We will start learning the design and analysis of algorithms. Understanding algorithms is like learning the fundamental language of problem-solving in computer science, programming, or data science. They form the core of how we design efficient and intelligent solutions. By mastering algorithms, you can write faster, smarter, and more optimized code, making your programs functional, scalable, and resource-efficient. We will start with definitions in this article and slowly progress.
Having algorithmic knowledge is a cornerstone for technical interviews, a common step in job recruitment for tech-related positions. Companies seek candidates who can devise efficient algorithms to solve real-world problems, showcasing both analytical and programming skills. By mastering algorithms, you become a sought-after candidate and gain the confidence to tackle a diverse range of computational challenges.
What is an Algorithm?
An algorithm is a step-by-step set of well-defined, unambiguous instructions to perform a specific task or solve a particular problem.
Why Use Algorithms?
Algorithms are crucial for problem-solving and efficient computation. They provide a systematic way to solve problems, enabling the development of efficient and scalable solutions.
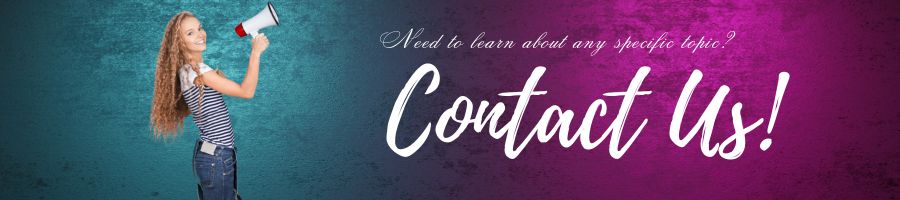
Where are Algorithms Used?
I would say Algorithms are used everywhere, every day, every moment in our life. Technically its used in various fields, including:
- Computer Science: Sorting, searching, and pathfinding algorithms.
- Mathematics: Algorithms for solving equations, finding roots, etc.
- Data Science: Machine learning algorithms, data processing algorithms.
- Cryptography: Algorithms for encrypting and decrypting data.
- Networking: Routing algorithms, data transmission algorithms.
- Artificial Intelligence: Search algorithms, optimization algorithms.
Characteristics/Properties of Algorithms:
The characteristics of an algorithm refer to specific attributes and properties that define its nature and behavior. These characteristics can help you write better algorithms. Below are the key characteristics and their significance.
- Finiteness: An algorithm must have a finite number of steps, ensuring that it eventually terminates. This characteristic guarantees that the algorithm doesn’t run indefinitely, thus making it practical and usable in real-world scenarios
- Input: Algorithms take zero or more inputs, representing the data on which they operate. The input is crucial as it allows the algorithm to adapt to different scenarios, making it versatile and applicable to a variety of problems.
- Definiteness: Each step in the algorithm must be precisely and unambiguously defined. This characteristic eliminates confusion and ensures that the algorithm’s execution is clear and understandable, facilitating implementation and review.
- Output: Every algorithm produces at least one output. This output represents the result of the computation or the solution to the problem. The clear definition of output ensures that the algorithm provides meaningful results, meeting its intended purpose.
- Effectiveness: Every step in the algorithm must be executable, either by a person or a machine. This characteristic ensures that the algorithm is practical and feasible, allowing for its implementation in software or hardware. It also contributes to the algorithm’s reliability and usefulness in real-world applications..
Types of Algorithms:
There are different types of algorithms which can can study but for the shake of this course we will focus on few specific types of algorithms. Understanding these algorithms is essential for developing efficient and effective solutions in programming and computer science and will eventually help you in your job interviews.
Sorting Algorithms:
Sorting algorithms arrange elements in a specific order, such as ascending or descending.
- Examples:
- Bubble Sort: Repeated steps through the list, compares adjacent elements, and swaps them if they are in the wrong order.
- Merge Sort: Divides the array into two halves, recursively sorts them, and then merges them.
Searching Algorithms:
Searching algorithms locate the position of a particular item within a collection of items.
- Examples:
- Binary Search: Divides the sorted array into halves, narrowing down the search space until the element is found.
Graph Algorithms:
Graph algorithms solve problems related to graphs, which consist of nodes and edges.
- Examples:
- Dijkstra’s Algorithm: Finds the shortest path between nodes in a graph.
- Depth-First Search (DFS): Explores as far as possible along each branch before backtracking.
How to Design an Algorithm?
Designing an algorithm is like creating a recipe for solving a specific problem. Let’s break down the process into simple steps that anyone can understand:
1. Understand the Problem:
- Imagine a Cooking Scenario:
- You want to make a sandwich, but you need to understand what ingredients you have and what kind of sandwich you want.
- Algorithm Design:
- Before creating an algorithm, understand the problem you’re trying to solve. What input do you have? What output are you looking for? Clearly define the goal.
2. Devise a Plan:
- Imagine a Cooking Plan:
- You decide to start by gathering ingredients, then assembling the sandwich step by step.
- Algorithm Design:
- Outline a plan or strategy for solving the problem. Think about the high-level steps you need to take to achieve the desired output.
3. Break it Down:
- Imagine a Cooking Breakdown:
- Breaking down involves preparing individual ingredients before putting them together.
- Algorithm Design:
- Divide the problem into smaller, more manageable subproblems. This helps make the overall problem less intimidating.
4. Solve Subproblems:
- Imagine Cooking Subproblems:
- You start by chopping vegetables, cooking meat, and preparing sauces—solving each part individually.
- Algorithm Design:
- Solve each subproblem independently. This could involve writing specific steps for each smaller task that contributes to the overall solution.
5. Combine Solutions:
- Imagine Assembling a Dish:
- Once each subproblem is solved, you combine the prepared ingredients to create the final dish.
- Algorithm Design:
- Integrate the solutions of the subproblems to solve the original problem. Ensure that the combination makes sense and achieves the overall goal.
6. Optimize:
- Imagine Tasting and Adjusting:
- Taste the dish, and if needed, adjust the seasoning or add more ingredients to make it perfect.
- Algorithm Design:
- Refine and optimize your algorithm. Consider if there are more efficient ways to solve the problem. Optimize for speed, efficiency, or simplicity.
7. Express Clearly:
- Imagine Sharing a Recipe:
- If someone else wants to make the same dish, you provide a clear recipe with easy-to-follow steps.
- Algorithm Design:
- Express your algorithm clearly. Use a combination of natural language and simple instructions so that someone else (or a computer) can follow the steps easily.
8. Test Your Algorithm:
- Imagine Checking if the Dish is Delicious:
- Before serving to others, you taste the dish to ensure it meets your expectations.
- Algorithm Design:
- Test your algorithm with different inputs to make sure it produces the correct and expected outputs.
Designing an algorithm is about breaking down a problem into manageable steps, solving each part independently, and then combining the solutions to achieve the desired result. It’s like creating a step-by-step guide for solving a problem, just as you would when cooking a delicious dish.
How to Express Algorithms?
Expressing an algorithm involves conveying step-by-step instructions for solving a problem using a clear representation. There are various ways to express algorithms, and two common methods are pseudocode and flowcharts:
1. Pseudocode:
Pseudocode is a mixture of natural language and simple programming constructs to describe the logic of an algorithm without being tied to a specific programming language. It’s a way to outline the steps in a manner that’s easy to understand.
Example: Finding the Maximum Number in a List
Algorithm: FindMaxNumber
Input: A list of numbers (List)
Output: The maximum number in the list (MaxNumber)
1. Set MaxNumber to the first number in the list.
2. For each number in the list from the second one onwards:
a. If the current number is greater than MaxNumber:
i. Update MaxNumber with the current number.
3. Return MaxNumber.
In this pseudocode, we outline the steps to find the maximum number in a list. It’s clear, concise, and independent of any specific programming language.
2. Flowcharts:
Flowcharts use shapes and arrows to represent the flow of control in an algorithm. Each shape denotes a specific action or decision, and arrows connect these shapes to illustrate the flow.
Example: Checking if a Number is Even or Odd
In this flowchart:
- The oval represents the start or end of the algorithm.
- The rectangle represents a process or action (checking if the number is divisible by 2).
- The diamond represents a decision point (whether the number is divisible by 2).
- The arrows indicate the flow of control.
3. Programming Language:
Expressing an algorithm in an actual programming language involves writing code that implements the steps outlined in the algorithm. This is more specific and closer to what a computer can execute.
Example: Finding the Maximum Number in Pytho def find_max_number(numbers): max_number = numbers[0] # Set the initial max to the first number for number in numbers[1:]: # Iterate through the rest of the numbers if number > max_number: max_number = number # Update max if a larger number is found return max_number
In this Python example, we’ve translated the pseudocode into actual code. This can be directly executed by a computer.
Expressing algorithms through pseudocode, flowcharts, or programming languages provides a structured and communicative way to convey the logic of a solution. The choice of representation depends on the audience — pseudocode for clarity, flowcharts for visualization, and programming languages for implementation.
How to Analyze Algorithms?
- Time Complexity: Measure the amount of time an algorithm takes to complete.
- Space Complexity: Assess the amount of memory an algorithm uses.
- Big-O Notation: Express the upper bound of an algorithm’s growth rate.
- Best, Average, and Worst Cases: Analyze performance under different scenarios.
- Amortized Analysis: Evaluate the average performance over a sequence of operations.
We will go in detail separately as we progress. This comprehensive understanding equips beginners to improve their problem-solving skills and explore algorithms and their applications. Happy learning and feel free to comment and ask any queries.