A data structure is a way of organizing and storing data in a computer so that it can be accessed and used efficiently. Data structures are important for managing and organizing information in a way that enables efficient retrieval and modification.
Data structures categories:
- Primitive Data Structures: These are the basic building blocks and include simple data types like integers, floats, characters, and pointers.
- Composite Data Structures: These structures are composed of primitive data types and provide a way to organize and manage data collections. Examples include arrays, linked lists, stacks, queues, trees, graphs, and hash tables.
Why Use Data Structures?
The use of data structures is fundamental in software development for several reasons:
- Efficient Data Retrieval: Different data structures are designed for different purposes. Choosing the right data structure allows for efficient retrieval of data, which is critical for the performance of algorithms and applications.
- Optimized Operations: Data structures define specific operations that can be performed on the stored data. Well-designed data structures enable optimized algorithms for common operations like searching, sorting, insertion, and deletion.
- Memory Utilization: Effective use of data structures helps in optimizing memory utilization. By selecting the appropriate structure, you can reduce memory overhead and improve the overall efficiency of your program.
- Scalability: As the data size grows, operations’ efficiency becomes crucial. Properly chosen data structures contribute to the scalability of the software, ensuring that it can handle larger datasets without significant performance degradation.
- Organizing Data: Data structures provide a way to organize data logically. For instance, trees and graphs are useful for representing hierarchical relationships, while arrays and lists are suitable for linear sequences.
- Ease of Maintenance: Well-organized data structures lead to more maintainable code. When the underlying data is structured efficiently, it becomes easier to understand and modify the code, reducing the likelihood of errors.
- Algorithm Design: Many algorithms are intricately linked to the choice of data structures. A thoughtful selection of data structures simplifies algorithm design and implementation.
In summary, the use of data structures is essential for efficient data management, algorithm design, and overall optimization of software. Let’s deep dive into various data strutures. The document covers fundamental data structures, advanced structures, and their use in real-world scenarios.
Data Structures Types
Let’s explain all the major data structures available
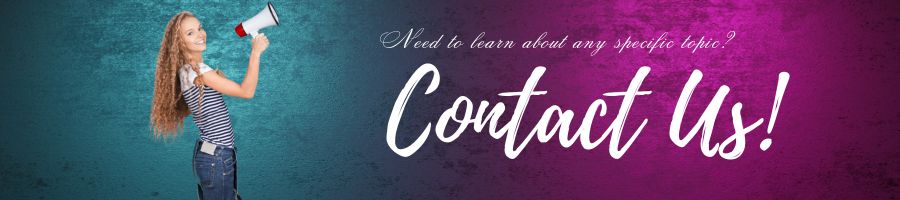
Arrays
An array is a fundamental data structure that stores elements of the same data type in contiguous memory locations. Each element in an array is identified by its index or key.
Linked List
A linked list is a linear data structure in which elements are stored in nodes, and each node points to the next node in the sequence. Unlike arrays, linked lists do not require contiguous memory locations, and their size can be dynamically changed during runtime.
Stack
A stack is a linear data structure that follows the Last-In-First-Out (LIFO) principle. In a stack, elements are added and removed from the same end, often referred to as the “top” of the stack. The last element added is the first one to be removed.
Queue
A queue is a linear data structure that follows the First-In-First-Out (FIFO) principle. In a queue, elements are added at the rear (enqueue) and removed from the front (dequeue). The first element added is the first one to be removed.
Hash Tables
A hash table, also known as a hash map, is a data structure that implements an associative array abstract data type, a structure that can map keys to values. It uses a hash function to compute an index into an array of buckets or slots, from which the desired value can be found.
Trees
A tree is a hierarchical data structure that consists of nodes connected by edges. Each node in a tree has a parent-child relationship with other nodes, and the topmost node is called the root. The nodes without any children are called leaves.
Heaps
A heap is a specialized tree-based data structure that satisfies the heap property. Heaps are commonly used to implement priority queues and are crucial in algorithms related to sorting, graph algorithms, and scheduling processes.
Tries
A trie (pronounced “try”) is a tree-like data structure that is used to store a dynamic set or associative array where the keys are usually strings. The term “trie” comes from the word “retrieval” because of its suitability for retrieval tasks.
Graphs
A graph is a mathematical and abstract representation of a set of objects where some pairs of objects are connected by links. The objects, often called vertices or nodes, can represent various entities, and the links, called edges, represent relationships or connections between the entities.
The above data structures give an overall idea of data structures. Each section are expanded with detailed explanations, code snippets, and additional examples to provide a thorough understanding of each topic in linked articles.