Output formatting is the process of arranging or formatting data in a specific way so that it can be presented or displayed in a readable and structured format. In Python, output formatting is an important concept, as it allows you to control the way that data is printed to the console or displayed to the user. In this article, we will cover the various methods of output formatting in Python, including the print()
function, old modulo method (% string interpolation), string formatting with placeholders(.format()), and the newer f-strings.
Using the print() Function
The simplest way to output data in Python is to use the print()
function. This function takes one or more arguments and displays them on the screen. For example, the following code will output the string “Hello, World!” to the console:
print("Hello, World!") #output: Hello, World!
You can also use the print()
function to output variables. For example, the following code will output the value of the variable x
:
x = 42 print(x) #output:42
By default, the print()
function separates multiple arguments with a space character. However, you can change this behavior by specifying a different separator using the sep
parameter. For example, the following code will separate the arguments with a hyphen instead of a space:
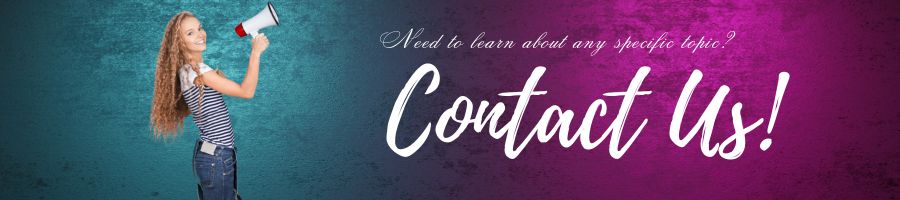
print("Hello", "World", sep="-") #output: Hello-World
The print()
function also adds a newline character (\n
) to the end of each output line by default. You can change this behavior by specifying a different end character using the end
parameter. For example, the following code will use a semicolon (;
) as the end character:
print("Hello", "World", end=";") #output:Hello World;
Using the % Operator:
The %
operator is used to format strings in Python. It is also known as the string formatting operator. The basic syntax of using the %
operator is as follows:
formatted_string = "String with formatting %formatting_specifier" % (variable1, variable2, ...)
The %
operator takes a string on the left-hand side and a tuple on the right-hand side. The tuple contains values that will be used to replace the formatting placeholders in the string. The %
operator works by replacing the formatting placeholders with the corresponding values from the tuple.
Formatting Specifiers:
Formatting specifiers are used to control how values are formatted in the output string. They are added after the %
character and before the type character. The basic syntax for a formatting specifier is as follows:
%[flags][width][.precision]type
Here is a detailed explanation of each component of the formatting specifier:
- Flags: Flags are optional and can be used to modify the formatting. There are three flags that can be used:
+
: This flag is used to force the sign of a number to be displayed, even if it is positive.-
: This flag is used to left-justify the output.0
: This flag is used to fill the width of the output with leading zeros.
- Width: Width is also optional and specifies the minimum number of characters to be used for the output. If the output is shorter than the specified width, the output is padded with spaces by default.
- Precision: Precision is also optional and specifies the number of decimal places to be used for floating-point numbers. For non-floating-point values, precision is ignored.
- Type: Type specifies the type of the value being formatted. There are several types available:
%
: This is used to output a literal%
character.d
: This is used for integer values.f
: This is used for floating-point values.s
: This is used for strings.x
: This is used for hexadecimal values, with lowercase letters.X
: This is used for hexadecimal values, with uppercase letters.o
: This is used for octal values.e
: This is used for scientific notation with lowercasee
.E
: This is used for scientific notation with uppercaseE
.
Examples: Here are some examples of using the %
operator for string formatting:
# Formatting integer values number_of_students = 25 message = "There are %d students in the class." % number_of_students print(message) # Output: There are 25 students in the class. # Formatting floating-point values price = 12.99 message = "The price is %.2f dollars." % price print(message) # Output: The price is 12.99 dollars. # Formatting strings name = "John" age = 25 message = "My name is %s and I am %d years old." % (name, age) print(message) # Output: My name is John and I am 25 years old.
Format specifiers flags:
Flag | Meaning |
---|---|
0 | The conversion will be zero padded for numeric values. |
+ | The sign of the number will be shown for both positive and negative numbers. |
- | The converted value is left aligned (default is right aligned) |
(space) | A blank space is inserted before positive numbers. |
# | Alternate form (octal and hexadecimal numbers will have a prefix). |
, | Adds a comma as a thousands separator. |
: | Introduces the format specifier. |
Here’s a more detailed explanation of each flag:
0
: When the0
flag is used with a numeric conversion type, the resulting string will be zero padded to the field width specified. For example, if we havex = 5
and use the format specifier%03d
, the resulting string will be'005'
.+
: When the+
flag is used with a numeric conversion type, the resulting string will always include the sign, even if it’s positive. For example, if we havex = 5
and use the format specifier'%+d'
, the resulting string will be'+5'
.-
: When the-
flag is used, the resulting string will be left aligned. By default, the resulting string is right aligned. For example, if we havex = 'hello'
and use the format specifier'%-10s'
, the resulting string will be'hello '
.- (space): When the space flag is used with a numeric conversion type, a blank space is inserted before positive numbers. For example, if we have
x = 5
and use the format specifier'% d'
, the resulting string will be' 5'
. If the number is negative, the-
sign will be used instead. #
: The#
flag is used to indicate that the alternate form of the conversion should be used. For example, with ano
conversion, the resulting string will have a leading0o
. With anx
orX
conversion, the resulting string will have a leading0x
or0X
, respectively.,
: When the,
flag is used with a numeric conversion type, a comma is inserted as a thousands separator. For example, if we havex = 1000000
and use the format specifier'%d'
, the resulting string will be'1000000'
. If we use the format specifier'%,'
, the resulting string will be'1,000,000'
.:
: The:
character is used to introduce the format specifier. This allows for more advanced formatting options, such as specifying the width and precision of the resulting string.
Format Specifiers Width:
The width field in format specifiers allows you to specify the minimum number of characters that should be used to represent the value being formatted. You can specify the width by adding an integer before the conversion specifier, separated by a :
character. For example, "{:5d}"
specifies a width of 5 characters for the integer conversion.
If the value being formatted is shorter than the specified width, it will be padded with spaces on the left (by default) to reach the minimum width. If the value is longer than the specified width, it will be displayed using its full length.
You can also specify the alignment of the value within the available space by adding an alignment option before the width specifier. The available alignment options are:
<
: left-align the value within the available space. This is the default if no alignment option is specified.>
: right-align the value within the available space.^
: center the value within the available space.=
: add padding after any sign or leading zeros to ensure the field width is reached before the value is displayed. This option is only valid for numeric types.
Here are some examples of using the width field with different alignment options:
# left-aligned within 10 spaces print("{:<10}".format("hello")) # right-aligned within 10 spaces print("{:>10}".format("hello")) # centered within 10 spaces print("{:^10}".format("hello")) # add padding to ensure 10 spaces before the value print("{:=10}".format(-1234))
Output:
hello hello hello -1234
In the first example, the <
option is used to left-align the value “hello” within 10 spaces. In the second example, the >
option is used to right-align the value within 10 spaces. In the third example, the ^
option is used to center the value within 10 spaces. In the fourth example, the =
option is used to add padding after the negative sign to ensure that the field width of 10 spaces is reached before the value is displayed.
Precision Specifiers
Precision specifiers are used to control the precision or number of decimal places displayed for floating point values. They can be used with the %
operator to format strings. The syntax for using precision specifiers is as follows:
%[flags][width][.precision]specifier
The .
character followed by a number specifies the precision of the floating point number. For example, %0.3f
would display a floating point number with 3 decimal places. If the number has fewer decimal places than the specified precision, zeros will be added to the end. If the number has more decimal places than the specified precision, the number will be rounded.
Here’s an example:
>>> num = 3.141592653589793 >>> print("The value of pi is approximately %0.3f." % num) The value of pi is approximately 3.142.
In this example, the %0.3f
specifier formats the num
variable as a floating point number with 3 decimal places.
In addition to specifying precision for floating point numbers, precision specifiers can also be used with other specifiers such as %s
and %d
. For %s
, the precision specifies the maximum number of characters to display. For %d
, the precision specifies the minimum number of digits to display, with leading zeros added if necessary.
Here’s an example:
>>> text = "Hello, world!" >>> print("The first five characters of the string are '%.5s'." % text) The first five characters of the string are 'Hello'.
In this example, the %.5s
specifier formats the text
variable as a string with a maximum of 5 characters.
Precision specifiers can also be combined with width specifiers to control both the width and precision of the formatted value.
Additionally, precision specifiers can be used with the g
or G
specifier to automatically choose between the %f
or %e
(or %E
) format based on the magnitude of the value.
Here’s an example:
>>> num1 = 0.000001 >>> num2 = 1000000 >>> print("%g %g" % (num1, num2)) 1e-06 1e+06
In this example, the %g
specifier formats num1
as 1e-06
and num2
as 1e+06
.
Overall, precision specifiers are useful for controlling the number of decimal places displayed for floating point values and the number of characters displayed for strings and integers.
Type Specifiers
Here is an updated table with %r
added:
Type Specifier | Meaning |
---|---|
%s | String |
%c | Character |
%d or %i | Signed integer |
%u | Unsigned integer |
%o | Octal integer |
%x | Hexadecimal integer (lowercase letters) |
%X | Hexadecimal integer (uppercase letters) |
%e | Exponential notation (with lowercase ‘e’) |
%E | Exponential notation (with uppercase ‘E’) |
%f or %F | Floating-point decimal |
%g or %G | Same as %e or %E if the exponent is less than -4 or greater than or equal to the precision, otherwise same as %f or %F |
%r | “Raw” string representation |
%% | A literal percent sign % |
String Formatting with Placeholders .format()
Another way to format output in Python is to use string formatting with placeholders. This method allows you to create a string template with placeholders that will be replaced by the values of variables at runtime. The placeholders are denoted by curly braces ({}
) and can include optional format specifiers. Here is a template for using .format()
for string formatting in Python:
formatted_string = "Your {} string {} here".format(variable1, variable2)
In this template:
formatted_string
is the string that will be formatted with the specified values of the variables.{}
acts as a placeholder for the values that will be inserted into the string.format()
is a method that is called on the string, and it takes the values that will be inserted into the string as its arguments.variable1
andvariable2
are the values that will be inserted into the string, in the order that they are passed to theformat()
method.
You can use as many placeholders {}
as you need in the string and pass corresponding number of arguments to the format()
method.
Here’s an example:
name = "Alice" age = 30 profession = "programmer" formatted_string = "My name is {}, I am {} years old, and I work as a {}.".format(name, age, profession) print(formatted_string) #output: My name is Alice, I am 30 years old, and I work as a programmer.
x = 42 print("The answer is {}.".format(x))
In this example, the placeholder {}
is replaced by the value of x
. You can also include multiple placeholders in the same string and provide the corresponding values in a tuple:
name = "Alice" age = 27 print("{} is {} years old.".format(name, age))
In this example, the first placeholder {}
is replaced by the value of name
, and the second placeholder is replaced by the value of age
. You can also include format specifiers to control the appearance of the output. For example, you can specify the number of decimal places for a floating-point value using the f
format specifier:
pi = 3.141592653589793 print("Pi is approximately {:.2f}.".format(pi))
In this example, the {:.2f}
format specifier indicates that the value of pi
should be formatted as a floating-point number with two decimal places.
F-Strings
The newest way to format output in Python is to use f-strings. This feature was introduced in Python 3.6 and provides a more concise and readable way to format strings. F-strings are similar to string formatting with placeholders but use a different syntax.
Here’s an example of using an f-string to output the value of the variable x
:
x = 42 print(f"The answer is {x}.")
In this example, the string is prefixed with the letter f
, which indicates that it is an f-string. The placeholder {x}
is replaced by the value of the variable x
. You can also include expressions inside the placeholders, which will be evaluated at runtime. For example:
x = 4 print(f"The result of 2 times {x} is {2*x}.")
In this example, the f-string includes an expression inside the second placeholder, which evaluates to 2*x
and is replaced by the value 8
.
F-strings also support format specifiers, which are specified after the variable name or expression inside the placeholder. For example:
pi = 3.141592653589793 print(f"Pi is approximately {pi:.2f}.")
In this example, the format specifier :.2f
indicates that the value of pi
should be formatted as a floating-point number with two decimal places.
In Python, there are several format specifiers that can be used to control the appearance of formatted output. These specifiers can be used with string formatting with placeholders and f-strings. Details about format specifiers have been given above. In addition to above, it also supports width specifiers:
Width Specifiers
{:<width}
: Left-align the value within a field of the specified width. For example,{:<10}
will left-align the value in a field of width 10.{:>width}
: Right-align the value within a field of the specified width. For example,{:>10}
will right-align the value in a field of width 10.{:^width}
: Center the value within a field of the specified width. For example,{:^10}
will center the value in a field of width 10.
Here are some examples of using these specifiers:
x = "Hello" y = "World" # Left-align the value of x in a field of width 10 print("{:<10} {}".format(x, y)) # Right-align
Other String Formatting Methods:
There are some other string formatting methods. I can explain in detail about the string methods ljust()
, rjust()
, center()
, and zfill()
in Python.
ljust(width, fillchar)
:
The ljust()
method returns a left-justified string of a specified width. It pads the original string with a specified fill character on the right if the original string is shorter than the specified width.
Syntax: string.ljust(width[, fill
char])
Parameters:
width
: an integer representing the width of the returned stringfillchar
(optional): a character to fill the remaining space. Default is whitespace' '
.
Example:
text = "Hello" print(text.ljust(10, '-')) # Output: "Hello-----"
rjust(width, fillchar)
:
The rjust()
method returns a right-justified string of a specified width. It pads the original string with a specified fill character on the left if the original string is shorter than the specified width.
Syntax: string.rjust(width[, fillchar])
Parameters:
width
: an integer representing the width of the returned stringfillchar
(optional): a character to fill the remaining space. Default is whitespace' '
. Example:
text = "Hello" print(text.rjust(10, '-')) # Output: "-----Hello"
center(width, fillchar)
:
The center()
method returns a centered string of a specified width. It pads the original string with a specified fill character on both sides if the original string is shorter than the specified width.
Syntax: string.center(width[, fillchar])
Parameters:
width
: an integer representing the width of the returned stringfillchar
(optional): a character to fill the remaining space. Default is whitespace' '
.
Example:
text = "Hello" print(text.center(10, '-')) # Output: "--Hello---"
zfill(width)
:
The zfill()
method returns a copy of the string with ‘0’ characters padded to the left. It is typically used to create strings with leading zeros for numeric formatting.
Syntax: string.zfill(width)
Parameters:
width
: an integer representing the width of the returned string, including the original string and ‘0’ characters.
Example:
num = "123" print(num.zfill(5)) # Output: "00123"
These string methods can be useful in formatting text or numbers in a visually appealing way.
This article provides an overview of the main methods of output formatting in Python, including the print()
function, string formatting with placeholders, and f-strings. It also includes examples of each method to help you understand how to use them in your own code. However, there are many more advanced formatting options that are not covered here, such as the str.format_map()
method, which allows you to use dictionaries to format strings, and the locale
module, which provides support for formatting numbers and dates according to the user’s locale. If you need to format output in more complex ways, I would recommend consulting the Python documentation or a more in-depth tutorial on the topic.
The codes (if any) mentioned in this post can be downloaded from github.com. Share the post with someone you think can benefit from the information