A tree is a hierarchical data structure that consists of nodes connected by edges. Each node in a tree has a parent-child relationship with other nodes, and the topmost node is called the root. The nodes without any children are called leaves. Trees are widely used in computer science for representing hierarchical relationships, organizing data, and implementing various algorithms.
Key Terminology:
- Node: Each element in a tree, which may contain a value or data.
- Edge: The link between two nodes.
- Root: The topmost node in a tree.
- Parent: A node that has one or more child nodes.
- Child: A node that has a parent node.
- Leaf: A node with no children.
- Depth: The level of a node in the tree, with the root having depth 0.
- Height: The length of the longest path from a node to a leaf. The height of the tree is the height of the root.
Types of Trees:
- Binary Tree: Each node has, at most, two children – a left child and a right child.
- Binary Search Tree (BST): A binary tree with the property that the left subtree of a node contains only nodes with values less than the node’s value, and the right subtree contains only nodes with values greater than the node’s value.
- AVL Tree: A self-balancing binary search tree, where the height difference between the left and right subtrees of any node is at most one.
- B-Tree: A tree structure optimized for disk storage and database systems.
- Trie: A tree-like data structure used for storing a dynamic set or associative array.
Common Operations on Trees:
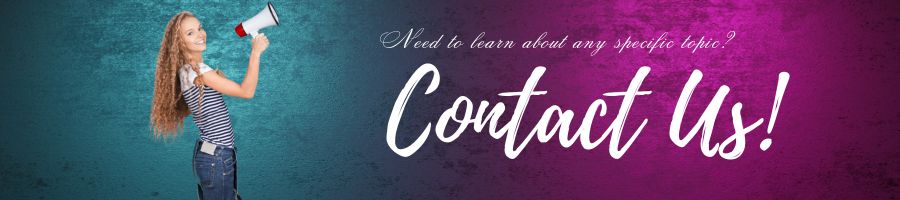
- Insertion: Adding a new node to the tree.
- Deletion: Removing a node from the tree.
- Traversal: Visiting all nodes in a specific order.
- In-order: Left, Root, Right
- Pre-order: Root, Left, Right
- Post-order: Left, Right, Root
- Search: Finding a specific node in the tree.
- Balancing (for self-balancing trees): Maintaining the balance property.
Use Cases:
- File Systems: Representing the hierarchical structure of files and directories.
- Expression Trees: Representing mathematical expressions.
- Huffman Coding Trees: Used in data compression algorithms.
- Abstract Syntax Trees (AST): Representing the syntactic structure of source code in compilers.
Thus we can say, trees are versatile data structures used for organizing and representing hierarchical relationships. They provide efficient solutions for various problems, and different types of trees serve specific purposes in different applications.