In Python, arrays are a fundamental data structure used to store homogeneous data. Arrays are similar to lists, but they are more efficient when working with large amounts of data. This is because arrays use less memory and provide faster access to elements.
In this article, we will explore Python arrays and their operations in detail. We will also discuss the time and space complexity of each operation.
Creating Arrays
To create an array in Python, we need to import the array module. The array module provides an array() function that takes two arguments: the type code and the sequence of values.
The type code is a character that represents the data type of the array elements. For example, ‘i’ represents integer, ‘f’ represents float, and ‘d’ represents double. The sequence of values is a list or tuple that contains the initial values of the array. Here is an example of creating an array of integers:
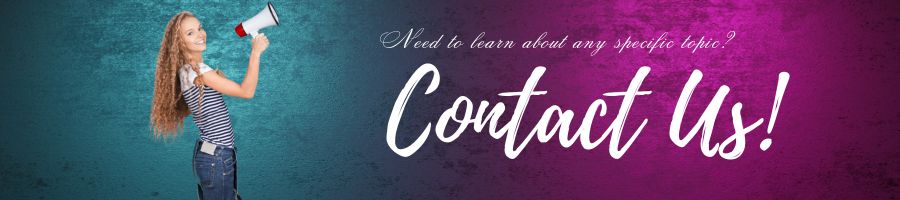
import array my_array = array.array('i', [1, 2, 3, 4, 5])
This creates an array of integers called ‘my_array’ that contains the values 1, 2, 3, 4, and 5.
Type Codes
Here are some of the important type codes in Python arrays:
Type Code | Python Type | Description |
---|---|---|
‘b’ | int | Signed integer |
‘B’ | int | Unsigned integer |
‘i’ | int | Signed integer |
‘I’ | int | Unsigned integer |
‘f’ | float | Floating-point |
‘d’ | float | Double precision |
These type codes allow you to work with a range of data types, including integers and floating-point numbers, in a more memory-efficient way than using regular Python lists. They are often used in scientific and numerical computing applications.
Accessing Elements
To access elements in an array, we can use the indexing operator []. The index starts at 0 for the first element and increases by 1 for each subsequent element. Here is an example of accessing elements in an array:
import array my_array = array.array('i', [1, 2, 3, 4, 5]) print(my_array[0]) # Output: 1 print(my_array[2]) # Output: 3
Modifying Elements
To modify elements in an array, we can use the indexing operator [] and assignment operator =. Here is an example of modifying elements in an array:
import array my_array = array.array('i', [1, 2, 3, 4, 5]) my_array[2] = 10 print(my_array) # Output: array('i', [1, 2, 10, 4, 5])
Adding Elements
To add elements to an array, we can use the append() method. The append() method adds the element to the end of the array. Here is an example of adding elements to an array:
import array my_array = array.array('i', [1, 2, 3, 4, 5]) my_array.append(6) print(my_array) # Output: array('i', [1, 2, 3, 4, 5, 6])
Removing Elements
To remove elements from an array, we can use the pop() method. The pop() method removes the element at the specified index and returns its value. Here is an example of removing elements from an array:
import array my_array = array.array('i', [1, 2, 3, 4, 5]) removed_element = my_array.pop(2) print(my_array) # Output: array('i', [1, 2, 4, 5]) print(removed_element) # Output: 3
Searching for an Element
To search for an element in an array, we can use the index() method. The index() method returns the index of the first occurrence of the specified element. Here is an example of searching for an element in an array:
import array my_array = array.array('i', [1, 2, 3, 4, 5]) index = my_array.index(3) print(index) # Output: 2
Time and Space Complexity
In the table below, we summarize the time and space complexity of the array operations discussed in this article:
Operation | Time Complexity | Space Complexity |
---|---|---|
Access | O(1) | O(1) |
Modify | O(1) | O(1) |
Add | O(1) | O(1) |
Remove | O(n) | O(1) |
Search | O(n) | O(1) |
Conclusion
Python arrays are a powerful tool for working with homogeneous data. They are efficient and easy to use. In this article, we covered the basics of creating arrays, accessing and modifying elements, adding and removing elements, and searching for an element. We also discussed the time and space complexity of each operation. Hopefully, this article has provided a good introduction to Python arrays and their operations.