When it comes to Python, decorators often feel like one of those advanced, mystical topics that many beginners shy away from. However, once you understand the concept and learn how to wield their power, decorators can transform your code, making it more elegant, efficient, and reusable.
In this guide, we’ll break down Python decorators, starting from the basics and building up to more advanced concepts. By the end, you’ll not only understand decorators but also feel confident using and even creating your own.
What Are Python Decorators?
Let’s start with the basics. A decorator is essentially a function that takes another function (or method) as input, adds some functionality to it, and then returns it. Decorators are a great way to modify or extend the behavior of functions or methods without changing their actual code.
Think of decorators as wrappers. Imagine you have a plain cupcake (a function), and you want to add frosting and sprinkles to make it more delightful. A decorator is the process of applying that frosting and sprinkles without changing the cupcake itself.
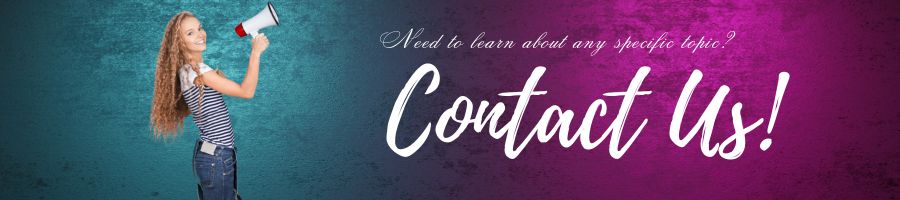
Here’s a simple example to get a taste of decorators:
# A simple decorator def my_decorator(func): def wrapper(): print("Something is happening before the function is called.") func() print("Something is happening after the function is called.") return wrapper @my_decorator def say_hello(): print("Hello, World!") say_hello()
What’s Happening Here?
- We define
my_decorator
, which takes a function (func
) as its argument. - Inside
my_decorator
, we define a nested function calledwrapper
that adds some extra behavior before and after callingfunc
. - The
@my_decorator
syntax applies the decorator to thesay_hello
function. - When we call
say_hello()
, the wrapper function executes, adding extra behavior around the originalsay_hello
function.
Output:
Something is happening before the function is called. Hello, World! Something is happening after the function is called.
Why Use Decorators?
Decorators are useful when you need to:
- Add functionality to existing code without modifying it.
- Apply the same logic to multiple functions (e.g., logging, timing, authentication).
- Write cleaner and more DRY (Don’t Repeat Yourself) code.
For example, decorators are widely used in web frameworks like Flask and Django to handle routing, authentication, and more.
Digging Deeper: Understanding the @ Syntax
The @
symbol is just syntactic sugar. When you write:
@my_decorator def my_function(): pass
It is equivalent to:
def my_function(): pass my_function = my_decorator(my_function)
This is why decorators must return a callable (usually the wrapper function).
Decorator Examples
Let’s look at some common use cases for decorators:
1. Logging
def log_decorator(func): def wrapper(*args, **kwargs): print(f"Function {func.__name__} is called with arguments: {args} {kwargs}") result = func(*args, **kwargs) print(f"Function {func.__name__} returned: {result}") return result return wrapper @log_decorator def add(a, b): return a + b add(3, 5)
Output:
Function add is called with arguments: (3, 5) {} Function add returned: 8
2. Timing Functions
import time def timer_decorator(func): def wrapper(*args, **kwargs): start_time = time.time() result = func(*args, **kwargs) end_time = time.time() print(f"Function {func.__name__} executed in {end_time - start_time:.4f} seconds") return result return wrapper @timer_decorator def slow_function(): time.sleep(2) print("Finished!") slow_function()
Output:
Finished! Function slow_function executed in 2.0001 seconds
3. Authentication
def requires_login(func): def wrapper(user): if not user.get("is_logged_in"): print("User is not logged in!") return return func(user) return wrapper @requires_login def show_dashboard(user): print(f"Welcome to your dashboard, {user['username']}!") user = {"username": "Alice", "is_logged_in": True} show_dashboard(user)
Output:
Welcome to your dashboard, Alice!
Creating Parameterized Decorators
Sometimes, you need decorators that accept arguments. This requires an extra layer of wrapping.
def repeat(times): def decorator(func): def wrapper(*args, **kwargs): for _ in range(times): func(*args, **kwargs) return wrapper return decorator @repeat(3) def greet(): print("Hello!") greet()
Output:
Hello! Hello! Hello!
Advanced: Class-Based Decorators
Decorators can also be implemented as classes by defining a __call__
method, which makes the instance callable.
class MyDecorator: def __init__(self, func): self.func = func def __call__(self, *args, **kwargs): print("Before the function call") result = self.func(*args, **kwargs) print("After the function call") return result @MyDecorator def say_hello(): print("Hello, World!") say_hello()
Output:
Before the function call Hello, World! After the function call
Decorator Stacking
You can stack multiple decorators on a single function. They are applied from the innermost to the outermost.
def uppercase(func): def wrapper(*args, **kwargs): result = func(*args, **kwargs) return result.upper() return wrapper def exclaim(func): def wrapper(*args, **kwargs): result = func(*args, **kwargs) return f"{result}!" return wrapper @uppercase @exclaim def greet(): return "hello" greet()
Output:
HELLO!
Best Practices for Using Decorators
- Use Built-in Decorators When Possible: Python provides some built-in decorators like
@staticmethod
,@classmethod
, and@property
. - Name Your Wrappers: Use
functools.wraps
to preserve the original function’s metadata (e.g., name and docstring).
from functools import wraps def my_decorator(func): @wraps(func) def wrapper(*args, **kwargs): # Add behavior here return func(*args, **kwargs) return wrapper
- Keep It Simple: Avoid overcomplicating your decorators. They should be easy to understand and maintain.
Conclusion
Decorators are a powerful feature in Python, enabling you to write cleaner, more reusable, and more expressive code. Whether you’re logging, timing, or enforcing authentication, decorators help you apply common patterns elegantly. Start simple, experiment with your own decorators, and soon you’ll be wielding their magic like a pro.