Structuring your Python code well can make it more readable, maintainable, and scalable. Structuring a program involves organizing the code into logical and modular components, such as functions, classes, modules, and packages. It also involves following best practices for naming conventions, code formatting, documentation, and error handling.
The need to structure a Python program arises from the fact that as a program grows in size and complexity, it becomes harder to manage and modify. A well-structured program helps to reduce the complexity and make it more manageable by breaking it down into smaller and more manageable components. This makes it easier to debug and maintain, as well as allowing for easier collaboration with other developers.
Structuring a program also makes it easier to reuse code, as the modular components can be reused in other parts of the program or in other programs altogether. This can save time and effort, as well as ensuring consistency and reliability across different programs.
By following these tips, you can structure your Python code in a way that makes it easy to read, understand, and maintain.
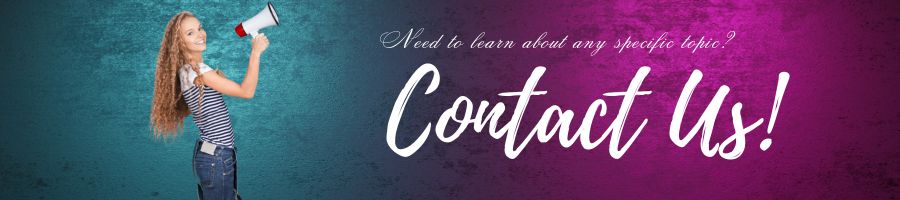
- Define the Purpose of the Program:
Before you begin writing any code, it’s important to have a clear understanding of what your program needs to do. Take some time to define the purpose of your program and identify the key features and functionalities it should have.
For example, if you were writing a program to calculate the area of a circle, the purpose of the program would be to provide a simple and accurate way to calculate the area of a circle given its radius. Knowing the purpose of the program helps to ensure that the code stays focused and meets the needs of its users. It also helps to avoid feature creep, where the program tries to do too many things and becomes bloated or difficult to use.
- Choose a Naming Convention:
Python has several naming conventions, such as snake_case, camelCase, and PascalCase, among others. Adhering to a consistent naming convention makes code more readable, understandable, and maintainable, especially when working collaboratively or when returning to code after some time. Python has several naming conventions, but the most commonly used ones are:
- Snake case: In snake case, words are separated by underscores, and all lowercase. For example:
my_variable
,my_function
. - Camel case: In camel case, the first word is lowercase, and each subsequent word is capitalized. For example:
myVariable
,myFunction
. - Pascal case: In Pascal case, the first letter of each word is capitalized. For example:
MyVariable
,MyFunction
.
It is recommended to follow the PEP 8 style guide, which is widely accepted and considered a standard in the Python community. PEP 8 recommends using snake_case for variable and function names, PascalCase for class names, and lowercase for modules and packages. It also helps to avoid potential errors that may arise due to typos or inconsistencies in naming conventions.
- Use Modular Design:
Modular design is the process of dividing a program into separate modules or functions, each with a specific purpose. This makes your code easier to read and understand, and helps you isolate and fix bugs more easily. Here’s an example of how to divide your code into modules:
my_project/ main.py utils/ __init__.py file_utils.py math_utils.py
In this example, the main.py file is the entry point of the program. The utils folder contains two sub-modules: file_utils.py, which contains functions for reading and writing files, and math_utils.py, which contains functions for performing mathematical operations.
- Follow PEP 8 style guide:
PEP 8 is a style guide for Python code. Adhering to PEP 8 makes your code more consistent and easier to read by others. Here are some key points of PEP 8:
- Use 4 spaces for indentation
- Use meaningful variable and function names
- Limit line length to 79 characters
- Avoid Hard coding values/configurations
Here’s an example of how to follow PEP 8:
# Good def calculate_total_price(price, quantity): return price * quantity # Bad def calculate(price, quantity): return price*quantity
In the example above, the first function adheres to PEP 8 by using descriptive function and variable names, using spaces around the *
operator, and keeping the line length under 79 characters. The second function violates PEP 8 by using a short function name, no spaces around the *
operator, and exceeding the 79 character limit.
- Use comments and docstrings:
Comments and docstrings help explain what the code is doing and how to use it. Here’s an example of how to use comments and docstrings:
def calculate_total_price(price, quantity): """Calculate the total price of an item. Args: price (float): The price of the item. quantity (int): The quantity of the item. Returns: float: The total price of the item. """ return price * quantity
In this example, the docstring explains what the function does, what arguments it expects, and what it returns. This makes it easier for others to understand and use the function.
- Use meaningful variable and function names:
Using descriptive variable and function names makes your code easier to read and understand. Here’s an example:
# Good def calculate_total_price(item_price, item_quantity): return item_price * item_quantity # Bad def calc(a, b): return a * b
In this example, the first function uses descriptive variable names that explain what the function does. The second function uses short variable names that do not provide any information about what the function does.
- Handle errors and exceptions:
Handling errors and exceptions properly can prevent your code from crashing unexpectedly. Here’s an example of how to handle exceptions:
try: x = int(input("Enter a number: ")) y = int(input("Enter another number: ")) print(x / y) except ValueError: print("Invalid input") except ZeroDivisionError: print("Cannot divide by zero")
In this example, we use a try/except block to catch two possible exceptions that might occur. If the user enters invalid input, a ValueError is raised and the message “Invalid input” is printed. If the user tries to divide by zero, a ZeroDivisionError is raised and the message “Cannot divide by zero” is printed.
- Write Test Scripts
Test scripts are an essential part of structuring a Python program as they help to ensure that the program works correctly and meets the desired requirements. Test scripts are used to check whether the various components of the program, such as functions, classes, and modules, are working as expected.
Test scripts are typically written using a testing framework like unittest
or pytest
. These frameworks provide a set of tools and utilities that make it easy to write, run, and manage test scripts. Test scripts are written to simulate different scenarios and edge cases, to ensure that the program behaves as expected in all situations.
The use of test scripts can also help in the development process as they encourage a test-driven development (TDD) approach. This approach involves writing test scripts before writing the actual code, and then writing the code to meet the requirements of the tests. This helps to ensure that the code is well-structured, modular, and meets the desired functionality.
The codes (if any) mentioned in this post can be downloaded from github.com. Share the post with someone you think can benefit from the information