Python is often celebrated for its simplicity and flexibility, largely thanks to its dynamic typing system. However, as projects grow in complexity, this flexibility can sometimes lead to ambiguity, bugs, or maintenance challenges. Enter static typing – a way to bring more clarity and robustness to your code without sacrificing Python’s inherent ease of use.
This guide will walk you through Python’s static typing system with practical examples, explaining the why, the how, and the when of static typing.
What Is Static Typing?
In dynamic typing, the type of a variable is determined at runtime:
x = 10 # x is an integer x = "Hello" # Now x is a string
Static typing, on the other hand, involves specifying types explicitly. This helps tools like mypy catch errors during development rather than at runtime. For example:
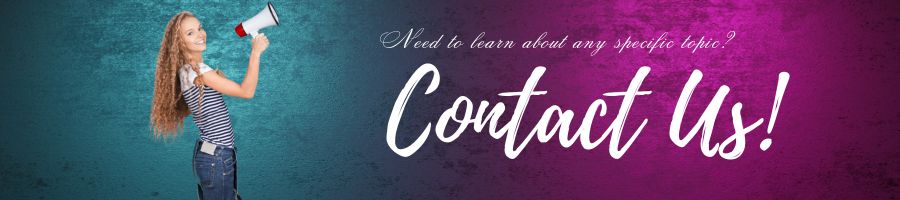
x: int = 10 x = "Hello" # Type checker will raise an error
With static typing, you’re essentially providing hints to both the developer and the tools about what kind of data your program expects.
Why Use Static Typing?
- Catch bugs early: Static typing helps detect type-related errors before your program even runs.
- Improved readability: Explicit types make it easier for others (and your future self) to understand the code.
- Better IDE support: Modern IDEs can leverage type hints for autocompletion, refactoring, and error detection.
- Easier maintenance: In large codebases, knowing the types of variables and function parameters can save hours of debugging.
- Enhanced collaboration: Static types act as documentation, making it easier for teams to collaborate on a project.
How to Use Static Typing in Python
Python introduced static typing in PEP 484 with the typing
module. Let’s explore its features step by step.
1. Basic Type Annotations
You can specify the type of variables, function parameters, and return values:
# Variables age: int = 25 name: str = "Alice" is_student: bool = False # Functions def add(a: int, b: int) -> int: return a + b result = add(5, 10) # Works fine result = add("5", 10) # Type checker will complain
2. Using Complex Types
The typing
module allows you to define complex types such as lists, dictionaries, and tuples:
from typing import List, Dict, Tuple # Lists names: List[str] = ["Alice", "Bob", "Charlie"] # Dictionaries scores: Dict[str, int] = {"Alice": 90, "Bob": 85} # Tuples def get_coordinates() -> Tuple[float, float]: return 12.34, 56.78
3. Optional Types
Sometimes, a variable or parameter can be None
. Use Optional
to indicate this:
from typing import Optional def greet(name: Optional[str] = None) -> str: if name: return f"Hello, {name}!" return "Hello, World!" print(greet()) # Hello, World! print(greet("Alice")) # Hello, Alice!
4. Type Aliases
For readability, you can define aliases for complex types:
from typing import List, Dict Student = Dict[str, int] # Alias for a dictionary with string keys and integer values students: List[Student] = [ {"Alice": 90}, {"Bob": 85} ]
5. Union Types
If a value can be of multiple types, use Union
:
from typing import Union def process_value(value: Union[int, str]) -> str: if isinstance(value, int): return f"Processed integer: {value}" return f"Processed string: {value}" print(process_value(10)) # Processed integer: 10 print(process_value("Hello")) # Processed string: Hello
6. Callable Types
Use Callable
to specify functions as arguments or return types:
from typing import Callable def apply_operation(a: int, b: int, operation: Callable[[int, int], int]) -> int: return operation(a, b) result = apply_operation(5, 3, lambda x, y: x + y) print(result) # 8
7. Generics
Generics allow you to write reusable, type-safe code:
from typing import TypeVar, List T = TypeVar('T') # A generic type variable def get_first_element(elements: List[T]) -> T: return elements[0] print(get_first_element([1, 2, 3])) # 1 print(get_first_element(["a", "b", "c"])) # a
8. Newer Typing Features (Python 3.9+)
Modern Python versions have simplified type annotations with built-in generics:
# Lists and dictionaries without importing typing names: list[str] = ["Alice", "Bob"] scores: dict[str, int] = {"Alice": 90, "Bob": 85}
Python 3.10 introduced |
as a shorthand for Union
:
def process_value(value: int | str) -> str: if isinstance(value, int): return f"Processed integer: {value}" return f"Processed string: {value}"
Type Checking in Action
To enforce static typing, use a type checker like mypy:
- Install mypy:
pip install mypy
- Run mypy on your script:
mypy your_script.py
Mypy will analyze your code and report any type inconsistencies.
Best Libraries and Tools for Static Type Checking
While Python’s built-in typing
module and tools like mypy are the foundation of static typing, other libraries and tools enhance this ecosystem. Here are some noteworthy ones:
- Pyright
- A fast, feature-rich static type checker developed by Microsoft.
- Integrated with Visual Studio Code for real-time type checking.
- Supports type inference and works seamlessly with Python’s type hinting system.
npm install -g pyright
- Pylance
- A Visual Studio Code extension that builds on Pyright.
- Provides enhanced type-checking, autocompletion, and in-editor diagnostics.
- MonkeyType
- Automatically generates type annotations for your code by analyzing runtime data.
- Useful for legacy projects where adding type hints manually can be daunting.
pip install monkeytype
Example usage:monkeytype run your_script.py monkeytype stub your_module
- Pyre
- A performant type checker developed by Meta (formerly Facebook).
- Focuses on scalability and integrates well with large codebases.
pip install pyre-check
- Pydantic
- A data validation and parsing library that leverages Python’s type hints.
- Commonly used in FastAPI and other frameworks to enforce data integrity.
- Automatically validates and converts data, raising errors for mismatched types.
pip install pydantic
Example usage:from pydantic import BaseModel class User(BaseModel): id: int name: str is_active: bool = True user = User(id=123, name="Alice") print(user.dict()) # {'id': 123, 'name': 'Alice', 'is_active': True} # Will raise a validation error invalid_user = User(id="123", name=123