The pathlib
module in Python provides an object-oriented approach to handle file system paths and operations. It offers a more intuitive and convenient way to work with paths compared to traditional string manipulation techniques.
To begin, you need to import the pathlib
module in your Python script:
from pathlib import Path
Now, let’s explore the key features and functionality of the pathlib
module:
Creating a Path object
The first step is to create a Path
object that represents a file or directory path. You can create a Path
object by passing a string representing the path:
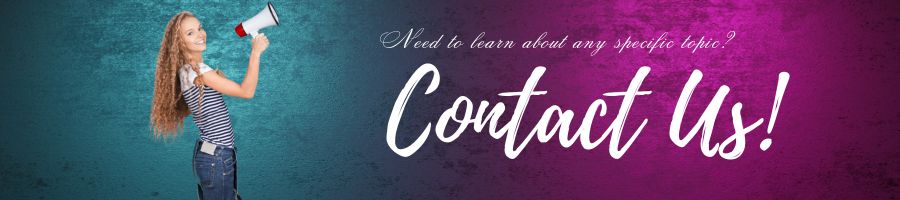
path = Path("/path/to/file.txt")
Alternatively, you can use the Path
constructor with a relative path:
path = Path("relative/path/to/file.txt")
Getting information about a path
Once you have a Path
object, you can extract various information about the path:
# Check if the path exists print(path.exists()) # Check if the path is a file print(path.is_file()) # Check if the path is a directory print(path.is_dir()) # Get the file name print(path.name) # Get the parent directory print(path.parent) # Get the absolute path print(path.absolute()) # Get the file size in bytes print(path.stat().st_size)
Navigating the file system
The pathlib
module provides convenient methods for navigating the file system:
# Join two paths new_path = path / "new_folder" / "new_file.txt" print(new_path) # Resolve the path (resolve symbolic links and remove redundant elements) resolved_path = path.resolve() print(resolved_path) # Get the relative path to another path relative_path = path.relative_to("/path/to") print(relative_path) # Get the list of files and directories in a directory for item in path.iterdir(): print(item)
Creating, copying, and deleting files/directories
The pathlib
module allows you to create, copy, and delete files and directories:
# Create a new directory path.mkdir() # Create a new file path.touch() # Copy a file or directory new_path = path.copy_to("/path/to/destination") # Rename a file or directory new_path = path.rename("/path/to/new_name") # Delete a file or directory path.unlink()
Reading and writing files
The pathlib
module provides methods for reading and writing file contents:
# Read the contents of a file content = path.read_text() print(content) # Write to a file path.write_text("Hello, World!") # Append to a file path.write_text("More content", append=True)
Tips and tricks
Here are some useful tips and tricks when working with the pathlib
module:
- You can use the
/
operator to join paths instead of manually concatenating strings. This helps to handle platform-specific path separators correctly. - If you have a string path, you can convert it to a
Path
object usingPath("your/path")
. - The
glob()
method allows you to search for files using wildcards. For example,path.glob("*.txt")
will find all text files in the given path. - The
with_suffix()
method lets you change the file extension of aPath
object. - If you want to iterate through all files in a directory recursively, you can use the
rglob()
method
- If you want to iterate through all files in a directory recursively, you can use the
rglob()
method. It works similar toglob()
, but it also searches for files in subdirectories:
for file_path in path.rglob("*"): print(file_path)
- You can check if a path is an absolute path by using the
is_absolute()
method. If the path is relative, you can make it absolute by using theresolve()
method. - The
Path
object provides methods likesuffix
andsuffixes
to extract the file extension(s) from a path. For example:
file_path = Path("/path/to/file.txt") print(file_path.suffix) # Output: ".txt" print(file_path.suffixes) # Output: [".txt"]
- The
iterdir()
method returns a generator that yields all the files and directories in the current directory. You can combine it with theis_file()
oris_dir()
methods to filter the results based on file type. - The
open()
method inPath
can be used to open a file for reading or writing. It returns a file object that you can use to perform read or write operations. For example:
file_path = Path("/path/to/file.txt") # Open the file for reading with file_path.open() as file: content = file.read() print(content) # Open the file for writing with file_path.open(mode="w") as file: file.write("Hello, World!")
- If you need to check permissions or ownership of a file, you can use the
stat()
method to get the file’s status information. It returns an object with various attributes related to the file.
file_path = Path("/path/to/file.txt") file_stats = file_path.stat() # Check permissions print(file_stats.st_mode) # Check owner user id print(file_stats.st_uid) # Check owner group id print(file_stats.st_gid) # Check modification time print(file_stats.st_mtime)
These are some key features and tips for working with the pathlib
module in Python. It offers a powerful and intuitive way to handle file system operations, making it easier to work with paths and files in your Python programs.
Here are a few more tips and tricks for working with the pathlib
module:
- The
pathlib
module provides methods for checking various properties of a file or directory. For example:
path.is_absolute()
checks if the path is an absolute path.path.is_relative_to(other_path)
checks if the path is relative to another path.path.is_symlink()
checks if the path is a symbolic link.path.is_socket()
checks if the path is a socket.path.is_fifo()
checks if the path is a FIFO (named pipe).
- The
resolve()
method not only resolves symbolic links and removes redundant elements but also returns an absolute path. It can be useful in cases where you want to work with the canonical absolute path. - You can use the
chmod()
method to change the permissions (mode) of a file or directory. For example:
path.chmod(0o755) # Sets permissions to read, write, and execute for the owner, and read and execute for others
- The
name
property returns the name of the file or directory, including the extension. If you want to extract only the file name without the extension, you can use thestem
property. For example:
file_path = Path("/path/to/file.txt") print(file_path.name) # Output: "file.txt" print(file_path.stem) # Output: "file"
- The
as_posix()
method returns the path as a string using the POSIX path separator (“/”) regardless of the platform. This can be useful when you need to work with paths that are compatible with POSIX systems. - The
with_name()
andwith_suffix()
methods allow you to change the name and extension of aPath
object while keeping the rest of the path intact. For example:
file_path = Path("/path/to/file.txt") new_path = file_path.with_name("new_file.txt") print(new_path) # Output: "/path/to/new_file.txt" new_path = file_path.with_suffix(".csv") print(new_path) # Output: "/path/to/file.csv"
- If you need to create temporary files or directories, the
pathlib
module provides theTemporaryFile()
andTemporaryDirectory()
functions as convenient alternatives to thetempfile
module. These functions returnPath
objects representing the temporary file or directory.
These additional tips and tricks should further enhance your understanding and usage of the pathlib
module in Python