When learning Python, one of the key aspects you’ll come across is type conversion and casting. These are fundamental concepts that allow you to work seamlessly with different data types, making your code more efficient, readable, and less prone to errors. Whether you’re a beginner or an experienced developer looking to polish your Python skills, this guide will take you through everything you need to know about type conversion and casting in Python—step by step and in plain English.
What Are Type Conversion and Casting?
In Python, every value has a specific data type, such as integers, strings, floats, or lists. Sometimes, you’ll need to convert one data type into another to perform operations or make your program more versatile. That’s where type conversion and casting come in.
- Type Conversion refers to converting one data type into another. Python provides built-in functions to handle this automatically or manually.
- Casting is a more explicit way of type conversion, where you take control of the process.
For example, you might need to convert a user’s input (which is usually a string) into an integer to perform arithmetic calculations.
Why Do We Need Type Conversion?
Let’s look at a common scenario:
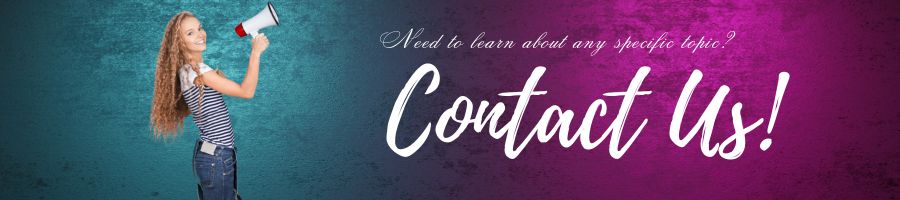
age = input("Enter your age: ") print(age + 5) # Raises a TypeError
Here, the input()
function returns a string, but you’re trying to add a number to it. Python throws a TypeError
because you can’t mix strings and integers directly in arithmetic operations.
To fix this, you’d need to convert the string age
into an integer using type conversion:
age = int(input("Enter your age: ")) print(age + 5) # Works perfectly
This simple adjustment makes the program work seamlessly. Now let’s dive deeper into how you can use type conversion and casting in your Python code.
Python’s Built-In Type Conversion Functions
Python offers several built-in functions for converting between data types. Here’s a quick overview:
Function | Description | Example |
---|---|---|
int() | Converts to an integer | int("42") -> 42 |
float() | Converts to a floating-point number | float("3.14") -> 3.14 |
str() | Converts to a string | str(42) -> "42" |
bool() | Converts to a boolean value | bool(1) -> True |
list() | Converts to a list | list("abc") -> ['a', 'b', 'c'] |
tuple() | Converts to a tuple | tuple([1, 2, 3]) -> (1, 2, 3) |
set() | Converts to a set | set([1, 2, 2]) -> {1, 2} |
dict() | Converts to a dictionary (from pairs) | dict([(1, 'a')]) -> {1: 'a'} |
Let’s break these down further with examples and use cases.
1. Converting Strings to Numbers
When you’re dealing with user inputs or file data, you’ll often need to convert strings to numbers. Here’s how:
Converting to an Integer:
number = int("123") # Converts string to integer print(number + 10) # Output: 133
Converting to a Float:
price = float("49.99") # Converts string to float print(price + 0.01) # Output: 50.0
Handling Invalid Conversions:
If you try to convert a non-numeric string, Python will raise a ValueError
. You can handle this gracefully with a try-except
block:
try: value = int("abc") except ValueError: print("Invalid input! Please enter a number.")
2. Numbers to Strings
Converting numbers to strings is useful when you want to concatenate them with text:
age = 30 message = "You are " + str(age) + " years old." print(message) # Output: You are 30 years old.
3. Boolean Conversions
Python treats certain values as False
and everything else as True
:
- False Values:
0
,0.0
,""
,[]
,{}
,None
- True Values: Everything else
print(bool(0)) # Output: False print(bool("hello")) # Output: True
4. Handling Edge Cases
Converting Very Large Numbers:
Python’s int
and float
types can handle very large numbers, but converting these between types can lead to precision loss:
large_num = 10**20 print(float(large_num)) # Output: 1e+20 (scientific notation)
Converting None
:
None
cannot be directly converted into int
or float
, but it converts to False
when cast to bool
:
print(bool(None)) # Output: False
5. Performance Considerations
Type conversions can be computationally expensive, especially in large datasets or loops. Avoid unnecessary conversions to optimize performance:
# Inefficient for i in range(1000000): num = int("123") # Efficient num = int("123") for i in range(1000000): pass
6. Common Pitfalls
Float Precision Errors:
Floats can introduce rounding errors due to their binary representation:
print(0.1 + 0.2) # Output: 0.30000000000000004
Use the decimal
module for high-precision calculations:
from decimal import Decimal print(Decimal("0.1") + Decimal("0.2")) # Output: 0.3
Implicit Conversions:
Python sometimes performs implicit conversions, which can lead to unexpected results:
print("5" * 2) # Output: 55 (string repetition) print(5 * 2.0) # Output: 10.0 (int to float conversion)
7. Real-Life Scenarios
Type Conversion in Data Analysis:
When working with CSV files, data often needs to be converted from strings to numbers for calculations:
import csv with open("data.csv") as file: reader = csv.reader(file) for row in reader: value = float(row[1]) # Convert string to float print(value * 2)
Type Conversion in APIs:
APIs often return JSON data as strings, which you’ll need to convert:
import json response = '{"age": "25"}' data = json.loads(response) age = int(data["age"]) print(age + 5) # Output: 30
Advanced Type Conversion: Custom Objects
Python allows you to define how your custom classes should behave during type conversion. To achieve this, you can override special methods like __int__
, __float__
, or __str__
in your class:
class Point: def __init__(self, x, y): self.x = x self.y = y def __str__(self): return f"Point({self.x}, {self.y})" def __int__(self): return self.x + self.y # Example Usage p = Point(3, 4) print(str(p)) # Output: Point(3, 4) print(int(p)) # Output: 7
Tips and Best Practices
- Use Explicit Casting: Avoid implicit conversions