heap is a specialized tree-based data structure that satisfies the heap property. Heaps are commonly used to implement priority queues and are crucial in algorithms related to sorting, graph algorithms, and scheduling processes. There are two main types of heaps: Max Heap and Min Heap.
Heap Property:
- Max Heap: In a max heap, for any given node, the value of that node is greater than or equal to the values of its children. The maximum element is at the root.
- Min Heap: In a min heap, for any given node, the value of that node is less than or equal to the values of its children. The minimum element is at the root.
Key Operations:
- Insertion: Adding a new element to the heap.
- Deletion: Removing the root element from the heap.
- Heapify: Reorganizing the elements to maintain the heap property after an operation.
Heap Structure:
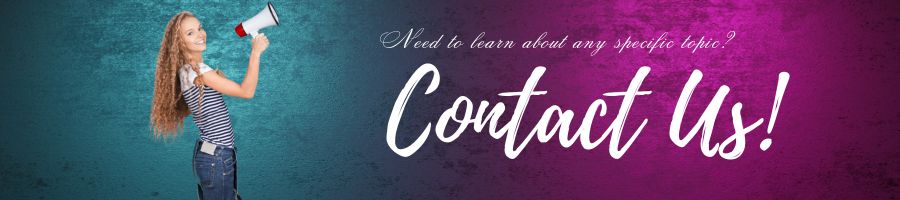
A heap is typically implemented as a binary tree, where each node corresponds to an element in the heap. The binary tree is usually represented as an array, and the elements are stored in a way that allows for easy navigation.
Common Use Cases:
- Priority Queues: Heaps are often used to implement priority queues, where elements with higher priority (or lower value in min heap) are dequeued first.
- Heap Sort: An in-place sorting algorithm that uses a binary heap data structure.
- Dijkstra’s Shortest Path Algorithm: Heaps are used to efficiently select the vertex with the smallest tentative distance in each iteration.
- Huffman Coding: Building a Huffman tree, a special type of heap, for data compression.
Operations on Heaps:
- Insertion:
- Add the new element to the end of the heap (as the last leaf).
- Compare the element with its parent and swap if necessary until the heap property is restored.
- Deletion (Extract Maximum or Extract Minimum):
- Remove the root element.
- Move the last leaf to the root.
- Compare the new root with its children and swap if necessary until the heap property is restored.
- Heapify:
- Starting from the last non-leaf node, compare the node with its children and swap if necessary until the heap property is satisfied.
Time Complexity:
- The time complexity for insertion and deletion in a heap is O(log n), where n is the number of elements in the heap.
Thus we can say, heaps are tree-based data structures that efficiently maintain the maximum or minimum element at the root, making them valuable for various applications such as priority queues and sorting algorithms.