A linked list is a linear data structure in which elements are stored in nodes, and each node points to the next node in the sequence. Unlike arrays, linked lists do not require contiguous memory locations, and their size can be dynamically changed during runtime. Each node in a linked list contains two fields: a data field to store the element and a reference (or link) field pointing to the next node in the sequence.
Characteristics of Linked Lists:
- Dynamic Size: Linked lists can easily grow or shrink in size during runtime, as memory is allocated or deallocated as needed.
- Non-contiguous Memory Allocation: Nodes in a linked list can be scattered in memory; they are connected through references rather than being stored in contiguous memory locations.
- No Fixed Size: Unlike arrays, linked lists do not have a fixed size, and you can keep adding or removing elements without worrying about pre-allocating a specific amount of memory.
Types of Linked Lists:
- Singly Linked List: Each node points to the next node in the sequence.
- Doubly Linked List: Each node has two references, one pointing to the next node and another pointing to the previous node.
- Circular Linked List: The last node in the list points back to the first node, creating a circular structure.
Advantages of Linked Lists:
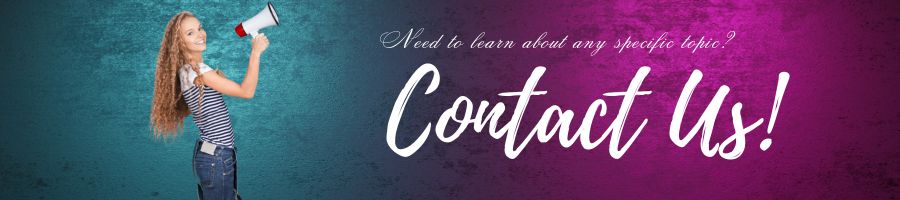
- Dynamic Size: Linked lists can grow or shrink easily during runtime.
- Efficient Insertions and Deletions: Adding or removing elements in a linked list is more efficient than in an array, especially for large datasets.
Disadvantages of Linked Lists:
- Random Access is Inefficient: Unlike arrays, you cannot directly access an element in constant time using an index. You have to traverse the list from the beginning.
- Extra Memory Overhead: Each node in a linked list requires additional memory for the reference field, which can result in higher memory overhead compared to arrays.
Common Operations:
- Insertion: Adding a new node to the list.
- Deletion: Removing a node from the list.
- Traversal: Iterating through the list.
- Searching: Finding a specific element in the list.
Use Cases:
Linked lists are often used when the size of the data structure is not known in advance, or when frequent insertions and deletions are expected.
Thus we can say, linked lists are a flexible and dynamic data structure that provides efficient insertion and deletion operations at the cost of random access efficiency. The choice between an array and a linked list depends on the specific requirements of the application.