You want to master FastAPI & Rest API’s? Good Decision. Nowadays, the exchange of information between different software applications is crucial for seamless functionality and efficient operations. This exchange is facilitated by Application Programming Interfaces (APIs). In this article, we’ll delve into what APIs are, why they are necessary, and explore the concepts of REST architecture and FastAPI. Additionally, we’ll guide you through creating your first FastAPI API. This will be the first article in series for Mastering FastAPI.
What is an API?
An API, or Application Programming Interface, is a set of rules, protocols, and tools that allows different software applications to communicate and interact with each other. It defines the methods and data formats that applications can use to request and exchange information. Think of it as a waiter in a restaurant; you, the client (or consumer), place an order (request) to the waiter (API), who then communicates with the kitchen (server) to fulfill your order and serves you the requested dish (response).
Example: Consider a weather application on your smartphone. This app might use an API provided by a weather service to fetch current weather data for your location. The API specifies how the weather app can request this data and what format the response will be in.
Why do you need an API?
APIs are essential for various reasons:
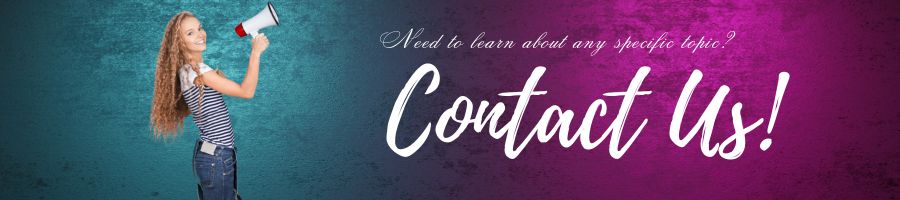
- Integration: APIs allow different software systems to integrate and work together seamlessly. For example, an e-commerce website might use APIs to connect with payment gateways, shipping services, and inventory management systems.
- Efficiency: Instead of building functionalities from scratch, developers can leverage existing APIs to add features to their applications quickly. This saves time and resources.
- Scalability: APIs enable scalability by allowing applications to access resources and services as needed, without needing to manage the underlying infrastructure.
- Standardization: APIs provide a standardized way for applications to communicate, which promotes interoperability and consistency across different systems.
Example: Imagine a travel booking website that needs to display flight options to users. Instead of manually contacting each airline, the website can use flight booking APIs provided by various airlines to fetch real-time flight information and display it to users.
What is REST?
REST, or Representational State Transfer, is a set of architectural constraints. When your API follows these constraints, then you can say it is a REST API. If you don’t follow these constraints, your API may still be a useful API, it may be exactly the thing you need, but it isn’t a REST API. It is based on a set of principles that define how resources are represented and addressed over the web. RESTful APIs adhere to these principles, making them easy to understand, scalable, and maintainable.
Key principles/constraints of REST:
- Client-Server Architecture: REST separates the client and server, allowing them to evolve independently. The client initiates requests, and the server processes them and sends back responses.
- Statelessness: Each request from the client to the server must contain all the information necessary to understand and process the request. The server does not store any client state between requests.
- Uniform Interface: RESTful APIs have a uniform interface, which simplifies communication between clients and servers. This interface typically includes standard HTTP methods (GET, POST, PUT, DELETE) and resource URIs. Any single resource should not be too large and contain each and everything in its representation. Whenever relevant, a resource should contain links (HATEOAS) pointing to relative URIs to fetch related information.
- Cacheability: Responses from the server can be cached to improve performance. Clients can specify whether a response is cacheable or not. Caching can be implemented on the server or client side.
- Layered System: The architecture is composed of multiple layers, where each layer has a specific role and interacts only with adjacent layers. This promotes scalability and flexibility. When you deploy the APIs on server A, and store data on server B and authenticate requests in Server C, for example. A client cannot ordinarily tell whether it is connected directly to the end server or an intermediary along the way.
Example: Suppose you have a RESTful API for managing a library. To retrieve information about a book, you would send a GET request to a specific URI, such as /books/{book_id}
. The server would then respond with the details of the requested book in a standardized format, such as JSON or XML.
What is FastAPI?
FastAPI is a modern, fast , web framework for building APIs with Python. It is built on top of standard Python type hints, making it highly intuitive and easy to use. FastAPI leverages asynchronous programming to achieve high performance and is designed to be easy to learn and use.
Key features of FastAPI:
- Automatic API Documentation: FastAPI automatically generates interactive API documentation based on your code and type hints. This documentation is accessible via a web browser and provides a convenient way for developers to understand and test the API endpoints.
- Validation and Serialization: FastAPI performs automatic data validation and serialization based on the type hints provided in your code. This helps ensure that input data is validated before being processed by your API endpoints, reducing the likelihood of errors.
- Asynchronous Support: FastAPI fully supports asynchronous programming, allowing you to write asynchronous code using Python’s
async
andawait
keywords. This enables you to build highly scalable and efficient APIs that can handle a large number of concurrent requests. - Dependency Injection: FastAPI supports dependency injection, allowing you to easily inject dependencies into your API endpoints. This promotes code reuse and makes it easier to write modular and testable code.
Example: Let’s say you want to build a simple API for a to-do list application using FastAPI. You can define a data model for a todo item using Python classes and then create API endpoints for creating, updating, and deleting todo items. FastAPI will automatically handle data validation, serialization, and documentation generation for these endpoints, allowing you to focus on writing the business logic.
Create Your First FastAPI API
Now, let’s walk through the process of creating your first FastAPI API. We’ll start by setting up a new Python project and installing FastAPI and Uvicorn (a lightning-fast ASGI server).
Step 1: Install FastAPI and Uvicorn
pip install fastapi uvicorn
Step 2: Create a new Python file (e.g., main.py
) and define your FastAPI application.
from fastapi import FastAPI # Create a FastAPI instance app = FastAPI() # Define a simple endpoint @app.get("/") async def read_root(): return {"message": "Hello, World!"}
Step 3: Run the FastAPI application using Uvicorn.
uvicorn main:app --reload ############################## # The command uvicorn main:app refers to: # main: the file main.py (the Python "module"). # app: the object created inside of main.py with the line app = FastAPI(). # --reload: make the server restart after code changes. Only use this for development. ###############################
Step 4: Test your API
Open your web browser and navigate to http://localhost:8000
. You should see a JSON response with the message “Hello, World!”
Congratulations! You’ve successfully created your first FastAPI API. From here, you can continue to expand and customize your API by adding new endpoints, integrating with databases, and implementing business logic as needed.
FastAPI Documentation
FastAPI comes with built-in support for automatically generating interactive API documentation, making it easy for developers to understand and test API endpoints. The documentation is based on the OpenAPI standard (formerly known as Swagger), which provides a standardized way to describe and document RESTful APIs.
How to Access FastAPI Documentation:
FastAPI documentation is automatically generated and hosted by the FastAPI application itself. You can access the documentation by navigating to the /docs
endpoint of your FastAPI application in a web browser. For example, if your FastAPI application is running locally on port 8000, you can access the documentation at http://localhost:8000/docs
.
Swagger UI Integration:
FastAPI uses Swagger UI, a popular tool for visualizing and interacting with OpenAPI-compliant APIs, to generate its documentation. Swagger UI provides a sleek and user-friendly interface for exploring API endpoints, making it easy for developers to understand and use your API.
FastAPI() Parameters
When initializing a FastAPI application, the FastAPI()
function can take several parameters to configure various aspects of the application. Let’s delve into each parameter and its purpose:
def __init__( self: AppType, *, debug: Annotated[ bool, Doc( """ Boolean indicating if debug tracebacks should be returned on server errors. Read more in the [Starlette docs for Applications](https://www.starlette.io/applications/#instantiating-the-application). """ ), ] = False, routes: Annotated[ Optional[List[BaseRoute]], Doc( """ **Note**: you probably shouldn't use this parameter, it is inherited from Starlette and supported for compatibility. --- A list of routes to serve incoming HTTP and WebSocket requests. """ ), deprecated( """ You normally wouldn't use this parameter with FastAPI, it is inherited from Starlette and supported for compatibility. In FastAPI, you normally would use the *path operation methods*, like `app.get()`, `app.post()`, etc. """ ), ] = None, title: Annotated[ str, Doc( """ The title of the API. It will be added to the generated OpenAPI (e.g. visible at `/docs`). Read more in the [FastAPI docs for Metadata and Docs URLs](https://fastapi.tiangolo.com/tutorial/metadata/#metadata-for-api). **Example** ```python from fastapi import FastAPI app = FastAPI(title="ChimichangApp") ``` """ ), ] = "FastAPI", summary: Annotated[ Optional[str], Doc( """ A short summary of the API. It will be added to the generated OpenAPI (e.g. visible at `/docs`). Read more in the [FastAPI docs for Metadata and Docs URLs](https://fastapi.tiangolo.com/tutorial/metadata/#metadata-for-api). **Example** ```python from fastapi import FastAPI app = FastAPI(summary="Deadpond's favorite app. Nuff said.") ``` """ ), ] = None, description: Annotated[ str, Doc( ''' A description of the API. Supports Markdown (using [CommonMark syntax](https://commonmark.org/)). It will be added to the generated OpenAPI (e.g. visible at `/docs`). Read more in the [FastAPI docs for Metadata and Docs URLs](https://fastapi.tiangolo.com/tutorial/metadata/#metadata-for-api). **Example** ```python from fastapi import FastAPI app = FastAPI( description=""" ChimichangApp API helps you do awesome stuff. 🚀 ## Items You can **read items**. ## Users You will be able to: * **Create users** (_not implemented_). * **Read users** (_not implemented_). """ ) ``` ''' ), ] = "", version: Annotated[ str, Doc( """ The version of the API. **Note** This is the version of your application, not the version of the OpenAPI specification nor the version of FastAPI being used. It will be added to the generated OpenAPI (e.g. visible at `/docs`). Read more in the [FastAPI docs for Metadata and Docs URLs](https://fastapi.tiangolo.com/tutorial/metadata/#metadata-for-api). **Example** ```python from fastapi import FastAPI app = FastAPI(version="0.0.1") ``` """ ), ] = "0.1.0", openapi_url: Annotated[ Optional[str], Doc( """ The URL where the OpenAPI schema will be served from. If you set it to `None`, no OpenAPI schema will be served publicly, and the default automatic endpoints `/docs` and `/redoc` will also be disabled. Read more in the [FastAPI docs for Metadata and Docs URLs](https://fastapi.tiangolo.com/tutorial/metadata/#openapi-url). **Example** ```python from fastapi import FastAPI app = FastAPI(openapi_url="/api/v1/openapi.json") ``` """ ), ] = "/openapi.json", openapi_tags: Annotated[ Optional[List[Dict[str, Any]]], Doc( """ A list of tags used by OpenAPI, these are the same `tags` you can set in the *path operations*, like: * `@app.get("/users/", tags=["users"])` * `@app.get("/items/", tags=["items"])` The order of the tags can be used to specify the order shown in tools like Swagger UI, used in the automatic path `/docs`. It's not required to specify all the tags used. The tags that are not declared MAY be organized randomly or based on the tools' logic. Each tag name in the list MUST be unique. The value of each item is a `dict` containing: * `name`: The name of the tag. * `description`: A short description of the tag. [CommonMark syntax](https://commonmark.org/) MAY be used for rich text representation. * `externalDocs`: Additional external documentation for this tag. If provided, it would contain a `dict` with: * `description`: A short description of the target documentation. [CommonMark syntax](https://commonmark.org/) MAY be used for rich text representation. * `url`: The URL for the target documentation. Value MUST be in the form of a URL. Read more in the [FastAPI docs for Metadata and Docs URLs](https://fastapi.tiangolo.com/tutorial/metadata/#metadata-for-tags). **Example** ```python from fastapi import FastAPI tags_metadata = [ { "name": "users", "description": "Operations with users. The **login** logic is also here.", }, { "name": "items", "description": "Manage items. So _fancy_ they have their own docs.", "externalDocs": { "description": "Items external docs", "url": "https://fastapi.tiangolo.com/", }, }, ] app = FastAPI(openapi_tags=tags_metadata) ``` """ ), ] = None, servers: Annotated[ Optional[List[Dict[str, Union[str, Any]]]], Doc( """ A `list` of `dict`s with connectivity information to a target server. You would use it, for example, if your application is served from different domains and you want to use the same Swagger UI in the browser to interact with each of them (instead of having multiple browser tabs open). Or if you want to leave fixed the possible URLs. If the servers `list` is not provided, or is an empty `list`, the default value would be a a `dict` with a `url` value of `/`. Each item in the `list` is a `dict` containing: * `url`: A URL to the target host. This URL supports Server Variables and MAY be relative, to indicate that the host location is relative to the location where the OpenAPI document is being served. Variable substitutions will be made when a variable is named in `{`brackets`}`. * `description`: An optional string describing the host designated by the URL. [CommonMark syntax](https://commonmark.org/) MAY be used for rich text representation. * `variables`: A `dict` between a variable name and its value. The value is used for substitution in the server's URL template. Read more in the [FastAPI docs for Behind a Proxy](https://fastapi.tiangolo.com/advanced/behind-a-proxy/#additional-servers). **Example** ```python from fastapi import FastAPI app = FastAPI( servers=[ {"url": "https://stag.example.com", "description": "Staging environment"}, {"url": "https://prod.example.com", "description": "Production environment"}, ] ) ``` """ ), ] = None, dependencies: Annotated[ Optional[Sequence[Depends]], Doc( """ A list of global dependencies, they will be applied to each *path operation*, including in sub-routers. Read more about it in the [FastAPI docs for Global Dependencies](https://fastapi.tiangolo.com/tutorial/dependencies/global-dependencies/). **Example** ```python from fastapi import Depends, FastAPI from .dependencies import func_dep_1, func_dep_2 app = FastAPI(dependencies=[Depends(func_dep_1), Depends(func_dep_2)]) ``` """ ), ] = None, default_response_class: Annotated[ Type[Response], Doc( """ The default response class to be used. Read more in the [FastAPI docs for Custom Response - HTML, Stream, File, others](https://fastapi.tiangolo.com/advanced/custom-response/#default-response-class). **Example** ```python from fastapi import FastAPI from fastapi.responses import ORJSONResponse app = FastAPI(default_response_class=ORJSONResponse) ``` """ ), ] = Default(JSONResponse), redirect_slashes: Annotated[ bool, Doc( """ Whether to detect and redirect slashes in URLs when the client doesn't use the same format. **Example** ```python from fastapi import FastAPI app = FastAPI(redirect_slashes=True) # the default @app.get("/items/") async def read_items(): return [{"item_id": "Foo"}] ``` With this app, if a client goes to `/items` (without a trailing slash), they will be automatically redirected with an HTTP status code of 307 to `/items/`. """ ), ] = True, docs_url: Annotated[ Optional[str], Doc( """ The path to the automatic interactive API documentation. It is handled in the browser by Swagger UI. The default URL is `/docs`. You can disable it by setting it to `None`. If `openapi_url` is set to `None`, this will be automatically disabled. Read more in the [FastAPI docs for Metadata and Docs URLs](https://fastapi.tiangolo.com/tutorial/metadata/#docs-urls). **Example** ```python from fastapi import FastAPI app = FastAPI(docs_url="/documentation", redoc_url=None) ``` """ ), ] = "/docs", redoc_url: Annotated[ Optional[str], Doc( """ The path to the alternative automatic interactive API documentation provided by ReDoc. The default URL is `/redoc`. You can disable it by setting it to `None`. If `openapi_url` is set to `None`, this will be automatically disabled. Read more in the [FastAPI docs for Metadata and Docs URLs](https://fastapi.tiangolo.com/tutorial/metadata/#docs-urls). **Example** ```python from fastapi import FastAPI app = FastAPI(docs_url="/documentation", redoc_url="redocumentation") ``` """ ), ] = "/redoc", swagger_ui_oauth2_redirect_url: Annotated[ Optional[str], Doc( """ The OAuth2 redirect endpoint for the Swagger UI. By default it is `/docs/oauth2-redirect`. This is only used if you use OAuth2 (with the "Authorize" button) with Swagger UI. """ ), ] = "/docs/oauth2-redirect", swagger_ui_init_oauth: Annotated[ Optional[Dict[str, Any]], Doc( """ OAuth2 configuration for the Swagger UI, by default shown at `/docs`. Read more about the available configuration options in the [Swagger UI docs](https://swagger.io/docs/open-source-tools/swagger-ui/usage/oauth2/). """ ), ] = None, middleware: Annotated[ Optional[Sequence[Middleware]], Doc( """ List of middleware to be added when creating the application. In FastAPI you would normally do this with `app.add_middleware()` instead. Read more in the [FastAPI docs for Middleware](https://fastapi.tiangolo.com/tutorial/middleware/). """ ), ] = None, exception_handlers: Annotated[ Optional[ Dict[ Union[int, Type[Exception]], Callable[[Request, Any], Coroutine[Any, Any, Response]], ] ], Doc( """ A dictionary with handlers for exceptions. In FastAPI, you would normally use the decorator `@app.exception_handler()`. Read more in the [FastAPI docs for Handling Errors](https://fastapi.tiangolo.com/tutorial/handling-errors/). """ ), ] = None, on_startup: Annotated[ Optional[Sequence[Callable[[], Any]]], Doc( """ A list of startup event handler functions. You should instead use the `lifespan` handlers. Read more in the [FastAPI docs for `lifespan`](https://fastapi.tiangolo.com/advanced/events/). """ ), ] = None, on_shutdown: Annotated[ Optional[Sequence[Callable[[], Any]]], Doc( """ A list of shutdown event handler functions. You should instead use the `lifespan` handlers. Read more in the [FastAPI docs for `lifespan`](https://fastapi.tiangolo.com/advanced/events/). """ ), ] = None, lifespan: Annotated[ Optional[Lifespan[AppType]], Doc( """ A `Lifespan` context manager handler. This replaces `startup` and `shutdown` functions with a single context manager. Read more in the [FastAPI docs for `lifespan`](https://fastapi.tiangolo.com/advanced/events/). """ ), ] = None, terms_of_service: Annotated[ Optional[str], Doc( """ A URL to the Terms of Service for your API. It will be added to the generated OpenAPI (e.g. visible at `/docs`). Read more at the [FastAPI docs for Metadata and Docs URLs](https://fastapi.tiangolo.com/tutorial/metadata/#metadata-for-api). **Example** ```python app = FastAPI(terms_of_service="http://example.com/terms/") ``` """ ), ] = None, contact: Annotated[ Optional[Dict[str, Union[str, Any]]], Doc( """ A dictionary with the contact information for the exposed API. It can contain several fields. * `name`: (`str`) The name of the contact person/organization. * `url`: (`str`) A URL pointing to the contact information. MUST be in the format of a URL. * `email`: (`str`) The email address of the contact person/organization. MUST be in the format of an email address. It will be added to the generated OpenAPI (e.g. visible at `/docs`). Read more at the [FastAPI docs for Metadata and Docs URLs](https://fastapi.tiangolo.com/tutorial/metadata/#metadata-for-api). **Example** ```python app = FastAPI( contact={ "name": "Deadpoolio the Amazing", "url": "http://x-force.example.com/contact/", "email": "[email protected]", } ) ``` """ ), ] = None, license_info: Annotated[ Optional[Dict[str, Union[str, Any]]], Doc( """ A dictionary with the license information for the exposed API. It can contain several fields. * `name`: (`str`) **REQUIRED** (if a `license_info` is set). The license name used for the API. * `identifier`: (`str`) An [SPDX](https://spdx.dev/) license expression for the API. The `identifier` field is mutually exclusive of the `url` field. Available since OpenAPI 3.1.0, FastAPI 0.99.0. * `url`: (`str`) A URL to the license used for the API. This MUST be the format of a URL. It will be added to the generated OpenAPI (e.g. visible at `/docs`). Read more at the [FastAPI docs for Metadata and Docs URLs](https://fastapi.tiangolo.com/tutorial/metadata/#metadata-for-api). **Example** ```python app = FastAPI( license_info={ "name": "Apache 2.0", "url": "https://www.apache.org/licenses/LICENSE-2.0.html", } ) ``` """ ), ] = None, openapi_prefix: Annotated[ str, Doc( """ A URL prefix for the OpenAPI URL. """ ), deprecated( """ "openapi_prefix" has been deprecated in favor of "root_path", which follows more closely the ASGI standard, is simpler, and more automatic. """ ), ] = "", root_path: Annotated[ str, Doc( """ A path prefix handled by a proxy that is not seen by the application but is seen by external clients, which affects things like Swagger UI. Read more about it at the [FastAPI docs for Behind a Proxy](https://fastapi.tiangolo.com/advanced/behind-a-proxy/). **Example** ```python from fastapi import FastAPI app = FastAPI(root_path="/api/v1") ``` """ ), ] = "", root_path_in_servers: Annotated[ bool, Doc( """ To disable automatically generating the URLs in the `servers` field in the autogenerated OpenAPI using the `root_path`. Read more about it in the [FastAPI docs for Behind a Proxy](https://fastapi.tiangolo.com/advanced/behind-a-proxy/#disable-automatic-server-from-root_path). **Example** ```python from fastapi import FastAPI app = FastAPI(root_path_in_servers=False) ``` """ ), ] = True, responses: Annotated[ Optional[Dict[Union[int, str], Dict[str, Any]]], Doc( """ Additional responses to be shown in OpenAPI. It will be added to the generated OpenAPI (e.g. visible at `/docs`). Read more about it in the [FastAPI docs for Additional Responses in OpenAPI](https://fastapi.tiangolo.com/advanced/additional-responses/). And in the [FastAPI docs for Bigger Applications](https://fastapi.tiangolo.com/tutorial/bigger-applications/#include-an-apirouter-with-a-custom-prefix-tags-responses-and-dependencies). """ ), ] = None, callbacks: Annotated[ Optional[List[BaseRoute]], Doc( """ OpenAPI callbacks that should apply to all *path operations*. It will be added to the generated OpenAPI (e.g. visible at `/docs`). Read more about it in the [FastAPI docs for OpenAPI Callbacks](https://fastapi.tiangolo.com/advanced/openapi-callbacks/). """ ), ] = None, webhooks: Annotated[ Optional[routing.APIRouter], Doc( """ Add OpenAPI webhooks. This is similar to `callbacks` but it doesn't depend on specific *path operations*. It will be added to the generated OpenAPI (e.g. visible at `/docs`). **Note**: This is available since OpenAPI 3.1.0, FastAPI 0.99.0. Read more about it in the [FastAPI docs for OpenAPI Webhooks](https://fastapi.tiangolo.com/advanced/openapi-webhooks/). """ ), ] = None, deprecated: Annotated[ Optional[bool], Doc( """ Mark all *path operations* as deprecated. You probably don't need it, but it's available. It will be added to the generated OpenAPI (e.g. visible at `/docs`). Read more about it in the [FastAPI docs for Path Operation Configuration](https://fastapi.tiangolo.com/tutorial/path-operation-configuration/). """ ), ] = None, include_in_schema: Annotated[ bool, Doc( """ To include (or not) all the *path operations* in the generated OpenAPI. You probably don't need it, but it's available. This affects the generated OpenAPI (e.g. visible at `/docs`). Read more about it in the [FastAPI docs for Query Parameters and String Validations](https://fastapi.tiangolo.com/tutorial/query-params-str-validations/#exclude-from-openapi). """ ), ] = True, swagger_ui_parameters: Annotated[ Optional[Dict[str, Any]], Doc( """ Parameters to configure Swagger UI, the autogenerated interactive API documentation (by default at `/docs`). Read more about it in the [FastAPI docs about how to Configure Swagger UI](https://fastapi.tiangolo.com/how-to/configure-swagger-ui/). """ ), ] = None, generate_unique_id_function: Annotated[ Callable[[routing.APIRoute], str], Doc( """ Customize the function used to generate unique IDs for the *path operations* shown in the generated OpenAPI. This is particularly useful when automatically generating clients or SDKs for your API. Read more about it in the [FastAPI docs about how to Generate Clients](https://fastapi.tiangolo.com/advanced/generate-clients/#custom-generate-unique-id-function). """ ), ] = Default(generate_unique_id), separate_input_output_schemas: Annotated[ bool, Doc( """ Whether to generate separate OpenAPI schemas for request body and response body when the results would be more precise. This is particularly useful when automatically generating clients. For example, if you have a model like: ```python from pydantic import BaseModel class Item(BaseModel): name: str tags: list[str] = [] ``` When `Item` is used for input, a request body, `tags` is not required, the client doesn't have to provide it. But when using `Item` for output, for a response body, `tags` is always available because it has a default value, even if it's just an empty list. So, the client should be able to always expect it. In this case, there would be two different schemas, one for input and another one for output. """ ), ] = True, **extra: Annotated[ Any, Doc( """ Extra keyword arguments to be stored in the app, not used by FastAPI anywhere. """ ), ], )
These parameters give you fine-grained control over the configuration of your FastAPI application, allowing you to customize its behavior to suit your specific needs. By leveraging these parameters effectively, you can create robust and highly customizable APIs with FastAPI.
In this document, we’ve explored the fundamental concepts of APIs, REST architecture, and FastAPI. This is the first in the Mastering FastAPI tutorial series. By understanding these concepts and learning how to create APIs with FastAPI, you are well-equipped to build robust and efficient web applications that meet the demands of today’s interconnected world. Stay tuned …